GenericInput is the class that set the initialization of a generic input data. More...
#include <GenericInput.hpp>
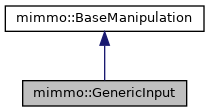
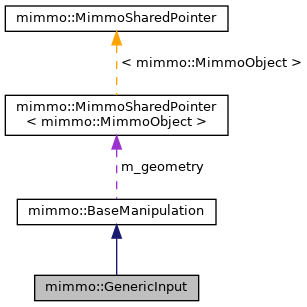
Public Member Functions | |
GenericInput (bool readFromFile=false, bool csv=false) | |
GenericInput (const bitpit::Config::Section &rootXML) | |
GenericInput (const GenericInput &other) | |
GenericInput (std::string dir, std::string filename, bool csv=false) | |
template<typename T > | |
GenericInput (T data) | |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | clearInput () |
void | clearResult () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
template<typename T > | |
T * | getInput () |
template<typename T > | |
T | getResult () |
GenericInput & | operator= (GenericInput other) |
void | setCSV (bool csv) |
void | setFilename (std::string filename) |
template<typename T > | |
void | setInput (T &data) |
template<typename T > | |
void | setInput (T *data) |
GENERICINPUT////////////////////////////////////////////////////////////////////////////. More... | |
void | setReadDir (std::string dir) |
void | setReadFromFile (bool readFromFile) |
template<typename T > | |
void | setResult (T &data) |
template<typename T > | |
void | setResult (T *data) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | swap (GenericInput &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
GenericInput is the class that set the initialization of a generic input data.
GenericInput is derived from BaseManipulation class. GenericInput can read the input from a data file (unformatted/csv) or it can be set by using setInput methods. When reading, the GenericInput object recognizes the type of data and it adapts the reading method in function of the output port used to build link (pin) with other objects. On MPI versions, GenericInput read data from file with proc rank 0, and send all the data to all other proc.
Ports available in GenericInput Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_COORDS | getResult | (MC_VECARR3, MD_FLOAT) |
M_DISPLS | getResult | (MC_VECARR3, MD_FLOAT) |
M_DATAFIELD | getResult | (MC_VECTOR, MD_FLOAT) |
M_POINT | getResult | (MC_ARRAY3, MD_FLOAT) |
M_SPAN | getResult | (MC_ARRAY3, MD_FLOAT) |
M_DIMENSION | getResult | (MC_ARRAY3, MD_INT) |
M_VALUED | getResult | (MC_SCALAR, MD_FLOAT) |
M_VALUEI | getResult | (MC_SCALAR, MD_INT) |
M_VALUEB | getResult | (MC_SCALAR, MD_BOOL) |
M_DEG | getResult | (MC_ARRAY3, MD_INT) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.GenericInput
; - Priority: uint marking priority in multi-chain execution;
Proper of the class:
- ReadFromFile: 0/1 set class to read from a file;
- CSV: 0/1 set class to read a CSV format;
- ReadDir: path to your current file data;
- Filename: path to your current file data.
- Examples
- genericinput_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00005.cpp, manipulators_example_00006.cpp, manipulators_example_00007.cpp, and manipulators_example_00008.cpp.
Definition at line 110 of file GenericInput.hpp.
Constructor & Destructor Documentation
◆ GenericInput() [1/5]
mimmo::GenericInput::GenericInput | ( | bool | readFromFile = false , |
bool | csv = false |
||
) |
Default constructor of GenericInput.
- Parameters
-
[in] readFromFile True if the object reads the values from file (default value false). [in] csv True if the input file is a csv format file (default value false).
Definition at line 36 of file GenericInput.cpp.
◆ GenericInput() [2/5]
mimmo::GenericInput::GenericInput | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 48 of file GenericInput.cpp.
◆ GenericInput() [3/5]
mimmo::GenericInput::GenericInput | ( | std::string | dir, |
std::string | filename, | ||
bool | csv = false |
||
) |
Custom constructor of GenericInput.
- Parameters
-
[in] dir Directory of the input file. [in] filename Name of the input file. [in] csv True if the input file is a csv format file (default value false). The m_readFromFile flag is set to true.
Definition at line 74 of file GenericInput.cpp.
◆ GenericInput() [4/5]
|
inline |
Custom template constructor of GenericInput. It sets the base class input with data passed as argument.
- Parameters
-
[in] data Data used to set the input.
Definition at line 133 of file GenericInput.hpp.
