Executable block handling io of 3D surface polygonal mesh in *.obj format. More...
#include <IOWavefrontOBJ.hpp>
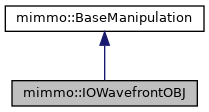
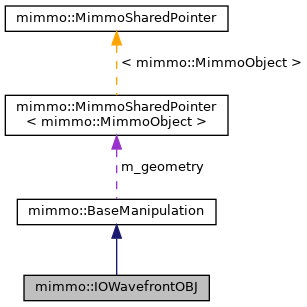
Public Types | |
enum | IOMode { IOMode::READ = 0, IOMode::RESTORE = 1, IOMode::WRITE = 2, IOMode::DUMP = 3 } |
Working mode for class IOWavefrontOBJ. More... | |
typedef std::map< long, std::map< long, std::vector< long > > > | TreeGroups |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
Protected Member Functions | |
void | buildPorts () |
void | dump (std::ostream &) |
void | read (const std::string &fullpath) |
void | readObjectData (std::ifstream &in, const std::streampos &begObjectStream, const long &PID, const std::string &defaultGroup, const std::array< long, 3 > &vCounter, long &vOffset, long &vnOffset, long &vTxtOffset, long &cOffset) |
TreeGroups | regroupCells (const WavefrontOBJData *objData, const livector1D &cellList) |
void | restore (std::istream &) |
std::string | searchMaterialFile (std::ifstream &in) |
void | searchObjectPosition (std::ifstream &in, std::vector< std::streampos > &mapPos, std::vector< std::string > &mapNames, std::vector< std::array< long, 3 >> &mapVCountObject, std::array< long, 3 > &mapVCountTotal, long &nCellTot) |
void | swap (IOWavefrontOBJ &x) noexcept |
void | write (const std::string &fullpath) |
void | writeObjectData (WavefrontOBJData *objData, std::ofstream &out, const std::array< std::vector< long >, 3 > &vertexLists, const std::vector< long > &cellList, std::array< long, 3 > &vOffsets, std::array< std::unordered_map< long, long >, 3 > &vinsertion_maps, const std::string &defaultGroup, long &activeGroup, long &activeMaterial, long &activeSmoothId) |
void | writeResumeFile () |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Executable block handling io of 3D surface polygonal mesh in *.obj format.
IOWavefrontOBJ manages reading/writing of surface mesh from/to WaveFront ASCII obj format to/from a MimmoObject surface mesh container (type=1). More information at https://en.wikipedia.org/wiki/Wavefront_.obj_file The class does not cover all the features supported by the format, but is restricted to description of 3D surface tessellated meshes. Points and Lines cell elements are not supported in the current class.
Materials file *.mtl attached to the inner *.obj file is not needed by the current class.
Thus, the obj file must have:
- Polygonal geometry statement only, No free form statement features (NURBS-CAD) are supported.
- Polygonal cells (only facets, no lines or vertex cells)
Mesh Object sub-parts are absorbed/flushed through PID/PIDNames mechanism of MimmoObject surface mesh cells. The class identifies as parts only sub-objects defined with o key entry (grouping with g key entry is ignored.)
Mesh accessory data are stored in a WaveFrontObjData class, containing:
- Textures and Normals properties (if any) are managed in independent MimmoObjects (their node coordinates list and their own connectivities).The link is that cell-ids are the same in all 3 objects (reference mesh, textures and normals).
- Materials assigned on cells, smoothing group ids and cellgroups id are managed as special fields attached to the MimmoObject mesh cells-ids.
WARNING 1: for reading/writing of v,vt,vn data chunks, the class supposes that the three of them are organized in the file in blocks of consecutive entries, i.e. after 'o' sub-object declaration all the v's of the object are written, then the vt's if any, and finally the vn's if any. This may be not true for the general Wavefront format(in which declarations can be random and not organized at all), but meet quite well with the practice of well known OBJ file exporter like the Blender's one.
