FVMeshSelection.hpp
214 void setSelection(MimmoSharedPointer<GenericSelection> selectBlock){BITPIT_UNUSED(selectBlock);};
291 FVSelectionByCylinder(int topo, darray3E origin, darray3E span, double infLimTheta, darray3E mainAxis);
309 void setSelection(MimmoSharedPointer<GenericSelection> selectBlock){BITPIT_UNUSED(selectBlock);};
391 FVSelectionBySphere(int topo, darray3E origin, darray3E span, double infLimTheta, double infLimPhi);
409 void setSelection(MimmoSharedPointer<GenericSelection> selectBlock){BITPIT_UNUSED(selectBlock);};
void swap(FVSelectionByCylinder &) noexcept
Definition: FVSelectionByCylinder.cpp:116
FVGenericSelection class specialized for selections with volume spherical primitive shapes.
Definition: FVMeshSelection.hpp:386
void swap(FVSelectionBySphere &) noexcept
Definition: FVSelectionBySphere.cpp:115
void cleanUpBoundaryPatch()
Definition: FVGenericSelection.cpp:265
FVGenericSelection class specialized for selections with volume cylindrical primitive shapes.
Definition: FVMeshSelection.hpp:286
FVSelectionByBox(int topo=1)
Definition: FVSelectionByBox.cpp:38
void setDual(bool flag=false)
Definition: FVGenericSelection.cpp:137
FVSelectionBySphere & operator=(FVSelectionBySphere other)
Definition: FVSelectionBySphere.cpp:105
MimmoSharedPointer< MimmoObject > m_intbndpatch
Definition: FVMeshSelection.hpp:117
MimmoSharedPointer< MimmoObject > m_bndgeometry
Definition: FVMeshSelection.hpp:113
virtual ~FVSelectionBySphere()
Definition: FVSelectionBySphere.cpp:95
MimmoSharedPointer< MimmoObject > createInternalBoundaryPatch()
Definition: FVGenericSelection.cpp:323
FVSelectionByCylinder(int topo=1)
Definition: FVSelectionByCylinder.cpp:38
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionBySphere.cpp:180
void setOrigin(darray3E origin)
Definition: FVSelectionByBox.cpp:136
Interface for applying selection methods simultaneously on bulk+boundary compound meshes.
Definition: FVMeshSelection.hpp:78
FVGenericSelection(int topo=1)
Definition: FVGenericSelection.cpp:38
FVGenericSelection & operator=(FVGenericSelection other)
Definition: FVGenericSelection.cpp:63
void setOrigin(darray3E origin)
Definition: FVSelectionByCylinder.cpp:143
void setInfLimits(darray3E val)
Definition: FVSelectionByCylinder.cpp:170
void setInfLimits(darray3E val)
Definition: FVSelectionBySphere.cpp:169
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByBox.cpp:265
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setSpan(darray3E span)
Definition: FVSelectionBySphere.cpp:151
FVGenericSelection class specialized for selections with volume box primitive shapes.
Definition: FVMeshSelection.hpp:192
void setBoundaryGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: FVGenericSelection.cpp:120
void swap(FVSelectionByBox &) noexcept
Definition: FVSelectionByBox.cpp:110
MimmoSharedPointer< MimmoObject > m_bndpatch
Definition: FVMeshSelection.hpp:116
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
const mimmo::MimmoSharedPointer< MimmoObject > getBoundaryPatch() const
Definition: FVGenericSelection.cpp:166
void setOrigin(darray3E origin)
Definition: FVSelectionBySphere.cpp:142
MimmoSharedPointer< GenericSelection > m_selectEngine
Definition: FVMeshSelection.hpp:114
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByCylinder.cpp:294
FVSelectionByCylinder & operator=(FVSelectionByCylinder other)
Definition: FVSelectionByCylinder.cpp:106
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByBox.cpp:163
virtual ~FVSelectionByBox()
Definition: FVSelectionByBox.cpp:90
FVSelectionByBox & operator=(FVSelectionByBox other)
Definition: FVSelectionByBox.cpp:100
MimmoSharedPointer< MimmoObject > m_volpatch
Definition: FVMeshSelection.hpp:115
void setSelection(MimmoSharedPointer< GenericSelection > selectBlock)
Definition: FVGenericSelection.cpp:146
virtual ~FVGenericSelection()
Definition: FVGenericSelection.cpp:48
const mimmo::MimmoSharedPointer< MimmoObject > getInternalBoundaryPatch() const
Definition: FVGenericSelection.cpp:176
bool checkCoherenceBulkBoundary()
Definition: FVGenericSelection.cpp:386
const mimmo::MimmoSharedPointer< MimmoObject > getVolumePatch() const
Definition: FVGenericSelection.cpp:157
virtual void plotOptionalResults()
Definition: FVGenericSelection.cpp:363
virtual ~FVSelectionByCylinder()
Definition: FVSelectionByCylinder.cpp:96
void setSpan(darray3E span)
Definition: FVSelectionByCylinder.cpp:152
void setRefSystem(dmatrix33E axes)
Definition: FVSelectionBySphere.cpp:160
void setRefSystem(dmatrix33E axes)
Definition: FVSelectionByCylinder.cpp:161
void setRefSystem(dmatrix33E axes)
Definition: FVSelectionByBox.cpp:153
void setGeometry(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: FVGenericSelection.cpp:107
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByCylinder.cpp:179
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionBySphere.cpp:295
FVSelectionBySphere(int topo=1)
Definition: FVSelectionBySphere.cpp:38
void swap(FVGenericSelection &x) noexcept
Definition: FVGenericSelection.cpp:73
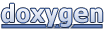