utils_example_00004.cpp
Comparison of Oriented Bounding Boxes between a deformed and undeformed geometry.Using: MimmoGeometry, OBBox, TranslationPoint, RotationAxes, FFDLattice, Chain, Partition(MPI version)
To run : ./utils_example_00004
To run (MPI version): mpirun -np X utils_example_00004
visit: mimmo website
/*---------------------------------------------------------------------------*\
*
* mimmo
*
* Copyright (C) 2015-2021 OPTIMAD engineering Srl
*
* -------------------------------------------------------------------------
* License
* This file is part of mimmo.
*
* mimmo is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License v3 (LGPL)
* as published by the Free Software Foundation.
*
* mimmo is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with mimmo. If not, see <http://www.gnu.org/licenses/>.
*
\ *---------------------------------------------------------------------------*/
#include "mimmo_utils.hpp"
#include "FFDLattice.hpp"
#if MIMMO_ENABLE_MPI
#include "Partition.hpp"
#endif
// =================================================================================== //
void test00004() {
/*
Read a target bunny geometry from file. CONVERT option will let the block to write
the just read file in another file, immediately after the reading.
*/
mimmo0->setReadFileType(FileType::STL);
mimmo0->setWriteFileType(FileType::SURFVTU);
#if MIMMO_ENABLE_MPI
/* Distribute bunny among processes.
*/
mimmo::Partition * mimmo0part = new mimmo::Partition();
mimmo0part->setPartitionMethod(mimmo::PartitionMethod::PARTGEOM);
mimmo0part->setName("StanfordBunnyPartitioner");
mimmo0part->setPlotInExecution(true);
#endif
/*
Calculate the OBB the original StanfordBunny
*/
obb_original->setName("OBBOriginal");
/*
Calculate the OBB the deformed StanfordBunny
*/
obb_deformed->setName("OBBDeformed");
obb_deformed->setWriteInfo(true);
obb_deformed->setPlotInExecution(true);
/*
Translate point. This is meant as the new origin of the FFDLattice for deformation
*/
translp->setName("TranslationOriginLattice");
translp->setOrigin({{0.0,0.0,0.0}});
translp->setDirection({{-0.714,0.0,1.0}});
translp->setTranslation(0.9);
/*
Rotate axes reference system. This is meant as the new sdr axes of the FFDLattice for deformation
*/
rot_axes->setName("SDRAxesLattice");
rot_axes->setOrigin({{0.0,0.0,0.0}});
rot_axes->setDirection({{0.0,0.0,1.0}});
rot_axes->setRotation(-30.0*BITPIT_PI/180.0);
rot_axes->setAxes({{1.0,0.0,0.0,0.0,1.0,0.0,0.0,0.0,1.0}});
rot_axes->setAxesOrigin({{0.0,0.0,0.0}});
/*
Create a box shaped FFD Lattice to deform the head of the rabbit.
Axes and Origin of the cube are provided by rot_axes and translp
through ports.
*/
latt->setName("FFDLattice");
latt->setSpan({{0.7,1.2,0.62}});
dvecarr3E displs(8,{{0.0,0.0,0.0}});
displs[1][0] = -0.5;
displs[3][0] = -0.5;
latt->setDisplacements(displs);
latt->setPlotInExecution(true);
// create connections
// original geoemetry passed to obb and lattice
#if MIMMO_ENABLE_MPI
#else
#endif
// origin, axes passed to lattice
// pass deformed geoemetry to obb_deformed
/* Setup execution chain.
*/
mimmo::Chain ch0,ch1;
ch0.addObject(mimmo0);
ch0.addObject(obb_original);
#if MIMMO_ENABLE_MPI
ch0.addObject(mimmo0part);
#endif
ch1.addObject(translp);
ch1.addObject(rot_axes);
ch1.addObject(latt);
ch1.addObject(obb_deformed);
/* Execute the chain.
* Use debug flag false to avoid to print out the execution steps on console.
*/
ch1.exec(true);
/*
Write deformed geometry;
*/
/* Clean up & exit;
*/
delete mimmo0;
delete translp;
delete rot_axes;
delete obb_original;
delete obb_deformed;
delete latt;
#if MIMMO_ENABLE_MPI
delete mimmo0part;
#endif
return;
}
int main(int argc, char *argv[]) {
BITPIT_UNUSED(argc);
BITPIT_UNUSED(argv);
#if MIMMO_ENABLE_MPI==1
MPI_Init(&argc, &argv);
{
#endif
try{
test00004() ;
}
catch(std::exception & e){
std::cout<<"utils_example_00004 exited with an error of type : "<<e.what()<<std::endl;
return 1;
}
#if MIMMO_ENABLE_MPI==1
}
MPI_Finalize();
#endif
return 0;
}
@ CUBE
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setDisplacements(dvecarr3E displacements)
Definition: FFDLattice.cpp:284
void setAxesOrigin(darray3E axes_origin)
Definition: RotationAxes.cpp:198
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setTranslation(double alpha)
Definition: TranslationPoint.cpp:145
RotationAxes is the class that applies a rotation to a given reference system.
Definition: RotationAxes.hpp:82
void setShape(ShapeType type=ShapeType::CUBE)
Definition: BasicMeshes.cpp:713
TranslationPoint is the class that applies the a translation to a point.
Definition: TranslationPoint.hpp:73
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void setDirection(darray3E direction)
Definition: TranslationPoint.cpp:133
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
void setDirection(darray3E direction)
Definition: RotationAxes.cpp:170
void setOrigin(darray3E origin)
Definition: TranslationPoint.cpp:153
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
Free Form Deformation of a 3D surface and point clouds, with structured lattice.
Definition: FFDLattice.hpp:133
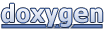