Radial Basis Function evaluation from clouds of control points. More...
#include <MRBF.hpp>
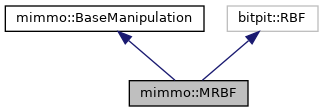
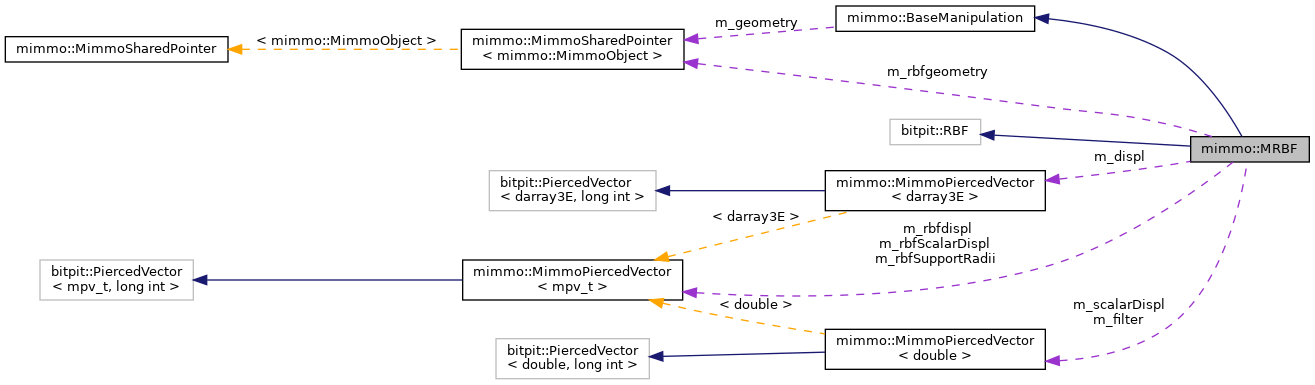
Protected Member Functions | |
void | checkFilter () |
void | computeEffectiveSupportRadiusList () |
std::vector< double > | evalRBF (const std::array< double, 3 > &val) |
bool | initRBFwGeometry () |
void | plotCloud (std::string directory, std::string filename, int counterFile, bool binary, bool deformed) |
virtual void | plotOptionalResults () |
void | setMode (MRBFSol solver) |
void | setWeight (dvector2D value) |
void | swap (MRBF &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
bool | m_areScalarResults |
bool | m_bfilter |
double | m_diagonalFactor |
dmpvecarr3E | m_displ |
dvector1D | m_effectiveSR |
dmpvector1D | m_filter |
int | m_functype |
bool | m_isCompact |
dmpvecarr3E * | m_rbfdispl |
MimmoSharedPointer< MimmoObject > | m_rbfgeometry |
dmpvector1D * | m_rbfScalarDispl |
dmpvector1D * | m_rbfSupportRadii |
dmpvector1D | m_scalarDispl |
MRBFSol | m_solver |
bool | m_srIsReal |
dvector1D | m_supportRadii |
double | m_supportRadiusValue |
double | m_tol |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Radial Basis Function evaluation from clouds of control points.
This class is derived from BaseManipulation class of mimmo and from RBF class of bitpit library. It evaluates the result of RBF functions built over a set of control point given externally or stored in a MimmoObject container. Displacements (DOFs) for RBF nodes are provided externally. They can be expressed as:
- a) 3-component vector
- b) 1-component scalar (as in the case of DOF representing displacements normal to surface). In that case the output geometry "displacement" field will be returned as a 3-component vector field (case a) ) or a scalar field (case b)).
The options are mutually exclusive (picking one exclude the other).
Default solver in execution is MRBFSol::NONE for direct parameterization. Use MRBFSol::GREEDY or MRBFSol::WHOLE to activate interpolation features. See bitpit::RBF docs for further information.