WARNING 2: During OBJ writing if empty cellgroup entry is encountered, it will be assigned the object name (o) by default.
Ports available in IOWaveFrontOBJ Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
M_WAVEFRONTDATA | setData | (MC_SCALAR, MD_WOBJDATA_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR,MD_MIMMO_) |
M_WAVEFRONTDATA | getData | (MC_SCALAR, MD_WOBJDATA_) |
M_STRINGFIELD | getMaterials | (MC_SCALAR, MD_MPVECSTRING_) |
M_STRINGFIELD2 | getCellGroups | (MC_SCALAR, MD_MPVECSTRING_) |
M_LONGFIELD | getSmoothIds | (MC_SCALAR, MD_MPVECLONG_) |
M_GEOM2 | getTextures | (MC_SCALAR,MD_MIMMO_) |
M_GEOM3 | getNormals | (MC_SCALAR,MD_MIMMO_) |
M_NAME | getMaterialFile | (MC_SCALAR, MD_STRING) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.IOWavefrontOBJ
; - Priority: uint marking priority in multi-chain execution;
Proper of the class:
- IOMode: 0-read from obj file, 1-restore from class dump file, 2- write to obj file, 3-write class dump file.
- Dir directory path to read from/write to
- Filename name of the file to be read/written (without tag .obj)
- GeomTolerance (float) geometric tolerance for repeated mesh vertices.
- CleanDoubleMeshVertices: for READ Mode only, boolean 0/1 if 1, force mesh vertices collapsing after reading with GeomTolerance.
- IgnoreCellGroups: for READ Mode only, boolean 0/1 if 1, ignore cellgroups labeling of the OBJ mesh.
- TextureUVMode: for WRITE Mode only, boolean 0/1 if 1, force writing textures with first 2 components only.
- PrintResumeFile: 0/1 print a resume file of the mesh contents after execution.
Geometry and additional fields have to be mandatorily passed through ports.
- Examples
- genericinput_example_00005.cpp.
Definition at line 336 of file IOWavefrontOBJ.hpp.
Member Typedef Documentation
◆ TreeGroups
typedef std::map<long,std::map<long, std::vector<long> > > mimmo::IOWavefrontOBJ::TreeGroups |
custom typedef for IOWavefrontOBJ class
Definition at line 339 of file IOWavefrontOBJ.hpp.
Constructor & Destructor Documentation
◆ IOWavefrontOBJ() [1/2]
mimmo::IOWavefrontOBJ::IOWavefrontOBJ | ( | IOWavefrontOBJ::IOMode | mode = IOMode::READ | ) |
Constructor
- Parameters
-
[in] mode working mode of the class - see IOWavefrontOBJ::IOMode enum
Definition at line 1138 of file IOWavefrontOBJ.cpp.
◆ ~IOWavefrontOBJ()
|
virtual |
Destructor
Definition at line 1190 of file IOWavefrontOBJ.cpp.
◆ IOWavefrontOBJ() [2/2]
mimmo::IOWavefrontOBJ::IOWavefrontOBJ | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 1155 of file IOWavefrontOBJ.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
Absorb class parameters from a section xml
- Parameters
-
[in] slotXML xml section [in] name unused string
Reimplemented from mimmo::BaseManipulation.
Definition at line 1445 of file IOWavefrontOBJ.cpp.
◆ buildPorts()
|
protectedvirtual |
Building class ports
Implements mimmo::BaseManipulation.
Definition at line 1215 of file IOWavefrontOBJ.cpp.
◆ dump()
|
protected |
write mesh and data attached to class own dump file
Definition at line 1999 of file IOWavefrontOBJ.cpp.
◆ execute()
|
virtual |
Class workflow execution
Implements mimmo::BaseManipulation.
Definition at line 1547 of file IOWavefrontOBJ.cpp.
◆ flushSectionXML()
|
virtual |
Flush class parameters to a section xml
- Parameters
-
[in] slotXML xml section to be filled [in] name unused string
Reimplemented from mimmo::BaseManipulation.
