IOWavefrontOBJ.hpp
434 long pushCell(MimmoSharedPointer<MimmoObject> geo, std::vector<long> &conn, long PID, long id, int rank = -1 );
void swap(WavefrontOBJData &x) noexcept
Definition: IOWavefrontOBJ.cpp:53
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: IOWavefrontOBJ.cpp:1445
OverlapAnnotationMode getMultipleAnnotationStrategy()
Definition: IOWavefrontOBJ.cpp:402
void setRecomputeNormalsCells(MimmoPiercedVector< long > *cellList)
Definition: IOWavefrontOBJ.cpp:454
void computeAnnotations()
Definition: IOWavefrontOBJ.cpp:806
void setGeometryTolerance(double tolerance)
Definition: IOWavefrontOBJ.cpp:1401
@ ANGLE_WEIGHTED
std::unordered_map< long, std::string > inv_materialsList
Definition: IOWavefrontOBJ.hpp:64
void clearRecomputeNormalsCells()
Definition: IOWavefrontOBJ.cpp:590
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: IOWavefrontOBJ.cpp:625
void swap(ManipulateWFOBJData &x) noexcept
Definition: IOWavefrontOBJ.cpp:347
MimmoSharedPointer< MimmoObject > textures
Definition: IOWavefrontOBJ.hpp:56
void autoCompleteCellFields()
Definition: IOWavefrontOBJ.cpp:137
void setDir(const std::string &pathdir)
Definition: IOWavefrontOBJ.cpp:1384
void setTextureUVMode(bool UVmode)
Definition: IOWavefrontOBJ.cpp:1428
std::string searchMaterialFile(std::ifstream &in)
Definition: IOWavefrontOBJ.cpp:2097
void read(const std::string &fullpath)
Definition: IOWavefrontOBJ.cpp:1601
void setPinCellGroups(const std::vector< std::string > &cellgroupsList)
Definition: IOWavefrontOBJ.cpp:543
std::unordered_map< std::string, long > smoothidsList
Definition: IOWavefrontOBJ.hpp:61
std::unordered_set< std::string > m_pinObjects
Definition: IOWavefrontOBJ.hpp:238
NormalsComputeMode getNormalsComputeStrategy()
Definition: IOWavefrontOBJ.cpp:411
std::vector< MimmoPiercedVector< long > > m_annotations
Definition: IOWavefrontOBJ.hpp:233
std::string getMaterialFile()
Definition: IOWavefrontOBJ.cpp:1285
MimmoSharedPointer< MimmoObject > getNormals()
Definition: IOWavefrontOBJ.cpp:1342
Executable block handling io of 3D surface polygonal mesh in *.obj format.
Definition: IOWavefrontOBJ.hpp:336
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: IOWavefrontOBJ.cpp:1353
MimmoPiercedVector< std::string > materials
Definition: IOWavefrontOBJ.hpp:52
std::unordered_map< std::string, long > cellgroupsList
Definition: IOWavefrontOBJ.hpp:62
TreeGroups regroupCells(const WavefrontOBJData *objData, const livector1D &cellList)
Definition: IOWavefrontOBJ.cpp:2606
MimmoPiercedVector< std::string > cellgroups
Definition: IOWavefrontOBJ.hpp:54
void setCheckNormalsMagnitude(bool flag)
Definition: IOWavefrontOBJ.cpp:493
void checkNormalsMagnitude()
Definition: IOWavefrontOBJ.cpp:786
void searchObjectPosition(std::ifstream &in, std::vector< std::streampos > &mapPos, std::vector< std::string > &mapNames, std::vector< std::array< long, 3 >> &mapVCountObject, std::array< long, 3 > &mapVCountTotal, long &nCellTot)
Definition: IOWavefrontOBJ.cpp:2131
void readObjectData(std::ifstream &in, const std::streampos &begObjectStream, const long &PID, const std::string &defaultGroup, const std::array< long, 3 > &vCounter, long &vOffset, long &vnOffset, long &vTxtOffset, long &cOffset)
Definition: IOWavefrontOBJ.cpp:2223
MimmoSharedPointer< MimmoObject > normals
Definition: IOWavefrontOBJ.hpp:57
void addAnnotation(MimmoPiercedVector< long > *annotation)
Definition: IOWavefrontOBJ.cpp:481
void setMultipleAnnotationStrategy(OverlapAnnotationMode mode)
Definition: IOWavefrontOBJ.cpp:505
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
std::unordered_map< long, std::string > getSubParts()
Definition: IOWavefrontOBJ.cpp:1275
Executable block manipulating optional data of WavefrontOBJ mesh.