Support radii of RBF Nodes can be set in 3 different ways:
- setting x as Local support radius : the effective support radius will be calculated as x * bbox_diag, where the last is the diagonal of the RBF set of nodes bounding box. This value will be equal for all RBF nodes.(setSupportRadiusLocal)
- setting x as Real support radius: the value of x will be used as effective support radius, equal for all RBF nodes (setSupportRadiusReal)
- setting variable Support Radii: provide a list of support radius values, one for each RBF node in the set. This option is available only in MRBFSol::NONE mode setVariableSupportRadii)
In case all three methods are connected through ports, priority list will be: setVariableSupportRadii, setSupportRadiusReal, setSupportRadiusLocal
Ports available in MRBF Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_COORDS | setNode | (MC_VECARR3, MD_FLOAT) |
M_DISPLS | setDisplacements | (MC_VECARR3, MD_FLOAT) |
M_DATAFIELD | setScalarDisplacements | (MC_VECTOR, MD_FLOAT) |
M_DATAFIELD2 | setVariableSupportRadii | (MC_VECTOR, MD_FLOAT) |
M_FILTER | setFilter | (MC_SCALAR, MD_MPVECFLOAT_) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
M_GEOM2 | setNode | (MC_SCALAR, MD_MIMMO_) |
M_VECTORFIELD | setDisplacements | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_SCALARFIELD | setScalarDisplacements | (MC_SCALAR, MD_MPVECFLOAT_) |
M_SCALARFIELD2 | setVariableSupportRadii | (MC_SCALAR, MD_MPVECFLOAT_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GDISPLS | getDisplacements | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_SCALARFIELD | getScalarDisplacements | (MC_SCALAR, MD_MPVECFLOAT_) |
M_GEOM | getGeometry | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.MRBF
; - Priority: uint marking priority in multi-chain execution;
- Apply: boolean 0/1 activate apply deformation result on target geometry directly in execution;
- PlotInExecution : plot optional results in execution;
- OutputPlot : path to store optional results.
Proper of the class:
- Mode: 0/1/2 mode of usage of the class see MRBFSol enum;
- SupportRadiusLocal: local homogeneous radius of RBF function for each nodes, expressed as ratio of local geometry bounding box; see setSupportRadiusLocal method documentation.
- SupportRadiusReal: homogeneous real radius of RBF function common to each RBF node; see setSupportRadiusReal method documentation.
- RBFShape: shape of RBF function see MRBFBasisFunction and bitpit::RBFBasisFunction enums;
- Tolerance: greedy engine tolerance (meaningful for Mode 2 only);
DiagonalFactor: factor used to define a threshold to filter geometry vertices (default 1.0);
if set, SupportRadiusReal parameter bypass SupportRadiusLocal one.
Geometry, filter field, RBF nodes and displacements have to be mandatorily passed through port. Please not if class is in MRBFSol::NONE mode and a port to setVariableSupportRadii is linked, SupportRadiusLocal/Real parameters are bypassed.
Constructor & Destructor Documentation
◆ MRBF() [1/3]
mimmo::MRBF::MRBF | ( | MRBFSol | mode = MRBFSol::NONE | ) |
◆ MRBF() [2/3]
mimmo::MRBF::MRBF | ( | const bitpit::Config::Section & | rootXML | ) |
◆ ~MRBF()
◆ MRBF() [3/3]
mimmo::MRBF::MRBF | ( | const MRBF & | other | ) |
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
◆ addNode() [1/2]
int mimmo::MRBF::addNode | ( | darray3E | node | ) |
Adds a RBF point to the total control node list and activate it. Reimplemented from RBF::addNode of bitpit;
- Parameters
-
[in] node coordinates of control point.
- Returns
- RBF id.
- Examples
- genericinput_example_00005.cpp.
◆ addNode() [2/2]
std::vector< int > mimmo::MRBF::addNode | ( | dvecarr3E | nodes | ) |
◆ apply()
|
virtual |
Directly apply deformation field to target geometry.
Reimplemented from mimmo::BaseManipulation.
◆ areResultsInScalarMode()
bool mimmo::MRBF::areResultsInScalarMode | ( | ) |
Return true if the the class is in Scalar mode, false otherwise. Scalar mode means that a set of Scalar DOFs displacements for RBFs nodes are feeded in input so that the final expected displacement field on the geometry is scalar. Otherwise, dofs are 3component vectors that produce a final 3-comp vector displacement field.
- Returns
- boolean true/false
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
◆ checkDuplicatedNodes()
ivector1D mimmo::MRBF::checkDuplicatedNodes | ( | double | tol = 1.0E-12 | ) |
◆ checkFilter()
|
protected |
◆ clear()
void mimmo::MRBF::clear | ( | ) |
◆ clearFilter()
◆ computeEffectiveSupportRadiusList()
|
protected |
◆ evalRBF()
|
protected |
Evaluates the displacements value with RBF . Supported in all modes. Use weights, RBF node positions and m_effectiveSR (support radius structure) of each RBF node to retrive the deformation field.
- Parameters
-
[in] point point where to evaluate the basis
- Returns
- array containing interpolated/parameterized values of displacements.