Definition at line 1528 of file IOWavefrontOBJ.cpp.
◆ getCellGroups()
MimmoPiercedVector< std::string > * mimmo::IOWavefrontOBJ::getCellGroups | ( | ) |
Return pointer to WavefrontOBJData cellgroups attached to polygonal mesh. Data are referred always to cell.
- Returns
- cellgroups data attached to the mesh
Definition at line 1307 of file IOWavefrontOBJ.cpp.
◆ getData()
WavefrontOBJData * mimmo::IOWavefrontOBJ::getData | ( | ) |
Return pointer to WavefrontOBJData (materials, smoothids, textures and normals, etc...) attached to polygonal mesh. Data are referred to cell (materials, smoothids ...) or points(texture, normals, ...).
- Returns
- data attached to the mesh
Definition at line 1262 of file IOWavefrontOBJ.cpp.
◆ getMaterialFile()
std::string mimmo::IOWavefrontOBJ::getMaterialFile | ( | ) |
Return string with filename with materials lib.
- Returns
- texture data
Definition at line 1285 of file IOWavefrontOBJ.cpp.
◆ getMaterials()
MimmoPiercedVector< std::string > * mimmo::IOWavefrontOBJ::getMaterials | ( | ) |
Return pointer to WavefrontOBJData materials attached to polygonal mesh. Data are referred always to cell.
- Returns
- materials data attached to the mesh
Definition at line 1296 of file IOWavefrontOBJ.cpp.
◆ getNormals()
MimmoSharedPointer< MimmoObject > mimmo::IOWavefrontOBJ::getNormals | ( | ) |
◆ getSmoothIds()
MimmoPiercedVector< long > * mimmo::IOWavefrontOBJ::getSmoothIds | ( | ) |
Return pointer to WavefrontOBJData smoothids attached to polygonal mesh. Data are referred always to cell.
- Returns
- materials data attached to the mesh
Definition at line 1318 of file IOWavefrontOBJ.cpp.
◆ getSubParts()
std::unordered_map< long, std::string > mimmo::IOWavefrontOBJ::getSubParts | ( | ) |
Return the map with PID and name of the object which the mesh is subdivided in. In read/restore mode, this subdivision is extrapolated from file obj, in write/dump mode this refers to subdivision hold by the geometry set with setGeometry method.
- Returns
- pid/name mesh internal subdivision.
Definition at line 1275 of file IOWavefrontOBJ.cpp.
◆ getTextures()
MimmoSharedPointer< MimmoObject > mimmo::IOWavefrontOBJ::getTextures | ( | ) |
◆ printResumeFile()
void mimmo::IOWavefrontOBJ::printResumeFile | ( | bool | ) |
If set to true print a resume file of the data referenced by the mesh.
- Parameters
-
[in] print true/false
- Examples
- genericinput_example_00005.cpp.
Definition at line 1436 of file IOWavefrontOBJ.cpp.
◆ read()
|
protected |
Read mesh and data attached from file obj.
Definition at line 1601 of file IOWavefrontOBJ.cpp.
◆ readObjectData()
|
protected |
Get the stream to the begin of a Object section and start absorb its data, i.e.
- v: vertices coordinates
- vt: texture coordinates (if any)
- vn: normals referred to vertices (if any)
- f : facet connectivity
- usemtl : all the cells after the keyword will be assigned to the material specified by mtllib file (if any)
- g: all the cells after the keyword will be assigned to cell group specified by g (if any)
- s: all the cells after the keyword will be assigned to smooth group specified by s (if any)
- vp, vertex in free form statement, is ignored. A warning will be raised on logger.
- l, line connectivity, is ignored. A warning will be raised on logger.
- p, point connectivity, is ignored. A warning will be raised on logger.
all other keywords are ignored.
