FVSelectionByBox.cpp
77 FVSelectionByBox::FVSelectionByBox(int topo, darray3E origin, darray3E span): FVGenericSelection(topo){
124 built = (built && createPortIn<darray3E, FVSelectionByBox>(this, &FVSelectionByBox::setOrigin, M_POINT ));
125 built = (built && createPortIn<darray3E, FVSelectionByBox>(this, &FVSelectionByBox::setSpan, M_SPAN));
126 built = (built && createPortIn<dmatrix33E, FVSelectionByBox>(this, &FVSelectionByBox::setRefSystem, M_AXES));
FVSelectionByBox(int topo=1)
Definition: FVSelectionByBox.cpp:38
void setDual(bool flag=false)
Definition: FVGenericSelection.cpp:137
void setOrigin(darray3E origin)
Definition: FVSelectionByBox.cpp:136
Interface for applying selection methods simultaneously on bulk+boundary compound meshes.
Definition: FVMeshSelection.hpp:78
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByBox.cpp:265
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
FVGenericSelection class specialized for selections with volume box primitive shapes.
Definition: FVMeshSelection.hpp:192
void swap(FVSelectionByBox &) noexcept
Definition: FVSelectionByBox.cpp:110
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
MimmoSharedPointer< GenericSelection > m_selectEngine
Definition: FVMeshSelection.hpp:114
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionByBox.cpp:163
virtual ~FVSelectionByBox()
Definition: FVSelectionByBox.cpp:90
FVSelectionByBox & operator=(FVSelectionByBox other)
Definition: FVSelectionByBox.cpp:100
void setRefSystem(dmatrix33E axes)
Definition: FVSelectionByBox.cpp:153
void swap(FVGenericSelection &x) noexcept
Definition: FVGenericSelection.cpp:73
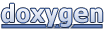