FVSelectionBySphere.cpp
80 FVSelectionBySphere::FVSelectionBySphere(int topo, darray3E origin, darray3E span, double infLimTheta, double infLimPhi):
100 FVSelectionBySphere::FVSelectionBySphere(const FVSelectionBySphere & other):FVGenericSelection(other){};
130 built = (built && createPortIn<darray3E, FVSelectionBySphere>(this, &FVSelectionBySphere::setOrigin,M_POINT));
131 built = (built && createPortIn<darray3E, FVSelectionBySphere>(this, &FVSelectionBySphere::setSpan, M_SPAN));
132 built = (built && createPortIn<dmatrix33E, FVSelectionBySphere>(this, &FVSelectionBySphere::setRefSystem, M_AXES));
133 built = (built && createPortIn<darray3E, FVSelectionBySphere>(this, &FVSelectionBySphere::setInfLimits, M_INFLIMITS));
180 FVSelectionBySphere::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
void setInfLimits(double val, int dir)
Definition: BasicShapes.cpp:163
FVGenericSelection class specialized for selections with volume spherical primitive shapes.
Definition: FVMeshSelection.hpp:386
void swap(FVSelectionBySphere &) noexcept
Definition: FVSelectionBySphere.cpp:115
void setDual(bool flag=false)
Definition: FVGenericSelection.cpp:137
FVSelectionBySphere & operator=(FVSelectionBySphere other)
Definition: FVSelectionBySphere.cpp:105
virtual ~FVSelectionBySphere()
Definition: FVSelectionBySphere.cpp:95
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionBySphere.cpp:180
Interface for applying selection methods simultaneously on bulk+boundary compound meshes.
Definition: FVMeshSelection.hpp:78
void setInfLimits(darray3E val)
Definition: FVSelectionBySphere.cpp:169
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
void setSpan(darray3E span)
Definition: FVSelectionBySphere.cpp:151
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
void setOrigin(darray3E origin)
Definition: FVSelectionBySphere.cpp:142
MimmoSharedPointer< GenericSelection > m_selectEngine
Definition: FVMeshSelection.hpp:114
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void setRefSystem(dmatrix33E axes)
Definition: FVSelectionBySphere.cpp:160
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: FVSelectionBySphere.cpp:295
FVSelectionBySphere(int topo=1)
Definition: FVSelectionBySphere.cpp:38
void swap(FVGenericSelection &x) noexcept
Definition: FVGenericSelection.cpp:73
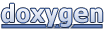