GenericInput.cpp
158 built = (built && createPortOut<dvecarr3E, GenericInput>(this, &mimmo::GenericInput::getResult<dvecarr3E>, M_COORDS));
159 built = (built && createPortOut<dvecarr3E, GenericInput>(this, &mimmo::GenericInput::getResult<dvecarr3E>, M_DISPLS));
160 built = (built && createPortOut<dvector1D, GenericInput>(this, &mimmo::GenericInput::getResult<dvector1D>, M_DATAFIELD));
161 built = (built && createPortOut<darray3E, GenericInput>(this, &mimmo::GenericInput::getResult<darray3E>, M_POINT));
162 built = (built && createPortOut<darray3E, GenericInput>(this, &mimmo::GenericInput::getResult<darray3E>, M_SPAN));
163 built = (built && createPortOut<iarray3E, GenericInput>(this, &mimmo::GenericInput::getResult<iarray3E>, M_DIMENSION));;
164 built = (built && createPortOut<double, GenericInput>(this, &mimmo::GenericInput::getResult<double>, M_VALUED));
165 built = (built && createPortOut<int, GenericInput>(this, &mimmo::GenericInput::getResult<int>, M_VALUEI));
166 built = (built && createPortOut<bool, GenericInput>(this, &mimmo::GenericInput::getResult<bool>, M_VALUEB));
167 built = (built && createPortOut<iarray3E, GenericInput>(this, &mimmo::GenericInput::getResult<iarray3E>, M_DEG));
318 GenericInputMPVData::GenericInputMPVData(const GenericInputMPVData & other):BaseManipulation(other){
387 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, GenericInputMPVData>(this, &mimmo::GenericInputMPVData::setGeometry, M_GEOM));
388 built = (built && createPortOut<dmpvector1D*, GenericInputMPVData>(this, &mimmo::GenericInputMPVData::getResult<double>, M_SCALARFIELD));
389 built = (built && createPortOut<dmpvecarr3E*, GenericInputMPVData>(this, &mimmo::GenericInputMPVData::getResult<darray3E>, M_VECTORFIELD));
415 GenericInputMPVData::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
GenericInputMPVData(bool csv=false)
Definition: GenericInput.cpp:266
GenericInputMPVData is the class that set a generic input data as mimmo::MimmoPiercedVector.
Definition: GenericInput.hpp:246
GenericInput & operator=(GenericInput other)
Definition: GenericInput.cpp:98
GenericInputMPVData & operator=(GenericInputMPVData other)
Definition: GenericInput.cpp:328
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setReadDir(std::string dir)
Definition: GenericInput.cpp:376
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericInput.cpp:199
GenericInput is the class that set the initialization of a generic input data.
Definition: GenericInput.hpp:110
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void setFilename(std::string filename)
Definition: GenericInput.cpp:368
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
GenericInput(bool readFromFile=false, bool csv=false)
Definition: GenericInput.cpp:36
void setReadFromFile(bool readFromFile)
Definition: GenericInput.cpp:123
void setFilename(std::string filename)
Definition: GenericInput.cpp:131
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericInput.cpp:465
void swap(GenericInputMPVData &x) noexcept
Definition: GenericInput.cpp:337
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericInput.cpp:415
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericInput.cpp:249
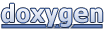