◆ execute()
|
virtual |
Execution of RBF object. It evaluates the displacements (values) over the point of the linked geometry, given as result of RBF technique implemented in bitpit::RBF base class. The result is stored in the m_displ member.
Implements mimmo::BaseManipulation.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
◆ getDiagonalFactor()
double mimmo::MRBF::getDiagonalFactor | ( | ) |
◆ getDisplacements()
dmpvecarr3E * mimmo::MRBF::getDisplacements | ( | ) |
Return actual computed vector displacements field (if any) for the geometry linked. BEWARE if class is working with scalar DOFs a scalar displacement field is expected. For vector DOFs a vector field is expected. If the class is in "scalar mode" (areResultsInScalarMode() is true) this method will return nullptr.Use getScalarDisplacements instead.
- Returns
- The computed deformation field on the vertices of the linked geometry
◆ getEffectivelyUsedSupportRadii()
dvector1D & mimmo::MRBF::getEffectivelyUsedSupportRadii | ( | ) |
◆ getFilter()
dmpvector1D * mimmo::MRBF::getFilter | ( | ) |
It gets current set filter field. See MRBF::setFilter
- Returns
- filter field.
◆ getFunctionType()
int mimmo::MRBF::getFunctionType | ( | ) |
◆ getMode()
MRBFSol mimmo::MRBF::getMode | ( | ) |
Return actual solver set for RBF data fields interpolation in MRBF::execute Reimplemented from RBF::getMode() of bitpit;
- Returns
- solver mode
◆ getNodes()
dvecarr3E * mimmo::MRBF::getNodes | ( | ) |
◆ getScalarDisplacements()
dmpvector1D * mimmo::MRBF::getScalarDisplacements | ( | ) |
Return actual computed scalar displacements field (if any) for the geometry linked. BEWARE if class is working with scalar DOFs a scalar displacement field is expected. For vector DOFs a vector field is expected. If the class is NOT in "scalar mode" (areResultsInScalarMode() is false) this method will return nullptr. Use getDisplacements instead.
- Returns
- The computed deformation field on the vertices of the linked geometry
◆ initRBFwGeometry()
|
protected |
Initialize RBF nodes and displacements with the related MimmoObject geometry.
- Returns
- Valid intialization flag; false if displacements fields and geometry are not coherent
◆ isCompact()
bool mimmo::MRBF::isCompact | ( | ) |
◆ isSupportRadiusReal()
bool mimmo::MRBF::isSupportRadiusReal | ( | ) |
◆ isVariableSupportRadiusSet()
bool mimmo::MRBF::isVariableSupportRadiusSet | ( | ) |
◆ operator=()
◆ plotCloud()
|
protected |
Plot your current rbf nodes as a point cloud to *vtu file.
- Parameters
-
[in] directory output directory [in] filename output filename w/out tag [in] counterFile integer identifier of the file [in] binary boolean flag for 0-"ascii" or 1-"appended" writing [in] deformed boolean flag for plotting 0-"original points", 1-"moved points"
◆ plotOptionalResults()
|
protectedvirtual |
Plot Optional results of the class. It plots the RBF control nodes as a point cloud in *.vtu format, for both original/moved control nodes.
Reimplemented from mimmo::BaseManipulation.
◆ removeDuplicatedNodes()
bool mimmo::MRBF::removeDuplicatedNodes | ( | ivector1D * | list = nullptr | ) |
Erase all nodes passed by their RBF id list. If no list is provided, the method find all possible duplicated nodes within a default tolerance of 1.0E-12 and erase them, if any.
- Parameters
-
[in] list pointer to a list of id's of RBF candidate nodes
- Returns
- boolean, true if all duplicated nodes are erased, false if one or more of them are not.
◆ setCompactSupport()
void mimmo::MRBF::setCompactSupport | ( | bool | isCompact = true | ) |
◆ setDiagonalFactor()
void mimmo::MRBF::setDiagonalFactor | ( | double | diagonalFactor | ) |
◆ setDisplacements() [1/2]
void mimmo::MRBF::setDisplacements | ( | dmpvecarr3E * | displ | ) |
Set a field of 3D displacements on your RBF Nodes. According to MRBFSol mode active in the class set: displacements as direct RBF weights coefficients in MRBFSol::NONE mode, or interpolate displacements to get the best fit weights in other modes MRBFSol::GREEDY/WHOLE Displacements size may not match the actual number of RBF nodes stored in the class. To ensure consistency call fitDataToNodes() method inherited from RBF class. BEWARE: calling this method implicitly set the class to work with 3Comp vector DOFs and to retrieve a 3 comp vector geometry displacement field.(Scalar mode off).
