Radial Basis Functions Bounding Box calculator. More...
#include <RBFBox.hpp>
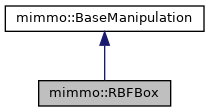
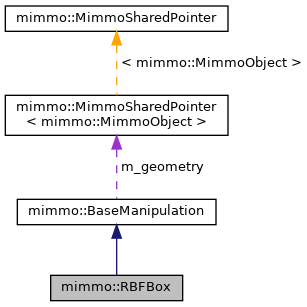
Public Member Functions | |
RBFBox () | |
RBFBox (const bitpit::Config::Section &rootXML) | |
RBFBox (const RBFBox &other) | |
virtual | ~RBFBox () |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | clearRBFBox () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
void | getAABB (darray3E &bMin, darray3E &bMax) |
darray3E | getOrigin () |
darray3E | getSpan () |
void | plot (std::string directory, std::string filename, int counter, bool binary) |
void | setGeometry (MimmoSharedPointer< MimmoObject > cloud) |
void | setSupportRadius (double suppR_) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
virtual void | plotOptionalResults () |
void | swap (RBFBox &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
darray3E | m_origin |
darray3E | m_span |
double | m_suppR |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Radial Basis Functions Bounding Box calculator.
Builds the Axes Aligned Bounding Box around a set of RBF points, described as spheres of support radius R.
Ports available in RBFBox Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
M_VALUED | setSupportRadius | (MC_SCALAR, MD_FLOAT) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_POINT | getOrigin | (MC_ARRAY3, MD_FLOAT) |
M_SPAN | getSpan | (MC_ARRAY3, MD_FLOAT) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.RBFBox
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing.
Proper of the class:
- SupportRadius: Influence Radius value for RBF cloud in input;
Nodes list has to be mandatorily passed through port.
- Examples
- utils_example_00003.cpp.
Definition at line 74 of file RBFBox.hpp.
Constructor & Destructor Documentation
◆ RBFBox() [1/3]
mimmo::RBFBox::RBFBox | ( | ) |
Basic Constructor.
Definition at line 31 of file RBFBox.cpp.
◆ RBFBox() [2/3]
mimmo::RBFBox::RBFBox | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 42 of file RBFBox.cpp.
◆ ~RBFBox()
|
virtual |
Destructor
Definition at line 61 of file RBFBox.cpp.
◆ RBFBox() [3/3]
mimmo::RBFBox::RBFBox | ( | const RBFBox & | other | ) |
Copy Constructor
- Parameters
-
[in] other RBFBox where copy from
Definition at line 66 of file RBFBox.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 256 of file RBFBox.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 87 of file RBFBox.cpp.
◆ clearRBFBox()
void mimmo::RBFBox::clearRBFBox | ( | ) |
Clean all stuffs in your class
Definition at line 102 of file RBFBox.cpp.
◆ execute()
|
virtual |
Execute your object. It calculates the RBFBox of the input set of RBFs (+1% of support radius).
Implements mimmo::BaseManipulation.
Definition at line 209 of file RBFBox.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 280 of file RBFBox.cpp.
◆ getAABB()
Return the min and max points of the RBFBox.
- Parameters
-
[out] bMin min AABB point [out] bMax max AABB point
Definition at line 133 of file RBFBox.cpp.
◆ getOrigin()
darray3E mimmo::RBFBox::getOrigin | ( | ) |
Return the origin of the RBFBox.
- Returns
- origin of the box
Definition at line 115 of file RBFBox.cpp.
◆ getSpan()
darray3E mimmo::RBFBox::getSpan | ( | ) |
◆ plot()
void mimmo::RBFBox::plot | ( | std::string | directory, |
std::string | filename, | ||
int | counter, | ||
bool | binary | ||
) |
Plot the AABB as a structured grid to *vtu file.
- Parameters
-
[in] directory output directory [in] filename output filename w/out tag [in] counter integer identifier of the file [in] binary boolean flag for 0-"ascii" or 1-"appended" writing
Definition at line 165 of file RBFBox.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
Plot Optional results of the class, that is the oriented bounding box as *.vtu mesh
Reimplemented from mimmo::BaseManipulation.
Definition at line 246 of file RBFBox.cpp.
◆ setGeometry()
void mimmo::RBFBox::setGeometry | ( | MimmoSharedPointer< MimmoObject > | cloud | ) |
Set a Point Cloud MimmoObject as reference target rbfs.
- Parameters
-
[in] cloud set of rbf nodes.
Definition at line 142 of file RBFBox.cpp.
◆ setSupportRadius()
void mimmo::RBFBox::setSupportRadius | ( | double | suppR_ | ) |
Set the value of the support radius R of RBF kernel functions.
- Parameters
-
[in] suppR_ new value of support radius.
- Examples
- utils_example_00003.cpp.
Definition at line 153 of file RBFBox.cpp.
◆ swap()
|
protectednoexcept |
Member Data Documentation
◆ m_origin
|
protected |
Origin of the BB.
Definition at line 77 of file RBFBox.hpp.
◆ m_span
|
protected |
Span of the BB.
Definition at line 78 of file RBFBox.hpp.
◆ m_suppR
|
protected |
Support radius value of RBFs.
Definition at line 79 of file RBFBox.hpp.
The documentation for this class was generated from the following files:
- src/utils/RBFBox.hpp
- src/utils/RBFBox.cpp
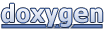