Primitive.cpp
77 built = (built && createPortIn<darray3E, Primitive>(this, &mimmo::Primitive::setInfLimits, M_INFLIMITS));
78 built = (built && createPortIn<dmatrix33E, Primitive>(this, &mimmo::Primitive::setRefSystem, M_AXES));
80 built = (built && createPortIn<darray3E, Primitive>(this, &mimmo::Primitive::setOrigin, M_POINT));
81 built = (built && createPortIn<mimmo::ShapeType, Primitive>(this, &mimmo::Primitive::setShape, M_SHAPE));
82 built = (built && createPortIn<const BasicShape *, Primitive>(this, &mimmo::Primitive::setShape, M_COPYSHAPE));
86 built = (built && createPortOut<BasicShape *, Primitive>(this, &mimmo::Primitive::getShape, M_COPYSHAPE));
@ CUBE
void setRefSystem(darray3E, darray3E, darray3E)
Definition: BasicMeshes.cpp:619
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setShape(ShapeType type=ShapeType::CUBE)
Definition: BasicMeshes.cpp:713
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: Primitive.cpp:111
@ WEDGE
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: Primitive.cpp:192
@ SPHERE
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void setInfLimits(double val, int dir)
Definition: BasicMeshes.cpp:588
@ CYLINDER
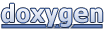