- Parameters
-
[in] displ pointer to mimmo pierced vector of nodal displacements
◆ setDisplacements() [2/2]
void mimmo::MRBF::setDisplacements | ( | dvecarr3E | displ | ) |
Set a field of 3D displacements on your RBF Nodes. According to MRBFSol mode active in the class set: displacements as direct RBF weights coefficients in MRBFSol::NONE mode, or interpolate displacements to get the best fit weights in other modes MRBFSol::GREEDY/WHOLE Displacements size may not match the actual number of RBF nodes stored in the class. To ensure consistency call fitDataToNodes() method inherited from RBF class. BEWARE: calling this method implicitly set the class to work with 3Comp vector DOFs and to retrieve a 3 comp vector geometry displacement field.(Scalar mode off).
- Parameters
-
[in] displ list of nodal displacements
- Examples
- genericinput_example_00005.cpp, and geohandlers_example_00003.cpp.
◆ setFilter()
void mimmo::MRBF::setFilter | ( | dmpvector1D * | filter | ) |
◆ setFunction() [1/2]
void mimmo::MRBF::setFunction | ( | const bitpit::RBFBasisFunction & | bfunc, |
bool | isCompact = false |
||
) |
◆ setFunction() [2/2]
void mimmo::MRBF::setFunction | ( | const MRBFBasisFunction & | bfunc, |
bool | isCompact = false |
||
) |
Sets the rbf function to be used. Supported in both modes. (Overloading for mimmo rbf functions)
- Parameters
-
[in] bfunc basis function to be used [in] isCompact is the basis function to be used on a compact support? (default = false)
◆ setMode()
|
protected |
Set type of solver set for RBF data fields interpolation/parameterization in MRBF::execute. Reimplemented from RBF::setMode() of bitpit;
- Parameters
-
[in] solver type of MRBFSol enum;
◆ setNode() [1/3]
void mimmo::MRBF::setNode | ( | darray3E | node | ) |
Set a RBF point as unique control node and activate it.
- Parameters
-
[in] node coordinates of control point.
- Examples
- geohandlers_example_00003.cpp.
◆ setNode() [2/3]
void mimmo::MRBF::setNode | ( | dvecarr3E | nodes | ) |
◆ setNode() [3/3]
void mimmo::MRBF::setNode | ( | MimmoSharedPointer< MimmoObject > | geometry | ) |
Set the RBF points as control nodes extracting the vertices stored in a MimmoObject container.
- Parameters
-
[in] geometry Pointer to MimmoObject that contains the geometry.
◆ setScalarDisplacements() [1/2]
void mimmo::MRBF::setScalarDisplacements | ( | dmpvector1D * | displ | ) |
Set a field of "Scalar" displacements on your RBF Nodes. According to MRBFSol mode active in the class set: displacements as direct RBF weights coefficients in MRBFSol::NONE mode, or interpolate displacements to get the best fit weights in other modes MRBFSol::GREEDY/WHOLE Displacements size may not match the actual number of RBF nodes stored in the class. To ensure consistency call fitDataToNodes() method inherited from RBF class. BEWARE: calling this method implicitly set the class to work with scalar DOFs and to retrieve a scalar geometry displacement field.(Scalar mode on).
- Parameters
-
[in] displ pointer to mimmo pierced vector of nodal displacements
◆ setScalarDisplacements() [2/2]
void mimmo::MRBF::setScalarDisplacements | ( | dvector1D | displ | ) |
Set a field of "Scalar" displacements (1 component) on your RBF Nodes. According to MRBFSol mode active in the class set: displacements as direct RBF weights coefficients in MRBFSol::NONE mode, or interpolate displacements to get the best fit weights in other modes MRBFSol::GREEDY/WHOLE Displacements size may not match the actual number of RBF nodes stored in the class. To ensure consistency call fitDataToNodes() method inherited from RBF class. BEWARE: calling this method implicitly set the class to work with scalar DOFs and to retrieve a scalar geometry displacement field.(Scalar mode on).
