RBFBox.cpp
91 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, RBFBox>(this, &mimmo::RBFBox::setGeometry, M_GEOM, true));
92 built = (built && createPortIn<double, RBFBox>(this, &mimmo::RBFBox::setSupportRadius, M_VALUED));
void setGeometry(MimmoSharedPointer< MimmoObject > cloud)
Definition: RBFBox.cpp:142
void plot(std::string directory, std::string filename, int counter, bool binary)
Definition: RBFBox.cpp:165
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: RBFBox.cpp:280
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: RBFBox.cpp:256
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
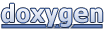