MeshChecker is the class to evaluate the quality of a volume mesh. More...
#include <MeshChecker.hpp>
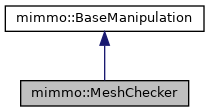

Public Types | |
enum | CMeshOutput { NOTRUN = -1, GOOD = 0, FACEVALIDITY = 1, VOLUMECHANGERATIO = 2, BOUNDARYSKEWNESS = 3, SKEWNESS = 4, MINIMUMVOLUME = 5, MAXIMUMVOLUME = 6 } |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
Protected Member Functions | |
bool | checkFaceValidity () |
CMeshOutput | checkMeshQuality () |
bool | checkSkewness () |
bool | checkVolume () |
void | clear () |
void | initializeVolumes () |
void | printResumeFile () |
void | setDefault () |
void | swap (MeshChecker &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
MeshChecker is the class to evaluate the quality of a volume mesh.
The available quality indices computed are :
- minimum and maximum cell volume
- maximum skweness angle inside the mesh and at boundaries
- minimum and maximum face validity
- minimum volume change ratio
It writes a resume of the quality mesh check directly on the mimmo::Logger. Optional results writes sick elements on file vtu.
Ports available in MeshChecker Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_VALUEB | isGood | (MC_SCALAR, MD_BOOL) |
M_VALUEI | getQualityStatusInt | (MC_SCALAR, MD_INT) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation/MimmoFvMesh:
- ClassName: name of the class as
mimmo.MeshChecker
; - Priority: uint marking priority in multi-chain execution;
Proper of the class:
- MinVolTOL: tolerance for minimum volume allowable.
- MaxVolTOL: tolerance for maximum volume allowable.
- MaxSkewTOL: tolerance for maximum skewness allowable.
- MaxBoundarySkewTOL: tolerance for maximum skewness on boundary allowable.
- MinFaceValidityTOL: tolerance for maximum skewness on boundary allowable.
- MinVolChangeTOL: tolerance for maximum skewness on boundary allowable.
- ResumeFile: boolean 1-print Resume on file, 0-do nothing.
Geometry has to be mandatorily passed by port.
- Examples
- iocgns_example_00002.cpp.
Definition at line 87 of file MeshChecker.hpp.
Constructor & Destructor Documentation
◆ MeshChecker() [1/3]
mimmo::MeshChecker::MeshChecker | ( | ) |
Constructor
Definition at line 33 of file MeshChecker.cpp.
◆ MeshChecker() [2/3]
mimmo::MeshChecker::MeshChecker | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 43 of file MeshChecker.cpp.
◆ ~MeshChecker()
|
virtual |
Destructor
Definition at line 61 of file MeshChecker.cpp.
◆ MeshChecker() [3/3]
mimmo::MeshChecker::MeshChecker | ( | const MeshChecker & | other | ) |
Copy Constructor. Result displacements are never copied.
- Parameters
-
[in] other MeshChecker where copy from
Definition at line 66 of file MeshChecker.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 723 of file MeshChecker.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 175 of file MeshChecker.cpp.
◆ checkFaceValidity()
|
protected |
It check the face validity of the cells of the mesh
- Returns
- false if face validity tolerance is not satisfied
Definition at line 563 of file MeshChecker.cpp.
◆ checkMeshQuality()
|
protected |
It check the quality of the mesh
- Returns
- error flag. If mesh is good return 0. Otherwise the error with highest priority. See enum CMeshOutput for errors and their priority.
Definition at line 291 of file MeshChecker.cpp.
◆ checkSkewness()
|
protected |
It check the quality of the skewness of the cells of the mesh
- Returns
- false if skewness or boundary skewness tolerance is not satisfied
Definition at line 478 of file MeshChecker.cpp.
◆ checkVolume()
|
protected |
It check the quality of the volume cells of the mesh
- Returns
- false if one of the volumes tolerance is not satisfied
Definition at line 391 of file MeshChecker.cpp.
◆ clear()
|
protected |
Clear auxiliary variables
Definition at line 663 of file MeshChecker.cpp.
◆ execute()
|
virtual |
Set Default Value
Implements mimmo::BaseManipulation.
Definition at line 147 of file MeshChecker.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 813 of file MeshChecker.cpp.
◆ getQualityStatus()
MeshChecker::CMeshOutput mimmo::MeshChecker::getQualityStatus | ( | ) |
- Returns
- quality flag (see class doc)
Definition at line 272 of file MeshChecker.cpp.
◆ getQualityStatusInt()
int mimmo::MeshChecker::getQualityStatusInt | ( | ) |
- Returns
- quality flag (see class doc)
Definition at line 281 of file MeshChecker.cpp.
◆ initializeVolumes()
|
protected |
Initialize cells volumes
Definition at line 650 of file MeshChecker.cpp.
◆ isGood()
bool mimmo::MeshChecker::isGood | ( | ) |
- Returns
- true is quality is good
Definition at line 263 of file MeshChecker.cpp.
◆ operator=()
MeshChecker & mimmo::MeshChecker::operator= | ( | MeshChecker | other | ) |
Assignement operator. Result displacements are never copied.
Definition at line 88 of file MeshChecker.cpp.
◆ plotOptionalResults()
|
virtual |
Plot mesh with failed check fields
Reimplemented from mimmo::BaseManipulation.
