Triangulate a target MimmoObject non-homogeneous and/or non-triangular surface mesh. More...
#include <SurfaceTriangulator.hpp>
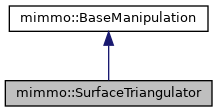
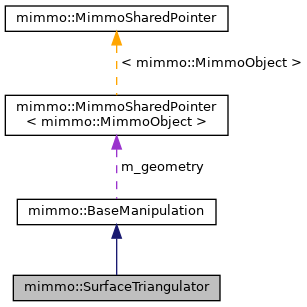
Protected Member Functions | |
void | swap (SurfaceTriangulator &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Triangulate a target MimmoObject non-homogeneous and/or non-triangular surface mesh.
Class force a given 3D surface tessellation to be a full homogeneous triangular one. By default class create a new indipendent copy of the target geometry and triangulate it. A workOnTarget option can be activated if the User want to apply modifications directly on the target mesh.
Ports available in SurfaceTriangulator Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName : name of the class as
mimmo.SurfaceTriangulator
; - Priority : uint marking priority in multi-chain execution;
- PlotInExecution : plot optional results in execution;
- OutputPlot : path to store optional results.
Proper of the class:
- WorkOnTarget : 0 -create a new homogeneously triangulated and independent copy of target mesh, 1 - modify directly the target mesh;
Geometry has to be mandatorily passed through ports.
- Examples
- geohandlers_example_00004.cpp.
Definition at line 74 of file SurfaceTriangulator.hpp.
Constructor & Destructor Documentation
◆ SurfaceTriangulator() [1/3]
mimmo::SurfaceTriangulator::SurfaceTriangulator | ( | ) |
Constructor
Definition at line 32 of file SurfaceTriangulator.cpp.
◆ SurfaceTriangulator() [2/3]
mimmo::SurfaceTriangulator::SurfaceTriangulator | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 42 of file SurfaceTriangulator.cpp.
◆ ~SurfaceTriangulator()
|
virtual |
Destructor;
Definition at line 60 of file SurfaceTriangulator.cpp.
◆ SurfaceTriangulator() [3/3]
mimmo::SurfaceTriangulator::SurfaceTriangulator | ( | const SurfaceTriangulator & | other | ) |
Copy constructor. Internal geometry data structure is not copied.
Definition at line 65 of file SurfaceTriangulator.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 170 of file SurfaceTriangulator.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 91 of file SurfaceTriangulator.cpp.
◆ execute()
|
virtual |
Triangulate your target non-homogeneous surface.
Implements mimmo::BaseManipulation.
Definition at line 139 of file SurfaceTriangulator.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 195 of file SurfaceTriangulator.cpp.
◆ getGeometry()
mimmo::MimmoSharedPointer< MimmoObject > mimmo::SurfaceTriangulator::getGeometry | ( | ) |
- Returns
- pointer to geometry resulting by class manipulation
Definition at line 103 of file SurfaceTriangulator.cpp.
◆ isWorkingOnTarget()
bool mimmo::SurfaceTriangulator::isWorkingOnTarget | ( | ) |
- Returns
- true if the class is directly applying on target mesh, false if the class is creating a newly indipendent patch.
Definition at line 112 of file SurfaceTriangulator.cpp.
◆ operator=()
SurfaceTriangulator & mimmo::SurfaceTriangulator::operator= | ( | SurfaceTriangulator | other | ) |
Assignment operator of the class. Copy class data as relative copy constructor.
Definition at line 72 of file SurfaceTriangulator.cpp.
◆ plotOptionalResults()
|
virtual |
Plot optional results during execution, that is the newly triangulated surface
Reimplemented from mimmo::BaseManipulation.
Definition at line 159 of file SurfaceTriangulator.cpp.
◆ setGeometry()
void mimmo::SurfaceTriangulator::setGeometry | ( | mimmo::MimmoSharedPointer< MimmoObject > | geo | ) |
Set reference geometry for your SurfaceTriangulator class. Geometry must be a surface tessellation.
- Parameters
-
[in] geo pointer to target geometry
Definition at line 121 of file SurfaceTriangulator.cpp.
◆ setWorkOnTarget()
void mimmo::SurfaceTriangulator::setWorkOnTarget | ( | bool | flag = false | ) |
If true, activate option to apply triangulator directly on the target geometry object linked to the class with setGeometry method. If false, create internally an indipendent clone of the target geometry and work on it. False is default.
- Parameters
-
[in] flag activation flag
Definition at line 132 of file SurfaceTriangulator.cpp.
◆ swap()
|
protectednoexcept |
Swap function.
- Parameters
-
[in] x object to be swapped
Definition at line 81 of file SurfaceTriangulator.cpp.
The documentation for this class was generated from the following files:
- src/geohandlers/SurfaceTriangulator.hpp
- src/geohandlers/SurfaceTriangulator.cpp
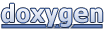