Executable block class capable of projecting a surface patch, 3DCurve or PointCloud on a 3D surface, both defined as MimmoObject. More...
#include <ProjPatchOnSurface.hpp>
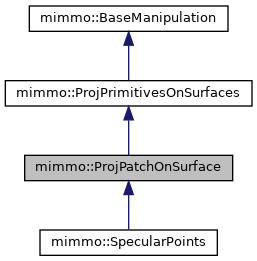
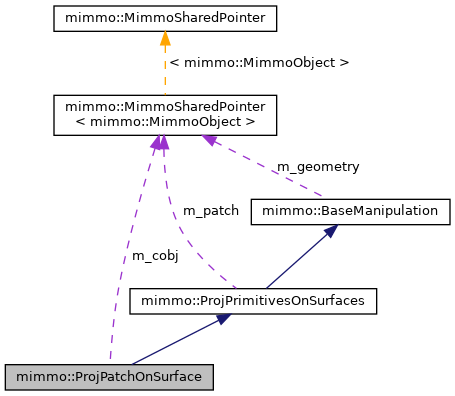
Protected Member Functions | |
void | projection () |
void | swap (ProjPatchOnSurface &x) noexcept |
![]() | |
void | swap (ProjPrimitivesOnSurfaces &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
MimmoSharedPointer< MimmoObject > | m_cobj |
bool | m_workingOnTarget |
![]() | |
bool | m_buildKdTree |
bool | m_buildSkdTree |
int | m_nC |
MimmoSharedPointer< MimmoObject > | m_patch |
int | m_topo |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Executable block class capable of projecting a surface patch, 3DCurve or PointCloud on a 3D surface, both defined as MimmoObject.
ProjPatchOnSurface project a 3D surface tessellations, 3DCurve tesselations or point clouds on a reference 3D surface mesh and return it in a MimmoObject container. Class does not support volume meshes projection.
Ports available in ProjPatchOnSurface Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM2 | setPatch | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
Inheriting ports from base class ProjPrimitivesOnSurfaces:
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getProjectedElement | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName : name of the class as
mimmo.ProjPatchOnSurface
; - Priority : uint marking priority in multi-chain execution;
- PlotInExecution : boolean 0/1 print optional results of the class;
- OutputPlot : target directory for optional results writing.
Proper of the class:
- SkdTree : evaluate skdTree true 1/false 0; this parameter is useless if the target surface to project is a PointCloud.
- KdTree : evaluate kdTree true 1/false 0;
- WorkingOnTarget : true 1/ 0 false to store projected target in the same container or and independent one;
Geometry has to be mandatorily passed through port.
- Examples
- manipulators_example_00006.cpp, manipulators_example_00007.cpp, and utils_example_00005.cpp.
Definition at line 99 of file ProjPatchOnSurface.hpp.
Constructor & Destructor Documentation
◆ ProjPatchOnSurface() [1/3]
mimmo::ProjPatchOnSurface::ProjPatchOnSurface | ( | ) |
Default constructor
Definition at line 43 of file ProjPatchOnSurface.cpp.
◆ ProjPatchOnSurface() [2/3]
mimmo::ProjPatchOnSurface::ProjPatchOnSurface | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 54 of file ProjPatchOnSurface.cpp.
◆ ~ProjPatchOnSurface()
|
virtual |
Default destructor
Definition at line 74 of file ProjPatchOnSurface.cpp.
◆ ProjPatchOnSurface() [3/3]
mimmo::ProjPatchOnSurface::ProjPatchOnSurface | ( | const ProjPatchOnSurface & | other | ) |
Copy constructor. No resulting projected patch is copied
Definition at line 80 of file ProjPatchOnSurface.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::SpecularPoints.
Definition at line 160 of file ProjPatchOnSurface.cpp.
◆ buildPorts()
|
virtual |
Building ports of the class
Reimplemented from mimmo::ProjPrimitivesOnSurfaces.
Reimplemented in mimmo::SpecularPoints.
Definition at line 227 of file ProjPatchOnSurface.cpp.
◆ clear()
void mimmo::ProjPatchOnSurface::clear | ( | ) |
Clear all elements on your current class
Definition at line 109 of file ProjPatchOnSurface.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::SpecularPoints.
Definition at line 205 of file ProjPatchOnSurface.cpp.
◆ isWorkingOnTarget()
bool mimmo::ProjPatchOnSurface::isWorkingOnTarget | ( | ) |
Return true, if the class will apply the modifications directly to the target surface container linked with setPatch. False, if projection is returned in an indipendent container.
- Returns
- true or false if is working on target.
Definition at line 140 of file ProjPatchOnSurface.cpp.
◆ operator=()
ProjPatchOnSurface & mimmo::ProjPatchOnSurface::operator= | ( | ProjPatchOnSurface | other | ) |
Assignement operator. No resulting projected patch is copied
Definition at line 89 of file ProjPatchOnSurface.cpp.
◆ projection()
|
protectedvirtual |
Core engine for projection.
Implements mimmo::ProjPrimitivesOnSurfaces.
Definition at line 240 of file ProjPatchOnSurface.cpp.
◆ setPatch()
void mimmo::ProjPatchOnSurface::setPatch | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Link the target Patch to be projected on the reference surface. Any patch type is allowed, except for volume meshes (MimmoObject of type 2). In that case nothing will be linked.
- Parameters
-
[in] geo pointer to patch that need to be projected
- Examples
- utils_example_00005.cpp.
Definition at line 122 of file ProjPatchOnSurface.cpp.
◆ setWorkingOnTarget()
void mimmo::ProjPatchOnSurface::setWorkingOnTarget | ( | bool | flag | ) |
Set to true, if the class will apply the modifications directly to the target surface container linked with setPatch. To False instead, if projection is returned in an indipendent container. param[in] flag true or false to set class working on target.
- Examples
- manipulators_example_00006.cpp, manipulators_example_00007.cpp, and utils_example_00005.cpp.
Definition at line 150 of file ProjPatchOnSurface.cpp.
◆ swap()
|
protectednoexcept |
Swap function
- Parameters
-
[in] x object to be swapped
Definition at line 98 of file ProjPatchOnSurface.cpp.
Member Data Documentation
◆ m_cobj
|
protected |
object to deal with patch
Definition at line 102 of file ProjPatchOnSurface.hpp.
◆ m_workingOnTarget
|
protected |
true, store projected results in the same target container
Definition at line 103 of file ProjPatchOnSurface.hpp.
The documentation for this class was generated from the following files:
- src/utils/ProjPatchOnSurface.hpp
- src/utils/ProjPatchOnSurface.cpp
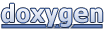