TranslationPoint is the class that applies the a translation to a point. More...
#include <TranslationPoint.hpp>
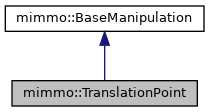
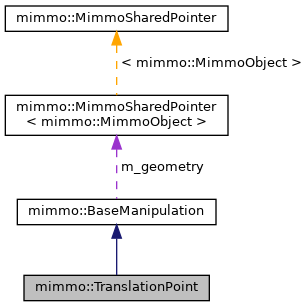
Public Member Functions | |
TranslationPoint (const bitpit::Config::Section &rootXML) | |
TranslationPoint (const TranslationPoint &other) | |
TranslationPoint (darray3E direction={ {0, 0, 0} }) | |
~TranslationPoint () | |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
darray3E | getDirection () |
darray3E | getOrigin () |
darray3E | getTranslatedOrigin () |
double | getTranslation () |
void | setDirection (darray3E direction) |
void | setOrigin (darray3E origin) |
void | setTranslation (double alpha) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | swap (TranslationPoint &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
double | m_alpha |
darray3E | m_direction |
darray3E | m_origin |
darray3E | m_translated |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
TranslationPoint is the class that applies the a translation to a point.
Here the point is supposed to be an origin of a reference system. The point is translated over a direction and for a quantity set by the user or an external input. Result of the translation are saved in result of base class and in the modified member m_origin.
Ports available in GenericInput Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_AXIS | setDirection | (MC_ARRAY3, MD_FLOAT) |
M_VALUED | setTranslation | (MC_SCALAR, MD_FLOAT) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_POINT | getOrigin | (MC_ARRAY3, MD_FLOAT) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.TranslationPoint
; - Priority: uint marking priority in multi-chain execution;
Proper of the class:
- Origin: point that need to be translated;
- Direction: translation direction;
- Translation: entity of translation.
- Examples
- utils_example_00004.cpp.
Definition at line 73 of file TranslationPoint.hpp.
Constructor & Destructor Documentation
◆ TranslationPoint() [1/3]
mimmo::TranslationPoint::TranslationPoint | ( | darray3E | direction = { {0, 0, 0} } | ) |
Default constructor of TranslationPoint
Definition at line 31 of file TranslationPoint.cpp.
◆ TranslationPoint() [2/3]
mimmo::TranslationPoint::TranslationPoint | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 41 of file TranslationPoint.cpp.
◆ ~TranslationPoint()
mimmo::TranslationPoint::~TranslationPoint | ( | ) |
Default destructor of TranslationPoint
Definition at line 59 of file TranslationPoint.cpp.
◆ TranslationPoint() [3/3]
mimmo::TranslationPoint::TranslationPoint | ( | const TranslationPoint & | other | ) |
Copy constructor of TranslationPoint.
Definition at line 63 of file TranslationPoint.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 174 of file TranslationPoint.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 86 of file TranslationPoint.cpp.
◆ execute()
|
virtual |
Execution command. It modifies the coordinates of the origin with the translation conditions. The result of the translation is stored in member result of base class and in the member m_origin. After exec() the original point coordinates will be permanently modified.
Implements mimmo::BaseManipulation.
Definition at line 164 of file TranslationPoint.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 221 of file TranslationPoint.cpp.
◆ getDirection()
darray3E mimmo::TranslationPoint::getDirection | ( | ) |
It gets the direction of the translation.
- Returns
- Direction of translation.
Definition at line 101 of file TranslationPoint.cpp.
◆ getOrigin()
darray3E mimmo::TranslationPoint::getOrigin | ( | ) |
It gets the original position of the point to be translated
- Returns
- Position of the point.
Definition at line 117 of file TranslationPoint.cpp.
◆ getTranslatedOrigin()
darray3E mimmo::TranslationPoint::getTranslatedOrigin | ( | ) |
It gets the position of the translated point (after the execution of the object).
- Returns
- Position of the translated point.
Definition at line 125 of file TranslationPoint.cpp.
◆ getTranslation()
double mimmo::TranslationPoint::getTranslation | ( | ) |
It gets the value of the translation.
- Returns
- Value of translation.
Definition at line 109 of file TranslationPoint.cpp.
◆ setDirection()
void mimmo::TranslationPoint::setDirection | ( | darray3E | direction | ) |
It sets the direction of the translation.
- Parameters
-
[in] direction Direction of translation.
- Examples
- utils_example_00004.cpp.
Definition at line 133 of file TranslationPoint.cpp.
◆ setOrigin()
void mimmo::TranslationPoint::setOrigin | ( | darray3E | origin | ) |
It sets the original coordinates of the point to be translated.
- Parameters
-
[in] origin Position of the point.
- Examples
- utils_example_00004.cpp.
Definition at line 153 of file TranslationPoint.cpp.
◆ setTranslation()
void mimmo::TranslationPoint::setTranslation | ( | double | alpha | ) |
It sets the value of the translation.
- Parameters
-
[in] alpha Value of translation.
- Examples
- utils_example_00004.cpp.
Definition at line 145 of file TranslationPoint.cpp.
◆ swap()
|
protectednoexcept |
Swap Function
- Parameters
-
[in] x object to be swapped
Definition at line 73 of file TranslationPoint.cpp.
Member Data Documentation
◆ m_alpha
|
protected |
Value of translation.
Definition at line 78 of file TranslationPoint.hpp.
◆ m_direction
|
protected |
Components of the translation axis.
Definition at line 76 of file TranslationPoint.hpp.
◆ m_origin
|
protected |
Origin of box to be deformed.
Definition at line 77 of file TranslationPoint.hpp.
◆ m_translated
|
protected |
Value translated
Definition at line 79 of file TranslationPoint.hpp.
The documentation for this class was generated from the following files:
- src/utils/TranslationPoint.hpp
- src/utils/TranslationPoint.cpp
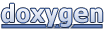