RefineGeometry.hpp
127 void ternaryRefine(std::unordered_map<long,long> * mapping = nullptr, mimmo::MimmoSharedPointer<MimmoObject> coarsepatch = nullptr, mimmo::MimmoSharedPointer<MimmoObject> refinepatch = nullptr);
128 std::vector<long> ternaryRefineCell(const long & cellId, const std::vector<bitpit::Vertex> & vertices, const std::array<double,3> & center);
129 void redgreenRefine(std::unordered_map<long,long> * mapping = nullptr, mimmo::MimmoSharedPointer<MimmoObject> coarsepatch = nullptr, mimmo::MimmoSharedPointer<MimmoObject> refinepatch = nullptr);
@ REDGREEN
std::vector< long > ternaryRefineCell(const long &cellId, const std::vector< bitpit::Vertex > &vertices, const std::array< double, 3 > ¢er)
Definition: RefineGeometry.cpp:412
void setSmoothingSteps(int steps)
Definition: RefineGeometry.cpp:160
void plotOptionalResults()
Definition: RefineGeometry.cpp:279
RefineType
Methods available for refining globally a (surface) geometry.
Definition: RefineGeometry.hpp:35
@ TERNARY
bool checkTriangulation()
Definition: RefineGeometry.cpp:1171
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: RefineGeometry.cpp:266
std::vector< long > m_activecells
Definition: RefineGeometry.hpp:99
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: RefineGeometry.cpp:218
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
void setRefineType(RefineType type)
Definition: RefineGeometry.cpp:126
void setRefineSteps(int steps)
Definition: RefineGeometry.cpp:150
std::vector< long > redRefineCell(const long &cellId, const std::vector< long > &newVertexIds)
Definition: RefineGeometry.cpp:850
std::vector< long > greenRefineCell(const long &cellId, const long newVertexId, int iface)
Definition: RefineGeometry.cpp:908
RefineGeometry is an executable block class capable of refine a surface geometry.
Definition: RefineGeometry.hpp:92
void redgreenRefine(std::unordered_map< long, long > *mapping=nullptr, mimmo::MimmoSharedPointer< MimmoObject > coarsepatch=nullptr, mimmo::MimmoSharedPointer< MimmoObject > refinepatch=nullptr)
Definition: RefineGeometry.cpp:461
RefineGeometry & operator=(RefineGeometry other)
Definition: RefineGeometry.cpp:81
void smoothing(std::set< long > *constrainedVertices=nullptr)
Definition: RefineGeometry.cpp:959
void ternaryRefine(std::unordered_map< long, long > *mapping=nullptr, mimmo::MimmoSharedPointer< MimmoObject > coarsepatch=nullptr, mimmo::MimmoSharedPointer< MimmoObject > refinepatch=nullptr)
Definition: RefineGeometry.cpp:288
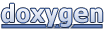