FVGenericSelection.cpp
53 FVGenericSelection::FVGenericSelection(const FVGenericSelection & other):BaseManipulation(other){
90 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, FVGenericSelection>(this, &FVGenericSelection::setGeometry, M_GEOM, true));
91 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, FVGenericSelection>(this, &FVGenericSelection::setBoundaryGeometry, M_GEOM2, true));
92 built = (built && createPortIn<bool, FVGenericSelection>(this, &FVGenericSelection::setDual,M_VALUEB));
94 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, FVGenericSelection>(this, &FVGenericSelection::getVolumePatch, M_GEOM));
95 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, FVGenericSelection>(this, &FVGenericSelection::getBoundaryPatch, M_GEOM2));
96 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, FVGenericSelection>(this, &FVGenericSelection::getInternalBoundaryPatch, M_GEOM3));
227 throw std::runtime_error (m_name + " : nullptr pointer to one or both bulk/boundary targets found");
237 (*m_log)<<"Warning in "+m_name + " : id-vertex uncoherent bulk/boundary geometry linked"<<std::endl;
279 livector1D temp = m_bndpatch->getCellFromVertexList(boundaryClearedVerts, true); // true strictly cell defined by this set.
339 livector1D sharedCells = m_patch->getCellFromVertexList(realBoundaryVertices, true); //strict=true means cells defined by this vertices list.
void cleanUpBoundaryPatch()
Definition: FVGenericSelection.cpp:265
void setDual(bool flag=false)
Definition: FVGenericSelection.cpp:137
MimmoSharedPointer< MimmoObject > m_intbndpatch
Definition: FVMeshSelection.hpp:117
MimmoSharedPointer< MimmoObject > m_bndgeometry
Definition: FVMeshSelection.hpp:113
MimmoSharedPointer< MimmoObject > createInternalBoundaryPatch()
Definition: FVGenericSelection.cpp:323
Interface for applying selection methods simultaneously on bulk+boundary compound meshes.
Definition: FVMeshSelection.hpp:78
FVGenericSelection(int topo=1)
Definition: FVGenericSelection.cpp:38
FVGenericSelection & operator=(FVGenericSelection other)
Definition: FVGenericSelection.cpp:63
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setBoundaryGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: FVGenericSelection.cpp:120
MimmoSharedPointer< MimmoObject > m_bndpatch
Definition: FVMeshSelection.hpp:116
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
const mimmo::MimmoSharedPointer< MimmoObject > getBoundaryPatch() const
Definition: FVGenericSelection.cpp:166
MimmoSharedPointer< GenericSelection > m_selectEngine
Definition: FVMeshSelection.hpp:114
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
@ NONE
MimmoSharedPointer< MimmoObject > m_volpatch
Definition: FVMeshSelection.hpp:115
void setSelection(MimmoSharedPointer< GenericSelection > selectBlock)
Definition: FVGenericSelection.cpp:146
virtual ~FVGenericSelection()
Definition: FVGenericSelection.cpp:48
const mimmo::MimmoSharedPointer< MimmoObject > getInternalBoundaryPatch() const
Definition: FVGenericSelection.cpp:176
bool checkCoherenceBulkBoundary()
Definition: FVGenericSelection.cpp:386
const mimmo::MimmoSharedPointer< MimmoObject > getVolumePatch() const
Definition: FVGenericSelection.cpp:157
virtual void plotOptionalResults()
Definition: FVGenericSelection.cpp:363
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: FVGenericSelection.cpp:107
void swap(FVGenericSelection &x) noexcept
Definition: FVGenericSelection.cpp:73
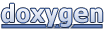