ClipGeometry is a class that clip a 3D geometry according to a plane intersecting it. More...
#include <ClipGeometry.hpp>
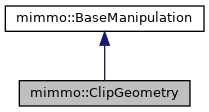
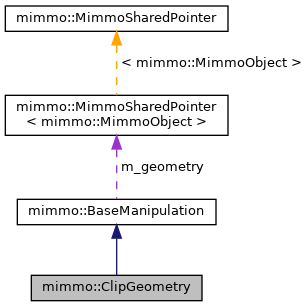
Protected Member Functions | |
virtual void | plotOptionalResults () |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
ClipGeometry is a class that clip a 3D geometry according to a plane intersecting it.
ClipGeometry is derived from BaseManipulation class. It needs a target MimmoObject geometry, alongside a clipping plane definition through its origin and normal or by a set af value [a,b,c,d], describing its implicit form a*x + b*y + c*z + d = 0; It returns geometry clipped in an independent MimmoObject. The class controls clipping direction (based on normal direction) with an "insideout" boolean. ClipGeometry plots as optional result the clipped portion of input geometry.
Ports available in ClipGeometry Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_PLANE | setClipPlane | (MC_ARRAY4, MD_FLOAT) |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_AXIS | setNormal | (MC_ARRAY3, MD_FLOAT) |
M_VALUEB | setInsideOut | (MC_SCALAR, MD_BOOL) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getClippedPatch | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.ClipGeometry
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution : boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing.
Proper of the class:
- InsideOut: boolean 0/1 to get direction of clipping according to given plane normal;
- ClipPlane: section defining the plane's normal and a point belonging to it:
<ClipPlane>
;
<Point> 0.0 0.0 0.0 </Point>
<Normal> 0.0 1.0 0.0 </Normal>
</ClipPlane>
Geometry has to be mandatorily passed through port.
- Examples
- geohandlers_example_00004.cpp.
Definition at line 85 of file ClipGeometry.hpp.
Constructor & Destructor Documentation
◆ ClipGeometry() [1/3]
mimmo::ClipGeometry::ClipGeometry | ( | ) |
Default constructor of ClipGeometry
Definition at line 30 of file ClipGeometry.cpp.
◆ ClipGeometry() [2/3]
mimmo::ClipGeometry::ClipGeometry | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 44 of file ClipGeometry.cpp.
◆ ~ClipGeometry()
mimmo::ClipGeometry::~ClipGeometry | ( | ) |
Default destructor of ClipGeometry
Definition at line 66 of file ClipGeometry.cpp.
◆ ClipGeometry() [3/3]
mimmo::ClipGeometry::ClipGeometry | ( | const ClipGeometry & | other | ) |
Copy constructor of ClipGeometry.
Definition at line 70 of file ClipGeometry.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 313 of file ClipGeometry.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 81 of file ClipGeometry.cpp.
◆ execute()
|
virtual |
Execution command. Clip geometry and save result in m_clipped member.
Implements mimmo::BaseManipulation.
Definition at line 191 of file ClipGeometry.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 361 of file ClipGeometry.cpp.
◆ getClippedPatch()
MimmoSharedPointer< MimmoObject > mimmo::ClipGeometry::getClippedPatch | ( | ) |
It gets copy of a pointer to the clipped geometry treated as an indipendent MimmoObject (owned by the class).
- Returns
- pointer to MimmoObject clipped patch
Definition at line 111 of file ClipGeometry.cpp.
◆ getClipPlane()
darray4E mimmo::ClipGeometry::getClipPlane | ( | ) |
It gets coefficients of the clipping plane in its implicit form a*x+b*y+c*z+d=0
- Returns
- pointer to MimmoObject clipped patch
Definition at line 121 of file ClipGeometry.cpp.
◆ isInsideOut()
bool mimmo::ClipGeometry::isInsideOut | ( | ) |
It gets direction for clipping. If false it takes all parts of target geometry lying on the half positive space delimited by the plane (where plane normal pointing), true the exact opposite.
- Returns
- insideout flag
Definition at line 101 of file ClipGeometry.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
It plots optional result of the class in execution, that is the clipped geometry as standard vtk unstructured grid.
Reimplemented from mimmo::BaseManipulation.
Definition at line 301 of file ClipGeometry.cpp.
◆ setClipPlane() [1/2]
It sets plane for clipping by point and normal
- Parameters
-
[in] origin point belonging to plane [in] normal plane normal
Definition at line 142 of file ClipGeometry.cpp.
◆ setClipPlane() [2/2]
void mimmo::ClipGeometry::setClipPlane | ( | darray4E | plane | ) |
It sets coefficients of the clipping plane in its implicit form a*x+b*y+c*z+d=0
- Parameters
-
[in] plane array of 4 coefficients a,b,c,d.
Definition at line 130 of file ClipGeometry.cpp.
◆ setGeometry()
void mimmo::BaseManipulation::setGeometry |
It sets the geometry linked by the manipulator object.
- Parameters
-
[in] geometry Pointer to geometry to be deformed by the manipulator object.
Definition at line 433 of file BaseManipulation.cpp.
◆ setInsideOut()
void mimmo::ClipGeometry::setInsideOut | ( | bool | flag | ) |
It sets direction for clipping. If false it takes all parts of target geometry lying on the half positive space delimited by the plane (where plane normal pointing), true the exact opposite.
- Parameters
-
[in] flag boolean
Definition at line 183 of file ClipGeometry.cpp.
◆ setNormal()
void mimmo::ClipGeometry::setNormal | ( | darray3E | normal | ) |
It sets normal of clipping plane
- Parameters
-
[in] normal plane normal
Definition at line 172 of file ClipGeometry.cpp.
◆ setOrigin()
void mimmo::ClipGeometry::setOrigin | ( | darray3E | origin | ) |
It sets origin of clipping plane
- Parameters
-
[in] origin point belonging to plane
Definition at line 162 of file ClipGeometry.cpp.
The documentation for this class was generated from the following files:
- src/geohandlers/ClipGeometry.hpp
- src/geohandlers/ClipGeometry.cpp
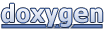