Definition at line 671 of file MeshChecker.cpp.
◆ printResumeFile()
|
protected |
Print resume file, of performing mesh check
Definition at line 859 of file MeshChecker.cpp.
◆ setDefault()
|
protected |
Set Default Value
Definition at line 122 of file MeshChecker.cpp.
◆ setGeometry()
void mimmo::MeshChecker::setGeometry | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Overload BaseManipulation setGeometry
- Parameters
-
[in] geo target geometry
Definition at line 187 of file MeshChecker.cpp.
◆ setMaximumBoundarySkewnessTolerance()
void mimmo::MeshChecker::setMaximumBoundarySkewnessTolerance | ( | double | tol | ) |
Set the tolerance in degrees
- Examples
- iocgns_example_00002.cpp.
Definition at line 226 of file MeshChecker.cpp.
◆ setMaximumSkewnessTolerance()
void mimmo::MeshChecker::setMaximumSkewnessTolerance | ( | double | tol | ) |
Set the tolerance in degrees
- Examples
- iocgns_example_00002.cpp.
Definition at line 217 of file MeshChecker.cpp.
◆ setMaximumVolumeTolerance()
void mimmo::MeshChecker::setMaximumVolumeTolerance | ( | double | tol | ) |
◆ setMinimumFaceValidityTolerance()
void mimmo::MeshChecker::setMinimumFaceValidityTolerance | ( | double | tol | ) |
◆ setMinimumVolumeChangeTolerance()
void mimmo::MeshChecker::setMinimumVolumeChangeTolerance | ( | double | tol | ) |
◆ setMinimumVolumeTolerance()
void mimmo::MeshChecker::setMinimumVolumeTolerance | ( | double | tol | ) |
◆ setPrintResumeFile()
void mimmo::MeshChecker::setPrintResumeFile | ( | bool | flag | ) |
set true to print resume file
Definition at line 253 of file MeshChecker.cpp.
◆ swap()
|
protectednoexcept |
Member Data Documentation
◆ m_facevalidity
|
protected |
INTERNAL USE Cells with poor face validity.
Definition at line 175 of file MeshChecker.hpp.
◆ m_isGood
|
protected |
true is good, false there are some errors
Definition at line 165 of file MeshChecker.hpp.
◆ m_maxFaceValidity
|
protected |
maxFaceValidity variable
Definition at line 154 of file MeshChecker.hpp.
◆ m_maxSkewness
|
protected |
maxSkewness variable
Definition at line 151 of file MeshChecker.hpp.
◆ m_maxSkewnessBoundary
|
protected |
maxSkewness on Boundary variable
Definition at line 152 of file MeshChecker.hpp.
◆ m_maxSkewnessBoundaryTol
|
protected |
check tolerance for maxSkewness on Boundary variable
Definition at line 161 of file MeshChecker.hpp.
◆ m_maxSkewnessTol
|
protected |
check tolerance for maxSkewness variable
Definition at line 160 of file MeshChecker.hpp.
◆ m_maxVolume
|
protected |
maxVolume variable
Definition at line 150 of file MeshChecker.hpp.
◆ m_maxVolumeTol
|
protected |
check tolerance for maxVolume variable
Definition at line 159 of file MeshChecker.hpp.
◆ m_minFaceValidity
|
protected |
minFaceValidity variable
Definition at line 153 of file MeshChecker.hpp.
◆ m_minFaceValidityTol
|
protected |
check tolerance for minFaceValidity variable
Definition at line 162 of file MeshChecker.hpp.
◆ m_minVolume
|
protected |
minVolume variable
Definition at line 149 of file MeshChecker.hpp.
◆ m_minVolumeChange
|
protected |
minVolumeChange variable
Definition at line 155 of file MeshChecker.hpp.
◆ m_minVolumeChangeTol
|
protected |
check tolerance for minVolumeChange variable
Definition at line 163 of file MeshChecker.hpp.
◆ m_minVolumeTol
|
protected |
check tolerance for minVolume variable
Definition at line 158 of file MeshChecker.hpp.
◆ m_printResumeFile
|
protected |
true, print a resume file after checking
Definition at line 178 of file MeshChecker.hpp.
◆ m_qualityStatus
|
protected |
Quality check flag of the mesh related to tolerance exceeded (first error encountered with this order): -1, not computed; 0, mesh good with input tolerances; 1, minimum face validity too low; 2, volume change error; 3, skewness on boundary error; 4, skewness error; 5, minimum volume error; 6, max volume error.
Definition at line 166 of file MeshChecker.hpp.
◆ m_skewness
|
protected |
INTERNAL USE Cells with poor skewness.
Definition at line 174 of file MeshChecker.hpp.
◆ m_volume
|
protected |
INTERNAL USE Cells with poor volume.
Definition at line 173 of file MeshChecker.hpp.
◆ m_volumechange
|
protected |
INTERNAL USE Cells with poor volume change ratio.
Definition at line 176 of file MeshChecker.hpp.
◆ m_volumes
|
protected |
Cell volumes.
Definition at line 171 of file MeshChecker.hpp.
The documentation for this class was generated from the following files:
- src/utils/MeshChecker.hpp
- src/utils/MeshChecker.cpp
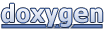