SurfaceTriangulator.cpp
65 SurfaceTriangulator::SurfaceTriangulator(const SurfaceTriangulator & other):BaseManipulation(other){
94 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, SurfaceTriangulator>(this, &mimmo::SurfaceTriangulator::setGeometry,M_GEOM, true));
95 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, SurfaceTriangulator>(this, &mimmo::SurfaceTriangulator::getGeometry, M_GEOM));
170 void SurfaceTriangulator::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
void swap(SurfaceTriangulator &x) noexcept
Definition: SurfaceTriangulator.cpp:81
virtual void plotOptionalResults()
Definition: SurfaceTriangulator.cpp:159
SurfaceTriangulator & operator=(SurfaceTriangulator other)
Definition: SurfaceTriangulator.cpp:72
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SurfaceTriangulator.cpp:195
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
bool isWorkingOnTarget()
Definition: SurfaceTriangulator.cpp:112
SurfaceTriangulator()
Definition: SurfaceTriangulator.cpp:32
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SurfaceTriangulator.cpp:170
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual ~SurfaceTriangulator()
Definition: SurfaceTriangulator.cpp:60
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void setGeometry(mimmo::MimmoSharedPointer< MimmoObject > geo)
Definition: SurfaceTriangulator.cpp:121
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
Triangulate a target MimmoObject non-homogeneous and/or non-triangular surface mesh.
Definition: SurfaceTriangulator.hpp:74
void setWorkOnTarget(bool flag=false)
Definition: SurfaceTriangulator.cpp:132
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
mimmo::MimmoSharedPointer< MimmoObject > getGeometry()
Definition: SurfaceTriangulator.cpp:103
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
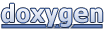