manipulators_example_00007.cpp
Example of usage of RBF manipulator with variable support radius to deform an input geometry.Using: MimmoGeometry, GenericInput, CreatePointCloud, ProjPatchOnSurface, MRBF, Apply, Chain.
To run : ./manipulators_example_00007
To run (MPI version): mpirun -np X manipulators_example_00007
visit: mimmo website
/*---------------------------------------------------------------------------*\
*
* mimmo
*
* Copyright (C) 2015-2021 OPTIMAD engineering Srl
*
* -------------------------------------------------------------------------
* License
* This file is part of mimmo.
*
* mimmo is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License v3 (LGPL)
* as published by the Free Software Foundation.
*
* mimmo is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with mimmo. If not, see <http://www.gnu.org/licenses/>.
*
\ *---------------------------------------------------------------------------*/
#include "mimmo_manipulators.hpp"
#include "mimmo_iogeneric.hpp"
#include "mimmo_utils.hpp"
#include <random>
// =================================================================================== //
void test00007() {
/*
Read a sphere from STL. Convert mode is to save the just read geometry in
another file with name manipulators_output_00007.0000.stl
*/
mimmo0->setReadFileType(FileType::STL);
mimmo0->setWriteFileType(FileType::STL);
/* write the deformed mesh to file */
mimmo1->setWriteDir(".");
mimmo1->setWriteFileType(FileType::STL);
mimmo1->setWriteFilename("manipulators_output_00007.0001");
/*
Creation of a random distribution of 10 points with coordinates between [-0.5, 0.5]
and a random distribution of 10 support radii between [0.05, 0.35]
*/
int np = 10;
dvecarr3E rbfNodes(10);
dvector1D supportRadii(10);
std::minstd_rand rgen, sgen;
rgen.seed(160);
sgen.seed(333);
double dist = (rgen.max()-rgen.min());
double dist2 = (sgen.max()-sgen.min());
for (int i=0; i<np; i++){
for (int j=0; j<3; j++){
rbfNodes[i][j] = 1.0*( double(rgen() - rgen.min() ) / dist ) - 0.5;
}
supportRadii[i] = 0.1 * ( double(sgen() - sgen.min() ) / dist2 ) + 0.05;
}
/*
Creation of a set of displacements of the control nodes of the radial basis functions.
* Use a radial displacements from a center point placed in axes origin.
*/
darray3E center({0.0, 0.0, 0.0});
for (int i=0; i<np; i++){
displ[i] = rbfNodes[i] - center;
displ[i] /= -20.0*norm2(displ[i]);
}
/*
Set Generic input block with the
nodes defined above.
*/
inputn->setInput(rbfNodes);
/* Set Generic input block with the
* supportRadii defined above.
*/
inputSR->setInput(supportRadii);
/* Set Generic input block with the
* displacements defined above.
*/
input->setInput(displ);
/*
Create a Point Cloud that will use the outputs of previous generic inputs.
Nodes, displacements and supportRadii will be stored as a point cloud mesh with a vector
field/scalar field attached, respectively.
*/
/*
This block will project the rbf point cloud onto the target surface
*/
/*
RBF manipulator, will use RBF point cloud, its vectorfield of displacements
and its scalarfield of variable supportRadii to build up itself.
It requires the definition of RBF function.
*/
/*
Create applier block.
It applies the deformation displacements to the original input geometry.
*/
/*
Setup pin connections.
*/
/*
Setup execution chain.
*/
mimmo::Chain ch0;
ch0.addObject(input);
ch0.addObject(inputn);
ch0.addObject(inputSR);
ch0.addObject(mimmo0);
ch0.addObject(pointcloud);
ch0.addObject(proj);
ch0.addObject(applier);
ch0.addObject(mrbf);
ch0.addObject(mimmo1);
/*
Execute the chain.
Use debug flag true to print out the execution steps.
*/
/*
Clean up & exit;
*/
delete mrbf;
delete proj;
delete pointcloud;
delete applier;
delete input;
delete inputSR;
delete mimmo0;
delete mimmo1;
return;
}
int main( int argc, char *argv[] ) {
BITPIT_UNUSED(argc);
BITPIT_UNUSED(argv);
#if MIMMO_ENABLE_MPI
MPI_Init(&argc, &argv);
#endif
try{
test00007() ;
}
catch(std::exception & e){
std::cout<<"manipulators_example_00007 exited with an error of type : "<<e.what()<<std::endl;
return 1;
}
#if MIMMO_ENABLE_MPI
MPI_Finalize();
#endif
return 0;
}
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setWorkingOnTarget(bool)
Definition: ProjPatchOnSurface.cpp:150
Apply is the class that applies the deformation resulting from a manipulation object to the geometry.
Definition: Apply.hpp:89
@ NONE
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setInput(T *data)
GENERICINPUT////////////////////////////////////////////////////////////////////////////.
Definition: GenericInput.tpp:209
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
@ DSIGMOID
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
Executable block class capable of projecting a surface patch, 3DCurve or PointCloud on a 3D surface,...
Definition: ProjPatchOnSurface.hpp:99
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
CreatePointCloud manages cloud point data in raw format to create a MimmoObject Point Cloud container...
Definition: CreatePointCloud.hpp:91
void setFunction(const MRBFBasisFunction &funct, bool isCompact=false)
Definition: MRBF.cpp:594
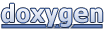