TranslationPoint.cpp
88 built = (built && createPortIn<darray3E, TranslationPoint>(this, &mimmo::TranslationPoint::setOrigin, M_POINT));
89 built = (built && createPortIn<darray3E, TranslationPoint>(this, &mimmo::TranslationPoint::setDirection, M_AXIS));
90 built = (built && createPortIn<double, TranslationPoint>(this, &mimmo::TranslationPoint::setTranslation, M_VALUED));
91 built = (built && createPortOut<darray3E, TranslationPoint>(this, &mimmo::TranslationPoint::getTranslatedOrigin, M_POINT));
92 // built = (built && createPortOut<darray3E, TranslationPoint>(this, &mimmo::TranslationPoint::getDirection, M_AXIS));
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: TranslationPoint.cpp:174
void setTranslation(double alpha)
Definition: TranslationPoint.cpp:145
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
TranslationPoint(darray3E direction={ {0, 0, 0} })
Definition: TranslationPoint.cpp:31
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
TranslationPoint is the class that applies the a translation to a point.
Definition: TranslationPoint.hpp:73
void setDirection(darray3E direction)
Definition: TranslationPoint.cpp:133
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: TranslationPoint.cpp:221
void setOrigin(darray3E origin)
Definition: TranslationPoint.cpp:153
darray3E getTranslatedOrigin()
Definition: TranslationPoint.cpp:125
void swap(TranslationPoint &x) noexcept
Definition: TranslationPoint.cpp:73
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
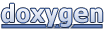