RotationAxes.cpp
138 built = (built && createPortIn<darray3E, RotationAxes>(this, &mimmo::RotationAxes::setOrigin, M_POINT));
139 built = (built && createPortIn<darray3E, RotationAxes>(this, &mimmo::RotationAxes::setDirection, M_AXIS));
140 built = (built && createPortIn<double, RotationAxes>(this, &mimmo::RotationAxes::setRotation, M_VALUED));
141 built = (built && createPortIn<darray3E, RotationAxes>(this, &mimmo::RotationAxes::setAxesOrigin, M_POINT2));
142 built = (built && createPortIn<dmatrix33E, RotationAxes>(this, &mimmo::RotationAxes::setAxes, M_AXES));
143 built = (built && createPortOut<darray3E, RotationAxes>(this, &mimmo::RotationAxes::getRotatedOrigin, M_POINT));
144 built = (built && createPortOut<dmatrix33E, RotationAxes>(this, &mimmo::RotationAxes::getRotatedAxes, M_AXES));
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationAxes.cpp:330
void setAxesOrigin(darray3E axes_origin)
Definition: RotationAxes.cpp:198
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
RotationAxes is the class that applies a rotation to a given reference system.
Definition: RotationAxes.hpp:82
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationAxes.cpp:253
RotationAxes & operator=(RotationAxes other)
Definition: RotationAxes.cpp:112
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void setDirection(darray3E direction)
Definition: RotationAxes.cpp:170
RotationAxes(darray3E origin={ {0, 0, 0} }, darray3E direction={ {0, 0, 0} })
Definition: RotationAxes.cpp:32
void setAxis(darray3E origin, darray3E direction)
Definition: RotationAxes.cpp:153
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
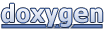