Definition: IOWavefrontOBJ.hpp:158
std::vector< long > getPinnedCellGroup()
Definition: IOWavefrontOBJ.cpp:428
void setIgnoreCellGroups(bool ignore)
Definition: IOWavefrontOBJ.cpp:1420
bool m_checkNormalsMag
Definition: IOWavefrontOBJ.hpp:234
void extractPinnedLists()
Definition: IOWavefrontOBJ.cpp:984
void setFilename(const std::string &name)
Definition: IOWavefrontOBJ.cpp:1393
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
std::unordered_map< long, std::string > inv_cellgroupsList
Definition: IOWavefrontOBJ.hpp:66
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: IOWavefrontOBJ.cpp:1528
MimmoPiercedVector< std::string > * getCellGroups()
Definition: IOWavefrontOBJ.cpp:1307
MimmoPiercedVector< long > * getSmoothIds()
Definition: IOWavefrontOBJ.cpp:1318
void setPinSmoothIds(const std::vector< int > &smoothidsList)
Definition: IOWavefrontOBJ.cpp:559
OverlapAnnotationMode m_annMode
Definition: IOWavefrontOBJ.hpp:235
std::unordered_set< std::string > m_pinCellGroups
Definition: IOWavefrontOBJ.hpp:240
MimmoPiercedVector< std::string > * getMaterials()
Definition: IOWavefrontOBJ.cpp:1296
void setData(WavefrontOBJData *data)
Definition: IOWavefrontOBJ.cpp:1372
std::array< double, 3 > evalVNormal(bitpit::SurfaceKernel *mesh, long idCell, int locVertex)
Definition: IOWavefrontOBJ.cpp:1064
MimmoSharedPointer< MimmoObject > refGeometry
Definition: IOWavefrontOBJ.hpp:70
NormalsComputeMode m_normalsMode
Definition: IOWavefrontOBJ.hpp:236
MimmoPiercedVector< long > m_normalsCells
Definition: IOWavefrontOBJ.hpp:232
void clearAnnotations()
Definition: IOWavefrontOBJ.cpp:584
void setNormalsComputeStrategy(NormalsComputeMode mode)
Definition: IOWavefrontOBJ.cpp:516
struct for storing cell data attached to Wavefront OBJ polygonal mesh
Definition: IOWavefrontOBJ.hpp:45
std::vector< long > getPinnedObjectGroup()
Definition: IOWavefrontOBJ.cpp:446
std::unordered_set< std::string > m_pinMaterials
Definition: IOWavefrontOBJ.hpp:239
MimmoSharedPointer< MimmoObject > getTextures()
Definition: IOWavefrontOBJ.cpp:1329
NormalsComputeMode
Strategy Mode to recompute Wavefront vertex normals on a set of candidate cells.
Definition: IOWavefrontOBJ.hpp:177
std::vector< long > getPinnedMaterialGroup()
Definition: IOWavefrontOBJ.cpp:420
void setData(WavefrontOBJData *data)
Definition: IOWavefrontOBJ.cpp:465
void setCleanDoubleMeshVertices(bool clean)
Definition: IOWavefrontOBJ.cpp:1412
std::unordered_map< long, std::string > inv_smoothidsList
Definition: IOWavefrontOBJ.hpp:65
MimmoPiercedVector< long > smoothids
Definition: IOWavefrontOBJ.hpp:53
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: IOWavefrontOBJ.cpp:728
void setPinObjects(const std::vector< std::string > &objectsList)
Definition: IOWavefrontOBJ.cpp:570
bool getCheckNormalsMagnitude()
Definition: IOWavefrontOBJ.cpp:393
std::unordered_set< int > m_pinSmoothIds
Definition: IOWavefrontOBJ.hpp:241
ManipulateWFOBJData()
Definition: IOWavefrontOBJ.cpp:307
IOWavefrontOBJ(IOMode mode=IOMode::READ)
Definition: IOWavefrontOBJ.cpp:1138
void write(const std::string &fullpath)
Definition: IOWavefrontOBJ.cpp:1864
OverlapAnnotationMode
Strategy Mode to deal with Multiple Annotations on a target cell in ManipulateWFOBJData.
Definition: IOWavefrontOBJ.hpp:165
void printResumeFile(bool print)
Definition: IOWavefrontOBJ.cpp:1436
void writeObjectData(WavefrontOBJData *objData, std::ofstream &out, const std::array< std::vector< long >, 3 > &vertexLists, const std::vector< long > &cellList, std::array< long, 3 > &vOffsets, std::array< std::unordered_map< long, long >, 3 > &vinsertion_maps, const std::string &defaultGroup, long &activeGroup, long &activeMaterial, long &activeSmoothId)
Definition: IOWavefrontOBJ.cpp:2467
virtual ~ManipulateWFOBJData()
Definition: IOWavefrontOBJ.cpp:341
std::array< std::vector< long >, 4 > m_pinnedCellLists
Definition: IOWavefrontOBJ.hpp:243
virtual ~WavefrontOBJData()
Definition: IOWavefrontOBJ.hpp:74
std::unordered_map< std::string, long > materialsList
Definition: IOWavefrontOBJ.hpp:60
std::vector< long > getPinnedSmoothGroup()
Definition: IOWavefrontOBJ.cpp:437
std::map< long, std::map< long, std::vector< long > > > TreeGroups
Definition: IOWavefrontOBJ.hpp:339
void setPinMaterials(const std::vector< std::string > &materialsList)
Definition: IOWavefrontOBJ.cpp:526
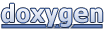