RotationAxes.hpp
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationAxes.cpp:330
void setAxesOrigin(darray3E axes_origin)
Definition: RotationAxes.cpp:198
RotationAxes is the class that applies a rotation to a given reference system.
Definition: RotationAxes.hpp:82
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: RotationAxes.cpp:253
RotationAxes & operator=(RotationAxes other)
Definition: RotationAxes.cpp:112
void setDirection(darray3E direction)
Definition: RotationAxes.cpp:170
RotationAxes(darray3E origin={ {0, 0, 0} }, darray3E direction={ {0, 0, 0} })
Definition: RotationAxes.cpp:32
void setAxis(darray3E origin, darray3E direction)
Definition: RotationAxes.cpp:153
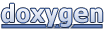