Primitive object generation. More...
#include <Primitive.hpp>
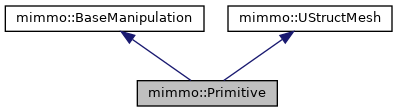
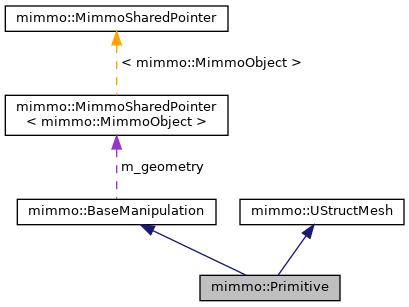
Protected Member Functions | |
void | swap (Primitive &) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
![]() | |
void | destroyNodalStructure () |
void | reshapeNodalStructure () |
void | resizeMesh () |
Detailed Description
Primitive object generation.
Basically, it builds an elemental 3D shape. The available shapes are: box, sphere, cylinder, wedge. A non-complete primitive can be defined by using custom span dimensions.
Ports available in Primitive class:
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_DIMENSION | setDimension | (MC_ARRAY3, MD_INT) |
M_INFLIMITS | setInfLimits | (MC_ARRAY3, MD_FLOAT) |
M_AXES | setRefSystem | (MC_ARR3ARR3, MD_FLOAT) |
M_SPAN | setSpan | (MC_ARRAY3, MD_FLOAT) |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_SHAPE | setShape(mimmo::ShapeType) | (MC_SCALAR, MD_SHAPET) |
M_SHAPEI | setShape(int) | (MC_SCALAR, MD_INT) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_COPYSHAPE | getShape | (MC_SCALAR, MD_SHAPE_) |
The xml available parameters, sections and subsections are the following:
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.Primitive
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class.
- OutputPlot: target directory for optional results writing.
Proper of the class:
- Shape: type of basic shape for your lattice. Available choice are CUBE, CYLINDER,SPHERE,WEDGE
- Origin: 3D point marking the shape barycenter
- Span: span dimensions of your shape (width-height-depth for CUBE, baseRadius-azimuthalspan-height for CYLINDER, radius-azimuthalspan-polarspan for SPHERE, triangle width-triangle height-depth for WEDGE)
- RefSystem: axes of current shape reference system. written in XML as:
<RefSystem>
<axis0> 1.0 0.0 0.0 </axis0>
<axis1> 0.0 1.0 0.0 </axis1>
<axis2> 0.0 0.0 1.0 </axis2>
</RefSystem>
- InfLimits: inferior limits for shape coordinates (meaningful only for CYLINDER AND SPHERE curvilinear coordinates)
Definition at line 92 of file Primitive.hpp.
Constructor & Destructor Documentation
◆ Primitive() [1/3]
mimmo::Primitive::Primitive | ( | ) |
Basic Constructor
Definition at line 32 of file Primitive.cpp.
◆ Primitive() [2/3]
mimmo::Primitive::Primitive | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 40 of file Primitive.cpp.
◆ ~Primitive()
|
virtual |
Destructor
Definition at line 53 of file Primitive.cpp.
◆ Primitive() [3/3]
mimmo::Primitive::Primitive | ( | const Primitive & | other | ) |
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 111 of file Primitive.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 74 of file Primitive.cpp.
◆ clearPrimitive()
void mimmo::Primitive::clearPrimitive | ( | ) |
Clean every setting and data hold by the class
Definition at line 94 of file Primitive.cpp.
◆ execute()
|
virtual |
Execute your object. Currently do nothing.
Implements mimmo::BaseManipulation.
Definition at line 103 of file Primitive.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 192 of file Primitive.cpp.
◆ swap()
|
protectednoexcept |
The documentation for this class was generated from the following files:
- src/core/Primitive.hpp
- src/core/Primitive.cpp
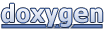