ApplyFilter.cpp
79 built = (built && createPortIn<dmpvecarr3E*, ApplyFilter>(this, &ApplyFilter::setInput, M_GDISPLS, true, 1));
80 built = (built && createPortIn<dmpvector1D*, ApplyFilter>(this, &ApplyFilter::setScalarInput, M_SCALARFIELD, true, 1));
81 built = (built && createPortIn<dmpvector1D*, ApplyFilter>(this, &ApplyFilter::setFilter, M_FILTER));
82 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, Apply>(this, &BaseManipulation::setGeometry, M_GEOM));
84 built = (built && createPortOut<dmpvecarr3E*, ApplyFilter>(this, &ApplyFilter::getOutput, M_GDISPLS));
181 bitpit::SurfaceKernel* skernel = static_cast<bitpit::SurfaceKernel*>(m_scalarinput.getGeometry()->getPatch());
ApplyFilter is a class that applies a filter field to a deformation field defined on a geometry.
Definition: ApplyFilter.hpp:73
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ApplyFilter.cpp:141
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ApplyFilter.cpp:117
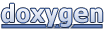