- Parameters
-
[in] displ list of nodal displacements
◆ setSupportRadiusLocal()
void mimmo::MRBF::setSupportRadiusLocal | ( | double | suppR_ | ) |
Set ratio a of support radius R of RBF kernel functions (HOMOGENEOUS FOR ALL OF THEM), according to the formula R = a*D, where D is the diagonal of the Axis Aligned Bounding Box referred to the targetgeometry. During the execution the correct value of R is applied. The ratio a can have value between ]0,+inf), which corresponding to minimum locally narrowed function, and almost flat functions (as sphere of infinite radius), respectively. Negative or zero values, bind the evaluation of R to the maximum displacement applied to RBF node, that is R is set proportional to the maximum displacement value.
- Parameters
-
[in] suppR_ new value of suppR
- Examples
- geohandlers_example_00003.cpp.
◆ setSupportRadiusReal()
void mimmo::MRBF::setSupportRadiusReal | ( | double | suppR_ | ) |
Set the real physical value of the support radius R of RBF kernel functions (HOMOGENEOUS FOR ALL OF THEM). During the execution the correct value of R is applied. The support radius a can have value between ]0,+inf), which corresponds to minimum locally narrowed function and almost flat functions (as sphere of infinite radius), respectively. Negative or zero values, bind the evaluation of R to the maximum displacement applied to RBF node, that is R is set proportional to the maximum displacement value.
- Parameters
-
[in] suppR_ new value of support radius.
◆ setSupportRadiusValue()
void mimmo::MRBF::setSupportRadiusValue | ( | double | suppR_ | ) |
◆ setTol()
void mimmo::MRBF::setTol | ( | double | tol | ) |
It sets the tolerance for GREEDY mode - interpolation algorithm. Tolerance infos are not used in MRBFSol::NONE/WHOLE mode.
- Parameters
-
[in] tol Target tolerance.
◆ setVariableSupportRadii() [1/2]
void mimmo::MRBF::setVariableSupportRadii | ( | dmpvector1D * | sradii | ) |
Set a list of real physical values of the support radius R, one for each RBF kernel functions. See MRBF::setVariableSupportRadii(dvector1D sradii) for details. This method is an overload with a different input container.
- Parameters
-
[in] sradii pointer to a MimmoPiercedVector with variable support radii Note. The MRBFSol::NONE method has to be set before to call this method.
◆ setVariableSupportRadii() [2/2]
void mimmo::MRBF::setVariableSupportRadii | ( | dvector1D | sradii | ) |
Set a list of real physical values of the support radius R, one for each RBF kernel functions. Method is available only in MRBFSol::NONE mode of the class. Support radii a can have value between ]0,+inf), which corresponds to minimum locally narrowed function and almost flat functions (as sphere of infinite radius), respectively. Negative values are set to minimum allowed support radius value. List size may not fit the number of RBF nodes: in that case automatic resize will be performed during execution.
- Parameters
-
[in] sradii non empty list of variable support radii (otherwise method does nothing) Note. The MRBFSol::NONE method has to be set before to call this method.
◆ setWeight()
|
protected |
Set a field of n-Dim weights on your RBF Nodes. Supported only in MRBFSol::NONE mode. Weights total number may not match the actual number of RBF nodes stored in the class. To ensure consistency call fitDataToNodes() method inherited from RBF class.
- Parameters
-
[in] value list of nodal weights
◆ swap()
|
protectednoexcept |
Member Data Documentation
◆ m_areScalarResults
|
protected |
◆ m_bfilter
|
protected |
◆ m_diagonalFactor
|
protected |
Factor used to define a threshold to filter geometry vertices to be deformed or not. The condition to use the whole geometry instead of filtering by a kdtree binary search is : maximum value of -support radii values- GREATER THAN m_diagonalFactor times diagonal of geometry AABB
◆ m_displ
|
protected |
◆ m_effectiveSR
|
protected |
◆ m_filter
|
protected |
◆ m_functype
|
protected |
◆ m_isCompact
|
protected |
◆ m_rbfdispl
|
protected |
RBF nodes displacements as vectors, when a RBF point cloud MimmoObject is linked.
◆ m_rbfgeometry
|
protected |
◆ m_rbfScalarDispl
|
protected |
RBF nodes displacements as scalars, when a RBF point cloud MimmoObject is linked.
◆ m_rbfSupportRadii
|
protected |
◆ m_scalarDispl
|
protected |
◆ m_solver
|
protected |
◆ m_srIsReal
|
protected |
◆ m_supportRadii
|
protected |
◆ m_supportRadiusValue
|
protected |
◆ m_tol
|
protected |
The documentation for this class was generated from the following files:
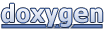