geohandlers_example_00002.cpp
Example of usage of stitching block of two input geometries.Using: MimmoGeometry, StitchGeometry, Chain, Partition(MPI version)
To run : ./geohandlers_example_00002
To run (MPI version): mpirun -np X geohandlers_example_00002
visit: mimmo website
/*---------------------------------------------------------------------------*\
*
* mimmo
*
* Copyright (C) 2015-2021 OPTIMAD engineering Srl
*
* -------------------------------------------------------------------------
* License
* This file is part of mimmo.
*
* mimmo is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License v3 (LGPL)
* as published by the Free Software Foundation.
*
* mimmo is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with mimmo. If not, see <http://www.gnu.org/licenses/>.
*
\ *---------------------------------------------------------------------------*/
#include "mimmo_geohandlers.hpp"
#if MIMMO_ENABLE_MPI
#include "mimmo_parallel.hpp"
#endif
// =================================================================================== //
// =================================================================================== //
void test00002() {
/*
Read a sphere from STL file. Convert mode is to save the just read geometry in
another file with name geohandlers_output_00002.0000.stl
*/
mimmo0->setReadFileType(FileType::STL);
mimmo0->setWriteFileType(FileType::STL);
/*
Read the Stanford bunny from STL file. Convert mode is to save the just read geometry in
another file with name geohandlers_output_00002.0001.stl
*/
mimmo1->setReadDir("geodata");
mimmo1->setReadFilename("stanfordBunny2");
mimmo1->setReadFileType(FileType::STL);
mimmo1->setWriteDir("./");
mimmo1->setWriteFileType(FileType::STL);
mimmo1->setWriteFilename("geohandlers_output_00002.0001");
/*
Write the stiched geometry to STL file.
*/
mimmo2->setWriteDir("./");
mimmo2->setWriteFileType(FileType::STL);
mimmo2->setWriteFilename("geohandlers_output_00002.0002");
#if MIMMO_ENABLE_MPI
/* Block to distribute among processors the sphere geometry */
mimmo::Partition* partition0 = new mimmo::Partition();
partition0->setPartitionMethod(mimmo::PartitionMethod::PARTGEOM);
partition0->setPlotInExecution(true);
/* Block to distribute among processors the bunny geometry */
mimmo::Partition* partition1 = new mimmo::Partition();
partition1->setPartitionMethod(mimmo::PartitionMethod::PARTGEOM);
partition1->setPlotInExecution(true);
#endif
/*
Stitcher of multiple geometries.
* Plot Optional results during execution active for Stitcher block.
*/
/*
Setup block pin connections.
*/
#if MIMMO_ENABLE_MPI
#else
#endif
/*
Setup execution chain.
*/
mimmo::Chain ch0;
ch0.addObject(mimmo0);
ch0.addObject(mimmo1);
#if MIMMO_ENABLE_MPI
ch0.addObject(partition0);
ch0.addObject(partition1);
#endif
ch0.addObject(stitcher);
ch0.addObject(mimmo2);
/*
Execution the chain.
Use debug flag true to to print out the execution steps.
*/
/* Clean up & exit;
*/
delete mimmo0;
delete mimmo1;
#if MIMMO_ENABLE_MPI
delete partition0;
delete partition1;
#endif
delete mimmo2;
delete stitcher;
return;
}
// =================================================================================== //
int main( int argc, char *argv[] ) {
BITPIT_UNUSED(argc);
BITPIT_UNUSED(argv);
#if MIMMO_ENABLE_MPI
MPI_Init(&argc, &argv);
#endif
try{
test00002() ;
}
catch(std::exception & e){
std::cout<<"geohandlers_example_00002 exited with an error of type : "<<e.what()<<std::endl;
return 1;
}
#if MIMMO_ENABLE_MPI
MPI_Finalize();
#endif
return 0;
}
Chain is the class used to manage the chain execution of multiple executable blocks (manipulation obj...
Definition: Chain.hpp:48
void setWriteFileType(FileType type)
Definition: MimmoGeometry.cpp:230
void setOutputPlot(std::string path)
Definition: BaseManipulation.cpp:453
void setWriteFilename(std::string filename)
Definition: MimmoGeometry.cpp:268
void setReadFilename(std::string filename)
Definition: MimmoGeometry.cpp:221
bool addPin(BaseManipulation *objSend, BaseManipulation *objRec, PortID portS, PortID portR, bool forced)
Definition: MimmoNamespace.cpp:68
MimmoGeometry is an executable block class wrapping(linking or internally instantiating) a Mimmo Obje...
Definition: MimmoGeometry.hpp:151
StitchGeometry is an executable block class capable of stitch multiple MimmoObject geometries of the ...
Definition: StitchGeometry.hpp:72
void setPlotInExecution(bool)
Definition: BaseManipulation.cpp:443
void setReadFileType(FileType type)
Definition: MimmoGeometry.cpp:192
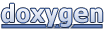