Data are directly stored in m_intPatch (for main mesh), m_intTexture(for texture) and m_intData(for attached WavefrontOBJData datatypes)
- Parameters
-
[in] in reading stream [in] begObjectStream position of the stream to start absorbing data [in] PID part identifier assigned to the current object. [in] defaultGroup track the name of the current object. [in] vCounter 3 element array carrying the number of v, vt and vn currently present in this sub-part. [in,out] vOffset i/o current id of the next-to-be inserted mesh vertex. [in,out] vnOffset i/o current id of the next-to-be inserted mesh vertex normal. [in,out] vTxtOffset i/o current id of the next-to-be inserted texture vertex. [in,out] cOffset i/o current id of the next-to-be inserted mesh cell (same for mesh and texture)
Definition at line 2223 of file IOWavefrontOBJ.cpp.
◆ regroupCells()
|
protected |
Regroup a list of mesh cells by cellgroups-materials
- Parameters
-
[in] objData source object data structure [in] cellList list of cell ids of the polygonal mesh
- Returns
- tree containing cell sublists reordered as [CellGroup][Material] -> sublist.
Definition at line 2606 of file IOWavefrontOBJ.cpp.
◆ restore()
|
protected |
Read mesh and data attached from class own dump file
Definition at line 1966 of file IOWavefrontOBJ.cpp.
◆ searchMaterialFile()
|
protected |
Search for material file name marked with entry key "mtllib". If none, return an empty string
- Returns
- materials file name
Definition at line 2097 of file IOWavefrontOBJ.cpp.
◆ searchObjectPosition()
|
protected |
While reading, scan the obj file to get the number of subparts, their stream positions and names eventually. Restore the stream to file begin after searching.
- Parameters
-
[in] in reading obj file stream [in,out] mapPos map pid/streampos to be filled [in,out] mapNames map pid/names to be filled [in,out] mapVCountObject list of 3elements array containing number of v, vt,and vn for each sub-part. [out] mapVCountTotal 3elements array containing total number of v, vt,and vn in the obj file [out] nCellTot total cells in the obj file
Definition at line 2131 of file IOWavefrontOBJ.cpp.
◆ setCleanDoubleMeshVertices()
void mimmo::IOWavefrontOBJ::setCleanDoubleMeshVertices | ( | bool | clean | ) |
For READ mode only, if true force double mesh vertices collapsing inside geometric tolerance m_tol (setGeometryTolerance method). This collapsing will not affect wavefront obj data attached to the mesh. Do nothing if false.
- Parameters
-
[in] clean true/false
Definition at line 1412 of file IOWavefrontOBJ.cpp.
◆ setData()
void mimmo::IOWavefrontOBJ::setData | ( | WavefrontOBJData * | data | ) |
Set data attached to surface polygonal mesh, i.e:
- materials associated to cells
- cell group labels associated to cells
- smoothing group ids associated to cells
- texture and vnormals list if any
- material file path. Meaningful only in write mode. Does nothing in read mode. Beware any other data are erased.
- Parameters
-
[in] data pointer to a WavefrontObjData container.
Definition at line 1372 of file IOWavefrontOBJ.cpp.
◆ setDir()
void mimmo::IOWavefrontOBJ::setDir | ( | const std::string & | pathdir | ) |
Set the directory where the read file is searched or the written file has to be placed.
- Parameters
-
[in] pathdir path to reference directory for I/O purposes
- Examples
- genericinput_example_00005.cpp.
Definition at line 1384 of file IOWavefrontOBJ.cpp.
◆ setFilename()
void mimmo::IOWavefrontOBJ::setFilename | ( | const std::string & | name | ) |
Set the name of the file to be read or written. No format tag .obj has to be specified.
- Parameters
-
[in] name of the file
- Examples
- genericinput_example_00005.cpp.
Definition at line 1393 of file IOWavefrontOBJ.cpp.
