ClipGeometry.cpp
85 built = (built && createPortIn<darray4E, ClipGeometry>(this, &mimmo::ClipGeometry::setClipPlane, M_PLANE));
86 built = (built && createPortIn<darray3E, ClipGeometry>(this, &mimmo::ClipGeometry::setOrigin, M_POINT));
87 built = (built && createPortIn<darray3E, ClipGeometry>(this, &mimmo::ClipGeometry::setNormal, M_AXIS));
88 built = (built && createPortIn<bool, ClipGeometry>(this, &mimmo::ClipGeometry::setInsideOut, M_VALUEB));
89 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ClipGeometry>(this, &mimmo::ClipGeometry::setGeometry, M_GEOM, true));
91 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, ClipGeometry>(this, &mimmo::ClipGeometry::getClippedPatch, M_GEOM));
MimmoObject is the basic geometry container for mimmo library.
Definition: MimmoObject.hpp:143
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ClipGeometry.cpp:361
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void plotOptionalResults()
Definition: ClipGeometry.cpp:301
MimmoSharedPointer< MimmoObject > getClippedPatch()
Definition: ClipGeometry.cpp:111
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ClipGeometry.cpp:313
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
ClipGeometry is a class that clip a 3D geometry according to a plane intersecting it.
Definition: ClipGeometry.hpp:85
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
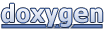