RBFBox.hpp
void setGeometry(MimmoSharedPointer< MimmoObject > cloud)
Definition: RBFBox.cpp:142
void plot(std::string directory, std::string filename, int counter, bool binary)
Definition: RBFBox.cpp:165
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: RBFBox.cpp:280
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: RBFBox.cpp:256
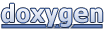