CreatePointCloud manages cloud point data in raw format to create a MimmoObject Point Cloud container. More...
#include <CreatePointCloud.hpp>
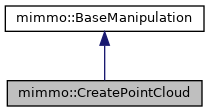

Protected Member Functions | |
void | buildPorts () |
void | plotOptionalResults () |
void | swap (CreatePointCloud &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
dmpvecarr3E | m_rawpoints |
dmpvector1D | m_rawscalar |
dmpvecarr3E | m_rawvector |
dmpvector1D | m_scalarfield |
dmpvecarr3E | m_vectorfield |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
CreatePointCloud manages cloud point data in raw format to create a MimmoObject Point Cloud container.
The class takes point data from a raw list and reverse them in a MimmoObject Point Cloud container. List can be set as pure vector or as a MimmoPiercedVector. Please be aware one set method exclude the other. Optionally, If any scalar/vector field are assigned to raw points, they will be trasformed in data containers associated to the point cloud. Please note if the raw points are passed with a specific container list (vector or MimmoPiercedVector), only data passed with the same kind of container will be taken into account.
MPI version retains data only on the master rank (rank 0). For now, MPI Setting of raw data is supposed to happen in this two configuration: 1) only master rank (0) retains the data 2) every rank has the same identical data sets. So, each time the class will consider only data from master rank. No Point Cloud distribution among ranks is perfomed. Mesh and Field Data, even if are parallel, are filled only for the partition of master rank(0). The other ranks have empty partition for Mesh and Field Data.
Ports available in CreatePointCloud Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_COORDS | setRawPoints | (MC_VECARR3, MD_FLOAT) |
M_DISPLS | setRawVectorField | (MC_VECARR3, MD_FLOAT) |
M_DATAFIELD | setRawScalarField | (MC_VECTOR, MD_FLOAT) |
M_VECTORFIELD | setRawPoints | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_VECTORFIELD2 | setRawVectorField | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_SCALARFIELD | setRawScalarField | (MC_SCALAR,MD_MPVECFLOAT_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR,MD_MIMMO_) |
M_SCALARFIELD | getScalarField | (MC_SCALAR,MD_MPVECFLOAT_) |
M_VECTORFIELD | getVectorField | (MC_SCALAR,MD_MPVECARR3FLOAT_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.CreatePointCloud
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing.
- Examples
- manipulators_example_00006.cpp, and manipulators_example_00007.cpp.
Definition at line 91 of file CreatePointCloud.hpp.
Constructor & Destructor Documentation
◆ CreatePointCloud() [1/3]
mimmo::CreatePointCloud::CreatePointCloud | ( | ) |
Default constructor of CreatePointCloud.
Definition at line 32 of file CreatePointCloud.cpp.
◆ CreatePointCloud() [2/3]
mimmo::CreatePointCloud::CreatePointCloud | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 40 of file CreatePointCloud.cpp.
◆ CreatePointCloud() [3/3]
mimmo::CreatePointCloud::CreatePointCloud | ( | const CreatePointCloud & | other | ) |
Copy constructor of CreatePointCloud. Only sensible inputs are copied.
Definition at line 61 of file CreatePointCloud.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 287 of file CreatePointCloud.cpp.
◆ buildPorts()
|
protectedvirtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 93 of file CreatePointCloud.cpp.
◆ clear()
void mimmo::CreatePointCloud::clear | ( | ) |
Clear all data stored in the class
Definition at line 206 of file CreatePointCloud.cpp.
◆ execute()
|
virtual |
Execution command. Read data from or Write data on linked filename
Implements mimmo::BaseManipulation.
Definition at line 220 of file CreatePointCloud.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 300 of file CreatePointCloud.cpp.
◆ getScalarField()
dmpvector1D * mimmo::CreatePointCloud::getScalarField | ( | ) |
Return the scalar field stored in the class as pointer to MimmoPiercedVector object.
- Returns
- scalar field stored in the class
Definition at line 116 of file CreatePointCloud.cpp.
◆ getVectorField()
dmpvecarr3E * mimmo::CreatePointCloud::getVectorField | ( | ) |
Return the vector field stored in the class as pointer to MimmoPiercedVector object.
- Returns
- vector field stored in the class
Definition at line 125 of file CreatePointCloud.cpp.
◆ operator=()
CreatePointCloud & mimmo::CreatePointCloud::operator= | ( | CreatePointCloud | other | ) |
Assignement operator of CreatePointCloud.
Definition at line 69 of file CreatePointCloud.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
Plot cloud point and store it in *.vtu file
Reimplemented from mimmo::BaseManipulation.
Definition at line 313 of file CreatePointCloud.cpp.
◆ setRawPoints() [1/2]
void mimmo::CreatePointCloud::setRawPoints | ( | dmpvecarr3E * | rawPoints | ) |
It sets the point cloud raw list
- Parameters
-
[in] rawPoints raw list of points.
Definition at line 135 of file CreatePointCloud.cpp.
◆ setRawPoints() [2/2]
void mimmo::CreatePointCloud::setRawPoints | ( | dvecarr3E | rawPoints | ) |
It sets the point cloud raw list
- Parameters
-
[in] rawPoints raw list of points.
Definition at line 144 of file CreatePointCloud.cpp.
◆ setRawScalarField() [1/2]
void mimmo::CreatePointCloud::setRawScalarField | ( | dmpvector1D * | rawScalarField | ) |
It sets the scalar field optionally attached to point cloud raw list
- Parameters
-
[in] rawScalarField raw scalar field.
Definition at line 183 of file CreatePointCloud.cpp.
◆ setRawScalarField() [2/2]
void mimmo::CreatePointCloud::setRawScalarField | ( | dvector1D | rawScalarField | ) |
It sets the scalar field optionally attached to point cloud raw list
- Parameters
-
[in] rawScalarField raw scalar field.
Definition at line 192 of file CreatePointCloud.cpp.
◆ setRawVectorField() [1/2]
void mimmo::CreatePointCloud::setRawVectorField | ( | dmpvecarr3E * | rawVectorField | ) |
It sets the vector field optionally attached to point cloud raw list
- Parameters
-
[in] rawVectorField raw vector field.
Definition at line 159 of file CreatePointCloud.cpp.
◆ setRawVectorField() [2/2]
void mimmo::CreatePointCloud::setRawVectorField | ( | dvecarr3E | rawVectorField | ) |
It sets the vector field optionally attached to point cloud raw list
- Parameters
-
[in] rawVectorField raw vector field.
Definition at line 168 of file CreatePointCloud.cpp.
◆ swap()
|
protectednoexcept |
Swap method
- Parameters
-
[in] x object to be swapped
Definition at line 78 of file CreatePointCloud.cpp.
Member Data Documentation
◆ m_rawpoints
|
protected |
input Cloud points list, in raw format.
Definition at line 97 of file CreatePointCloud.hpp.
◆ m_rawscalar
|
protected |
input scalar attached to Cloud points, in raw format.
Definition at line 98 of file CreatePointCloud.hpp.
◆ m_rawvector
|
protected |
input vector attached to Cloud points, in raw format
Definition at line 99 of file CreatePointCloud.hpp.
◆ m_scalarfield
|
protected |
MimmoPiercedVector scalar field
Definition at line 94 of file CreatePointCloud.hpp.
◆ m_vectorfield
|
protected |
MimmoPiercedVector vector field
Definition at line 95 of file CreatePointCloud.hpp.
The documentation for this class was generated from the following files:
- src/iogeneric/CreatePointCloud.hpp
- src/iogeneric/CreatePointCloud.cpp
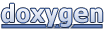