RefineGeometry is an executable block class capable of refine a surface geometry. More...
#include <RefineGeometry.hpp>
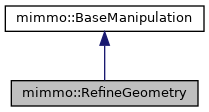
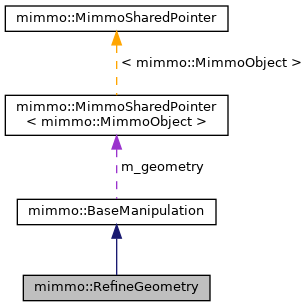
Public Member Functions | |
RefineGeometry () | |
RefineGeometry (const bitpit::Config::Section &rootXML) | |
RefineGeometry (const RefineGeometry &other) | |
virtual | ~RefineGeometry () |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | clear () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
RefineType | getRefineType () |
RefineGeometry & | operator= (RefineGeometry other) |
void | plotOptionalResults () |
void | setRefineSteps (int steps) |
void | setRefineType (int type) |
void | setRefineType (RefineType type) |
void | setSmoothingSteps (int steps) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
bool | checkTriangulation () |
std::vector< long > | greenRefineCell (const long &cellId, const long newVertexId, int iface) |
void | redgreenRefine (std::unordered_map< long, long > *mapping=nullptr, mimmo::MimmoSharedPointer< MimmoObject > coarsepatch=nullptr, mimmo::MimmoSharedPointer< MimmoObject > refinepatch=nullptr) |
std::vector< long > | redRefineCell (const long &cellId, const std::vector< long > &newVertexIds) |
void | smoothing (std::set< long > *constrainedVertices=nullptr) |
void | swap (RefineGeometry &x) noexcept |
void | ternaryRefine (std::unordered_map< long, long > *mapping=nullptr, mimmo::MimmoSharedPointer< MimmoObject > coarsepatch=nullptr, mimmo::MimmoSharedPointer< MimmoObject > refinepatch=nullptr) |
std::vector< long > | ternaryRefineCell (const long &cellId, const std::vector< bitpit::Vertex > &vertices, const std::array< double, 3 > ¢er) |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
std::vector< long > | m_activecells |
int | m_refinements |
int | m_steps |
RefineType | m_type |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
RefineGeometry is an executable block class capable of refine a surface geometry.
RefineGeometry is the object to refine a surface MimmoObject. After the execution of the block the geometry will be refined in function of the refinement method chosen. Beware, the original geometry is permanently modified after refinement.
At the end of the refinement a laplacian smoothing regularization can be performed. Two sub-steps are carried out, a positive and a negative smoothing step, that minimizes the effect on the sharp edges of the geometry. The smoothing is activated by imposing a number of smoothing steps > 0 (default = 0).
Ports available in RefineGeometry Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.RefineGeometry
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class.
- OutputPlot: target directory for optional results writing.
Proper of the class:
- RefineType: refine method-> 0 Ternary , 1 Red-Green
- RefineSteps: (uint) number of refinements. 0 means no refinement.
- SmoothingSteps: (uint) number of smoothing steps. 0 means no smoothing.
Geometries have to be mandatorily passed through port.
- Examples
- geohandlers_example_00001.cpp.
Definition at line 92 of file RefineGeometry.hpp.
Constructor & Destructor Documentation
◆ RefineGeometry() [1/3]
mimmo::RefineGeometry::RefineGeometry | ( | ) |
Default constructor of RefineGeometry.
Definition at line 35 of file RefineGeometry.cpp.
◆ RefineGeometry() [2/3]
mimmo::RefineGeometry::RefineGeometry | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 46 of file RefineGeometry.cpp.
◆ ~RefineGeometry()
|
virtual |
Default destructor of RefineGeometry.
Definition at line 67 of file RefineGeometry.cpp.
◆ RefineGeometry() [3/3]
mimmo::RefineGeometry::RefineGeometry | ( | const RefineGeometry & | other | ) |
Copy constructor of RefineGeometry.
Definition at line 72 of file RefineGeometry.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 218 of file RefineGeometry.cpp.
◆ buildPorts()
|
virtual |
Building ports of the class
Implements mimmo::BaseManipulation.
Definition at line 104 of file RefineGeometry.cpp.
◆ checkTriangulation()
|
protected |
Check if the target geometry of the class is a regular triangulation
- Returns
- true if a regular triangulation.
Definition at line 1171 of file RefineGeometry.cpp.
◆ clear()
void mimmo::RefineGeometry::clear | ( | ) |
Clear all stuffs in your class
Definition at line 168 of file RefineGeometry.cpp.
◆ execute()
|
virtual |
Execution command. It refines the target surface geometry.
Implements mimmo::BaseManipulation.
Definition at line 177 of file RefineGeometry.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 266 of file RefineGeometry.cpp.
◆ getRefineType()
RefineType mimmo::RefineGeometry::getRefineType | ( | ) |
Return kind of refinement set for the object.
