CreatePointCloud.cpp
96 built = (built && createPortIn<dvecarr3E, CreatePointCloud>(this, &mimmo::CreatePointCloud::setRawPoints, M_COORDS, true,1));
97 built = (built && createPortIn<dvecarr3E, CreatePointCloud>(this, &mimmo::CreatePointCloud::setRawVectorField, M_DISPLS));
98 built = (built && createPortIn<dvector1D, CreatePointCloud>(this, &mimmo::CreatePointCloud::setRawScalarField, M_DATAFIELD));
100 built = (built && createPortIn<dmpvecarr3E*, CreatePointCloud>(this, &mimmo::CreatePointCloud::setRawPoints, M_VECTORFIELD, true,1));
101 built = (built && createPortIn<dmpvecarr3E*, CreatePointCloud>(this, &mimmo::CreatePointCloud::setRawVectorField, M_VECTORFIELD2));
102 built = (built && createPortIn<dmpvector1D*, CreatePointCloud>(this, &mimmo::CreatePointCloud::setRawScalarField, M_SCALARFIELD));
104 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, CreatePointCloud>(this, &mimmo::CreatePointCloud::getGeometry, M_GEOM));
105 built = (built && createPortOut<dmpvector1D*, CreatePointCloud>(this, &mimmo::CreatePointCloud::getScalarField, M_SCALARFIELD));
106 built = (built && createPortOut<dmpvecarr3E*, CreatePointCloud>(this, &mimmo::CreatePointCloud::getVectorField, M_VECTORFIELD));
void plotOptionalResults()
Definition: CreatePointCloud.cpp:313
void setRawVectorField(dvecarr3E rawVectorField)
Definition: CreatePointCloud.cpp:168
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
dmpvecarr3E * getVectorField()
Definition: CreatePointCloud.cpp:125
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: CreatePointCloud.cpp:300
dmpvector1D * getScalarField()
Definition: CreatePointCloud.cpp:116
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: CreatePointCloud.cpp:287
void setRawPoints(dvecarr3E rawPoints)
Definition: CreatePointCloud.cpp:144
void initialize(MimmoSharedPointer< MimmoObject >, MPVLocation, const mpv_t &)
Definition: MimmoPiercedVector.tpp:593
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
@ POINT
CreatePointCloud & operator=(CreatePointCloud other)
Definition: CreatePointCloud.cpp:69
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(CreatePointCloud &x) noexcept
Definition: CreatePointCloud.cpp:78
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
CreatePointCloud manages cloud point data in raw format to create a MimmoObject Point Cloud container...
Definition: CreatePointCloud.hpp:91
void setRawScalarField(dvector1D rawScalarField)
Definition: CreatePointCloud.cpp:192
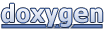