FVSelectionByCylinder.cpp
101 FVSelectionByCylinder::FVSelectionByCylinder(const FVSelectionByCylinder & other):FVGenericSelection(other){};
130 built = (built && createPortIn<darray3E, FVSelectionByCylinder>(this, &FVSelectionByCylinder::setOrigin,M_POINT));
131 built = (built && createPortIn<darray3E, FVSelectionByCylinder>(this, &FVSelectionByCylinder::setSpan, M_SPAN));
132 built = (built && createPortIn<dmatrix33E, FVSelectionByCylinder>(this, &FVSelectionByCylinder::setRefSystem, M_AXES));
133 built = (built && createPortIn<darray3E, FVSelectionByCylinder>(this, &FVSelectionByCylinder::setInfLimits, M_INFLIMITS));
179 FVSelectionByCylinder::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
void swap(FVSelectionByCylinder &) noexcept
Definition: FVSelectionByCylinder.cpp:116
void setInfLimits(double val, int dir)
Definition: BasicShapes.cpp:163
FVGenericSelection class specialized for selections with volume cylindrical primitive shapes.
Definition: FVMeshSelection.hpp:286
void setDual(bool flag=false)
Definition: FVGenericSelection.cpp:137
FVSelectionByCylinder(int topo=1)
Definition: FVSelectionByCylinder.cpp:38
Interface for applying selection methods simultaneously on bulk+boundary compound meshes.
Definition: FVMeshSelection.hpp:78
void setOrigin(darray3E origin)
Definition: FVSelectionByCylinder.cpp:143
void setInfLimits(darray3E val)
Definition: FVSelectionByCylinder.cpp:170
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicShapes.cpp:196
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
MimmoSharedPointer< GenericSelection > m_selectEngine
Definition: FVMeshSelection.hpp:114
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByCylinder.cpp:294
FVSelectionByCylinder & operator=(FVSelectionByCylinder other)
Definition: FVSelectionByCylinder.cpp:106
virtual ~FVSelectionByCylinder()
Definition: FVSelectionByCylinder.cpp:96
void setSpan(darray3E span)
Definition: FVSelectionByCylinder.cpp:152
void setRefSystem(dmatrix33E axes)
Definition: FVSelectionByCylinder.cpp:161
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByCylinder.cpp:179
void swap(FVGenericSelection &x) noexcept
Definition: FVGenericSelection.cpp:73
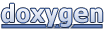