ProjPatchOnSurface.cpp
80 ProjPatchOnSurface::ProjPatchOnSurface(const ProjPatchOnSurface & other):ProjPrimitivesOnSurfaces(other){
160 void ProjPatchOnSurface::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
231 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ProjPatchOnSurface>(this, &mimmo::ProjPatchOnSurface::setPatch, M_GEOM2, true));
264 skdTreeUtils::projectPointGlobal(npoints, points.data(), getGeometry()->getSkdTree(), projs.data(), cell_ids.data(), ranks.data(), radius, false);
266 skdTreeUtils::projectPoint(npoints, points.data(), getGeometry()->getSkdTree(), projs.data(), cell_ids.data());
bool m_buildKdTree
Definition: ProjPrimitivesOnSurfaces.hpp:76
virtual void buildPorts()
Definition: ProjPrimitivesOnSurfaces.cpp:96
bool m_workingOnTarget
Definition: ProjPatchOnSurface.hpp:103
void setWorkingOnTarget(bool)
Definition: ProjPatchOnSurface.cpp:150
MimmoSharedPointer< MimmoObject > m_patch
Definition: ProjPrimitivesOnSurfaces.hpp:77
ProjPatchOnSurface()
Definition: ProjPatchOnSurface.cpp:43
bool m_buildSkdTree
Definition: ProjPrimitivesOnSurfaces.hpp:75
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ProjPatchOnSurface.cpp:205
void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ProjPatchOnSurface.cpp:160
void setPatch(MimmoSharedPointer< MimmoObject > geo)
Definition: ProjPatchOnSurface.cpp:122
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
Class for projecting 1D/2D primitives on a target 3D surface mesh.
Definition: ProjPrimitivesOnSurfaces.hpp:70
void swap(ProjPrimitivesOnSurfaces &x) noexcept
Definition: ProjPrimitivesOnSurfaces.cpp:82
Executable block class capable of projecting a surface patch, 3DCurve or PointCloud on a 3D surface,...
Definition: ProjPatchOnSurface.hpp:99
MimmoSharedPointer< MimmoObject > m_cobj
Definition: ProjPatchOnSurface.hpp:102
bool isWorkingOnTarget()
Definition: ProjPatchOnSurface.cpp:140
void setBuildSkdTree(bool build)
Definition: ProjPrimitivesOnSurfaces.cpp:154
darray3E projectPoint(const std::array< double, 3 > *point, const bitpit::PatchSkdTree *skdtree, double r)
Definition: SkdTreeUtils.cpp:375
virtual ~ProjPatchOnSurface()
Definition: ProjPatchOnSurface.cpp:74
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void setBuildKdTree(bool build)
Definition: ProjPrimitivesOnSurfaces.cpp:163
void swap(ProjPatchOnSurface &x) noexcept
Definition: ProjPatchOnSurface.cpp:98
ProjPatchOnSurface & operator=(ProjPatchOnSurface other)
Definition: ProjPatchOnSurface.cpp:89
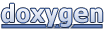