◆ GenericInput() [5/5]
mimmo::GenericInput::GenericInput | ( | const GenericInput & | other | ) |
Copy constructor of GenericInput. m_input and m_result members are not copied.
Definition at line 88 of file GenericInput.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 199 of file GenericInput.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 154 of file GenericInput.cpp.
◆ clearInput()
void mimmo::GenericInput::clearInput | ( | ) |
It clear the input member of the object
Definition at line 175 of file GenericInput.cpp.
◆ clearResult()
void mimmo::GenericInput::clearResult | ( | ) |
It clear the result member of the object
Definition at line 182 of file GenericInput.cpp.
◆ execute()
|
virtual |
Execution command. It does nothing, the real execution of the object happens in setInput/getResult.
Implements mimmo::BaseManipulation.
Definition at line 191 of file GenericInput.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 249 of file GenericInput.cpp.
◆ getInput()
T * mimmo::GenericInput::getInput |
It gets the input member of the object.
- Returns
- Pointer to data stored in the input member.
Definition at line 232 of file GenericInput.tpp.
◆ getResult()
T mimmo::GenericInput::getResult |
Overloaded function of base class getResult. It gets the result of the object, equal to the input. In the case it reads the input from file before to set and to get the result.
- Returns
- Pointer to data stored in result member.
Definition at line 323 of file GenericInput.tpp.
◆ operator=()
GenericInput & mimmo::GenericInput::operator= | ( | GenericInput | other | ) |
Assignment operator. m_input and m_result members are not copied.
Definition at line 98 of file GenericInput.cpp.
◆ setCSV()
void mimmo::GenericInput::setCSV | ( | bool | csv | ) |
It sets if the input file is in csv format.
- Parameters
-
[in] csv Is the input file write in comma separated value format?
- Examples
- genericinput_example_00001.cpp.
Definition at line 147 of file GenericInput.cpp.
◆ setFilename()
void mimmo::GenericInput::setFilename | ( | std::string | filename | ) |
It sets the name of the input file.
- Parameters
-
[in] filename Name of the input file.
- Examples
- genericinput_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00005.cpp, and manipulators_example_00008.cpp.
Definition at line 131 of file GenericInput.cpp.
◆ setInput() [1/2]
void mimmo::GenericInput::setInput | ( | T & | data | ) |
Overloaded function of base class setInput. It sets the input of the object, but at the same time it sets even the result.
- Parameters
-
[in] data Data to be used to set the input/result.
Definition at line 221 of file GenericInput.tpp.
◆ setInput() [2/2]
void mimmo::GenericInput::setInput | ( | T * | data | ) |
GENERICINPUT////////////////////////////////////////////////////////////////////////////.
Overloaded function of base class setInput. It sets the input of the object, but at the same time it sets even the result.
- Parameters
-
[in] data Pointer to data to be used to set the input/result.
- Examples
- manipulators_example_00003.cpp, manipulators_example_00004.cpp, manipulators_example_00006.cpp, and manipulators_example_00007.cpp.
Definition at line 209 of file GenericInput.tpp.
◆ setReadDir()
void mimmo::GenericInput::setReadDir | ( | std::string | dir | ) |
It sets the name of the directory of input file.
- Parameters
-
[in] dir Name of the directory of input file.
- Examples
- genericinput_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00005.cpp, and manipulators_example_00008.cpp.
Definition at line 139 of file GenericInput.cpp.
◆ setReadFromFile()
void mimmo::GenericInput::setReadFromFile | ( | bool | readFromFile | ) |
It sets if the object imports the displacements from an input file.
- Parameters
-
[in] readFromFile True if the object reads the values from file.
- Examples
- genericinput_example_00001.cpp, manipulators_example_00002.cpp, manipulators_example_00003.cpp, and manipulators_example_00005.cpp.
Definition at line 123 of file GenericInput.cpp.
◆ setResult() [1/2]
void mimmo::GenericInput::setResult | ( | T & | data | ) |
It sets the result member of the object.
- Parameters
-
[in] data Data to be stored in the result member.
Definition at line 251 of file GenericInput.tpp.
◆ setResult() [2/2]
void mimmo::GenericInput::setResult | ( | T * | data | ) |
It sets the result member of the object.
- Parameters
-
[in] data Pointer to data to be stored in the result member.
Definition at line 242 of file GenericInput.tpp.
◆ swap()
|
protectednoexcept |
The documentation for this class was generated from the following files:
- src/iogeneric/GenericInput.hpp
- src/iogeneric/GenericInput.cpp
- src/iogeneric/GenericInput.tpp
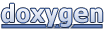