◆ setGeometry()
void mimmo::IOWavefrontOBJ::setGeometry | ( | MimmoSharedPointer< MimmoObject > | geo | ) |
Set the geometry meant to be written. Does nothing in read/restore mode.
- Parameters
-
[in] geo MimmoObject surface mesh of type 1
Definition at line 1353 of file IOWavefrontOBJ.cpp.
◆ setGeometryTolerance()
void mimmo::IOWavefrontOBJ::setGeometryTolerance | ( | double | tolerance | ) |
Set the geometric tolerance for duplicated vertices/vertex normals collapsing
- Parameters
-
[in] tolerance
Definition at line 1401 of file IOWavefrontOBJ.cpp.
◆ setIgnoreCellGroups()
void mimmo::IOWavefrontOBJ::setIgnoreCellGroups | ( | bool | ignore | ) |
For READ mode only, if true skip absorbing cellgroup g infromation of the OBJ mesh.
- Parameters
-
[in] ignore true/false
Definition at line 1420 of file IOWavefrontOBJ.cpp.
◆ setTextureUVMode()
void mimmo::IOWavefrontOBJ::setTextureUVMode | ( | bool | UVmode | ) |
For WRITE mode only, if any textures is available in wavefront optional data force writing textures with only the first two components U and V (ignoring optional W components.).
- Parameters
-
[in] UVmode true/false to activate/deactivate texture UV mode.
- Examples
- genericinput_example_00005.cpp.
Definition at line 1428 of file IOWavefrontOBJ.cpp.
◆ swap()
|
protectednoexcept |
Swap utility
- Parameters
-
[in] x other object to swap from
Definition at line 1196 of file IOWavefrontOBJ.cpp.
◆ whichMode()
IOWavefrontOBJ::IOMode mimmo::IOWavefrontOBJ::whichMode | ( | ) |
- Returns
- working mode of the class, see IOWavefrontOBJ::IOMode enum.
Definition at line 1245 of file IOWavefrontOBJ.cpp.
◆ whichModeInt()
int mimmo::IOWavefrontOBJ::whichModeInt | ( | ) |
- Returns
- working mode of the class as integer, see IOWavefrontOBJ::IOMode enum.
Definition at line 1252 of file IOWavefrontOBJ.cpp.
◆ write()
|
protected |
Write mesh and data attached to file obj
Definition at line 1864 of file IOWavefrontOBJ.cpp.
◆ writeObjectData()
|
protected |
Write data of an object to OBJ output stream. Write, only:
- v: vertices coordinates
- vt: texture coordinates (if any)
- vn: normals referred to vertices
- f : facet connectivity
- usemtl : all the cells after the keyword will be assigned to the material specified by mtllib file (if any)
- g: all the cells after the keyword will be assigned to cell group specified by g (if any)
- s: all the cells after the keyword will be assigned to smooth group specified by s (if any)
Data are taken from m_geometry (for main mesh), m_extTexture(for texture) and m_extData(for attached WavefrontOBJData datatypes)
- Parameters
-
[in] objData source object data structure [in] out writing stream [in] vertexLists list of points involved for the object for all 3 type v, vt, vn [in] cellList list of cells involved for the object [in,out] vOffsets offset for local v,vt,vn counting [in,out] vinsertion_maps local map id-written insertion index for v,vt,vn (to avoid rewrite vertices) [in] defaultGroup name of the current object part (save all empty cellgroups entry in defaultGroup). [in,out] activeGroup currently active cellgroup for the mesh [in,out] activeMaterial currently active material for the mesh [in,out] activeSmoothId currently active smoothids for the mesh
Definition at line 2467 of file IOWavefrontOBJ.cpp.
◆ writeResumeFile()
|
protected |
Print the resume file
Definition at line 2023 of file IOWavefrontOBJ.cpp.
The documentation for this class was generated from the following files:
- src/iogeneric/IOWavefrontOBJ.hpp
- src/iogeneric/IOWavefrontOBJ.cpp
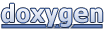