- Returns
- refine type
Definition at line 116 of file RefineGeometry.cpp.
◆ greenRefineCell()
|
protected |
It refines the target cell with green method.
- Parameters
-
[in] cellId Id of the target cell [in] newVertexId Id of the new vertex (already in the mesh) to be used to refine the cell [in] splitEdgeIndex Index of the edge to split
- Returns
- Ids of the new created cells
Definition at line 908 of file RefineGeometry.cpp.
◆ operator=()
RefineGeometry & mimmo::RefineGeometry::operator= | ( | RefineGeometry | other | ) |
Assignement operator of RefineGeometry. Soft copy of MimmoObject
Definition at line 81 of file RefineGeometry.cpp.
◆ plotOptionalResults()
|
virtual |
Plot stitched geometry in *.vtu file as optional result;
Reimplemented from mimmo::BaseManipulation.
Definition at line 279 of file RefineGeometry.cpp.
◆ redgreenRefine()
|
protected |
Refinement by redgreen method. The starting elements must be all triangles. All the triangle edges are splitted for every cell in case of red refinement (bulk cells), only one edge is splitted in case of green refinement (boundary cells).
- Parameters
-
[out] mapping pointer to mapping container (new child cell id -> parent cell id) [out] coarsepatch pointer to geometry containing the original parent cells [out] refinepatch pointer to geometry containing the new children refined cells
Definition at line 461 of file RefineGeometry.cpp.
◆ redRefineCell()
|
protected |
It refines the target cell with red method.
- Parameters
-
[in] cellId Id of the target cell [in] newVertexIds Ids of the new vertices (already in the mesh) to be used to refine the cell
- Returns
- Ids of the new created cells
Definition at line 850 of file RefineGeometry.cpp.
◆ setRefineSteps()
void mimmo::RefineGeometry::setRefineSteps | ( | int | steps | ) |
It sets the number of refinement steps
- Parameters
-
[in] steps refinement steps
- Examples
- geohandlers_example_00001.cpp.
Definition at line 150 of file RefineGeometry.cpp.
◆ setRefineType() [1/2]
void mimmo::RefineGeometry::setRefineType | ( | int | type | ) |
It sets refinement method of refine block
- Parameters
-
[in] type refine type
Definition at line 137 of file RefineGeometry.cpp.
◆ setRefineType() [2/2]
void mimmo::RefineGeometry::setRefineType | ( | RefineType | type | ) |
It sets refinement method of refine block
- Parameters
-
[in] type refine type
- Examples
- geohandlers_example_00001.cpp.
Definition at line 126 of file RefineGeometry.cpp.
◆ setSmoothingSteps()
void mimmo::RefineGeometry::setSmoothingSteps | ( | int | steps | ) |
It sets the number of positive/negative laplacian smoothing steps
- Parameters
-
[in] steps smoothing steps
- Examples
- geohandlers_example_00001.cpp.
Definition at line 160 of file RefineGeometry.cpp.
◆ smoothing()
|
protected |
Perform the laplacian smoothing for the surface patch. Each step is divided in two sub-steps, a laplacian smoothing and a laplacian anti-smoothing with negative coefficient.
- Parameters
-
[in] constrainedVertices Pointer to set of constrined vertices indices
Definition at line 959 of file RefineGeometry.cpp.
◆ swap()
|
protectednoexcept |
Swap function of RefineGeometry.
- Parameters
-
[in] x object to be swapped.
Definition at line 91 of file RefineGeometry.cpp.
◆ ternaryRefine()
|
protected |
Global refinement by ternary method. A vertex is added on the barycenter of each cell. One triangle for each edge is added for every cell.
Definition at line 288 of file RefineGeometry.cpp.
◆ ternaryRefineCell()
|
protected |
It refines the target cell with perimeter defined by vertices and center point.
- Parameters
-
[in] cellId cell id of the cell to be refined [in] vertices perimeter vertices of the cell [in] center center point of the cell
- Returns
- Cell ids of the new refined cells insert in the geometry
Note: the old refined cell is not deleted from the geometry in this function.
Definition at line 412 of file RefineGeometry.cpp.
Member Data Documentation
◆ m_activecells
|
protected |
Cells Id candidate to be refined.
Definition at line 99 of file RefineGeometry.hpp.
◆ m_refinements
|
protected |
Number of refinements. Default 1
Definition at line 96 of file RefineGeometry.hpp.
◆ m_steps
|
protected |
Smoothing steps after the refinement.
Definition at line 97 of file RefineGeometry.hpp.
◆ m_type
|
protected |
Refine mode. Default 1 - RedGreen
Definition at line 95 of file RefineGeometry.hpp.
The documentation for this class was generated from the following files:
- src/geohandlers/RefineGeometry.hpp
- src/geohandlers/RefineGeometry.cpp
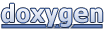