GenericDispls.cpp
114 built = (built && createPortIn<dvecarr3E, GenericDispls>(this, &mimmo::GenericDispls::setDispl, M_DISPLS, !m_read));
115 built = (built && createPortIn<livector1D, GenericDispls>(this, &mimmo::GenericDispls::setLabels, M_VECTORLI));
116 built = (built && createPortIn<int, GenericDispls>(this, &mimmo::GenericDispls::setNDispl, M_VALUEI));
118 built = (built && createPortOut<dvecarr3E, GenericDispls>(this, &mimmo::GenericDispls::getDispl, M_DISPLS));
119 built = (built && createPortOut<livector1D, GenericDispls>(this, &mimmo::GenericDispls::getLabels, M_VECTORLI));
120 built = (built && createPortOut<int, GenericDispls>(this, &mimmo::GenericDispls::getNDispl, M_VALUEI));
282 void GenericDispls::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
298 (*m_log)<< "warning in class "<<m_name<<": cannot absorb xml parameters for class IOmode mismatching"<<std::endl;
427 (*m_log)<<"error of "<<m_name<<" : cannot open "<<m_filename<< " requested. Exiting... "<<std::endl;
504 (*m_log)<<"error of "<<m_name<<" : cannot open "<<m_filename<< " requested. Exiting... "<<std::endl;
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericDispls.cpp:360
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
GenericDispls(bool readMode=true)
Definition: GenericDispls.cpp:34
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void setReadFilename(std::string filename)
Definition: GenericDispls.cpp:178
void setWriteFilename(std::string filename)
Definition: GenericDispls.cpp:198
GenericDispls is the class to read from file an initial set of displacements as a generic vector fiel...
Definition: GenericDispls.hpp:107
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: GenericDispls.cpp:282
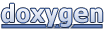