BasicMeshes.hpp
Abstract Interface class for Elementary Shape Representation.
Definition: BasicShapes.hpp:91
@ CUBE
void plotCloudScalar(std::string, std::string, int, bool, dvector1D &data)
Definition: BasicMeshes.cpp:1448
void reshapeNodalStructure()
Definition: BasicMeshes.cpp:1598
dvecarr3E getGlobalCellCentroids()
Definition: BasicMeshes.cpp:526
CoordType
Specify type of conditions to distribute NURBS nodes in a given coordinate of the shape.
Definition: BasicShapes.hpp:49
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicMeshes.cpp:619
int accessCellIndex(int i, int j, int k)
Definition: BasicMeshes.cpp:1049
dvecarr3E getLocalCellCentroids()
Definition: BasicMeshes.cpp:513
void destroyNodalStructure()
Definition: BasicMeshes.cpp:1585
void locateCellByPoint(darray3E &point, int &i, int &j, int &k)
Definition: BasicMeshes.cpp:1018
int accessPointIndex(int i, int j, int k)
Definition: BasicMeshes.hpp:204
void setShape(ShapeType type=ShapeType::CUBE)
Definition: BasicMeshes.cpp:713
std::array< CoordType, 3 > getCoordType()
Definition: BasicMeshes.cpp:316
void setMesh(darray3E &origin, darray3E &span, ShapeType, iarray3E &dimensions)
Definition: BasicMeshes.cpp:874
darray3E transfToLocal(darray3E &point)
Definition: BasicMeshes.cpp:1121
darray3E transfToGlobal(darray3E &point)
Definition: BasicMeshes.cpp:1090
void plotGridScalar(std::string, std::string, int, bool, dvector1D &data)
Definition: BasicMeshes.cpp:1544
ShapeType
Identifies the type of elemental shape supported by BasicShape class.
Definition: BasicShapes.hpp:38
double interpolateCellData(darray3E &point, dvector1D &celldata)
Definition: BasicMeshes.cpp:1152
double interpolatePointData(darray3E &point, dvector1D &pointdata)
Definition: BasicMeshes.cpp:1266
void plotCloud(std::string &, std::string, int, bool, const ivector1D &labels, dvecarr3E *extPoints=nullptr)
Definition: BasicMeshes.cpp:1396
UStructMesh & operator=(UStructMesh other)
Definition: BasicMeshes.cpp:128
void plotGrid(std::string &, std::string, int, bool, const ivector1D &labels, dvecarr3E *extPoints=nullptr)
Definition: BasicMeshes.cpp:1492
void setInfLimits(double val, int dir)
Definition: BasicMeshes.cpp:588
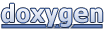