FVGenericSelection class specialized for selections with volume cylindrical primitive shapes. More...
#include <FVMeshSelection.hpp>
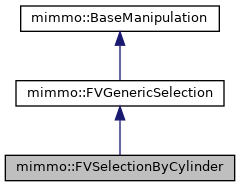

Protected Member Functions | |
void | buildPorts () |
void | swap (FVSelectionByCylinder &) noexcept |
![]() | |
bool | checkCoherenceBulkBoundary () |
void | cleanUpBoundaryPatch () |
MimmoSharedPointer< MimmoObject > | createInternalBoundaryPatch () |
virtual void | plotOptionalResults () |
void | swap (FVGenericSelection &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Detailed Description
FVGenericSelection class specialized for selections with volume cylindrical primitive shapes.
Use SelectionByCylinder internally to extract bulk+boundary sub-patches.
Ports available in FVSelectionByCylinder Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_AXES | setRefSystem | (MC_ARR3ARR3, MD_FLOAT) |
M_SPAN | setSpan | (MC_ARRAY3, MD_FLOAT) |
M_INFLIMITS | setInfLimits | (MC_ARRAY3, MD_FLOAT) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
Inherited from FVGenericSelection
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_VALUEB | setDual | (MC_SCALAR, MD_BOOL) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
M_GEOM2 | setBoundary | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getVolumePatch | (MC_SCALAR, MD_MIMMO_) |
M_GEOM2 | getBoundaryPatch | (MC_SCALAR, MD_MIMMO_) |
M_GEOM3 | getInternalBoundaryPatch | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.FVSelectionByCylinder
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing.
Proper of the class:
- Topology : 1 for volume mesh+surface boundary, 2 for surface mesh + 3Dcurve boundary. No other value allowed.
- Dual: boolean to get straight what given by selection method or its exact dual;
- Origin: array of 3 doubles identifying origin of cylinder (space separated);
- Span: span of the cylinder (base_radius angular_azimuthal_width height);
- RefSystem: reference system of the cylinder
<RefSystem>
<axis0> 1.0 0.0 0.0 </axis0>
<axis1> 0.0 1.0 0.0 </axis1>
<axis2> 0.0 0.0 1.0 </axis2>
</RefSystem>
- InfLimits: set starting point for each cylindrical coordinate. Useful to assign different starting angular coordinate to azimuthal width.
Geometry has to be mandatorily passed through port.
Definition at line 286 of file FVMeshSelection.hpp.
Constructor & Destructor Documentation
◆ FVSelectionByCylinder() [1/4]
mimmo::FVSelectionByCylinder::FVSelectionByCylinder | ( | int | topo = 1 | ) |
Basic Constructor. Parameter topo get topology of the target MimmoFvMesh where performing extraction:
- 1 for volume bulk and surface boundary
- 2 for surface bulk and 3DCurve boundary
No other values are allowed
- Parameters
-
[in] topo topology of the target MimmoFvMesh.
Definition at line 38 of file FVSelectionByCylinder.cpp.
◆ FVSelectionByCylinder() [2/4]
mimmo::FVSelectionByCylinder::FVSelectionByCylinder | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 47 of file FVSelectionByCylinder.cpp.
◆ FVSelectionByCylinder() [3/4]
mimmo::FVSelectionByCylinder::FVSelectionByCylinder | ( | int | topo, |
darray3E | origin, | ||
darray3E | span, | ||
double | infLimTheta, | ||
darray3E | mainAxis | ||
) |
Custom Constructor. Pay attention span of angular coordinate must be at most 2*pi.
- Parameters
-
[in] topo topology of the target MimmoFvMesh.See Basic Constructor doxy. [in] origin Origin of the cylinder->baricenter [in] span Span of the cylinder, basis radius/ span of angular coord in radians/height [in] infLimTheta Starting origin of the angular coordinate. default is 0 radians. [in] mainAxis Orientation of the cylinder height axis
Definition at line 80 of file FVSelectionByCylinder.cpp.
◆ ~FVSelectionByCylinder()
|
virtual |
Destructor
Definition at line 96 of file FVSelectionByCylinder.cpp.
◆ FVSelectionByCylinder() [4/4]
mimmo::FVSelectionByCylinder::FVSelectionByCylinder | ( | const FVSelectionByCylinder & | other | ) |
Copy Constructor
Definition at line 101 of file FVSelectionByCylinder.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 179 of file FVSelectionByCylinder.cpp.
◆ buildPorts()
|
protectedvirtual |
It builds the input/output ports of the object
Reimplemented from mimmo::FVGenericSelection.
Definition at line 125 of file FVSelectionByCylinder.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 294 of file FVSelectionByCylinder.cpp.
◆ operator=()
FVSelectionByCylinder & mimmo::FVSelectionByCylinder::operator= | ( | FVSelectionByCylinder | other | ) |
Copy operator
Definition at line 106 of file FVSelectionByCylinder.cpp.
◆ setInfLimits()
void mimmo::FVSelectionByCylinder::setInfLimits | ( | darray3E | val | ) |
Set inferior limits of cylinder, useful to alter starting angular coordinate (0,2*pi) of cylinder (pos 1 in the array,for example {{0, pi/3, 0}}).
- Parameters
-
[in] val lower value origin for all three coordinates
Definition at line 170 of file FVSelectionByCylinder.cpp.
◆ setOrigin()
void mimmo::FVSelectionByCylinder::setOrigin | ( | darray3E | origin | ) |
Set origin of the cylinder. The origin is meant as the cylinder baricenter.
- Parameters
-
[in] origin new origin point
Definition at line 143 of file FVSelectionByCylinder.cpp.
◆ setRefSystem()
void mimmo::FVSelectionByCylinder::setRefSystem | ( | dmatrix33E | axes | ) |
Set new axis orientation of the local reference system
- Parameters
-
[in] axes
Definition at line 161 of file FVSelectionByCylinder.cpp.
◆ setSpan()
void mimmo::FVSelectionByCylinder::setSpan | ( | darray3E | span | ) |
Set span of the cylinder according to its local reference system of axes Angular span (1 position) is expressed in radians
- Parameters
-
[in] span
Definition at line 152 of file FVSelectionByCylinder.cpp.
◆ swap()
|
protectednoexcept |
Swap function. Assign data of this class to another of the same type and vice-versa. Resulting patch of selection is not swapped, ever.
- Parameters
-
[in] x FVSelectionByCylinder object
Definition at line 116 of file FVSelectionByCylinder.cpp.
The documentation for this class was generated from the following files:
- src/geohandlers/FVMeshSelection.hpp
- src/geohandlers/FVSelectionByCylinder.cpp
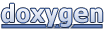