Executable block manipulating optional data of WavefrontOBJ mesh. More...
#include <IOWavefrontOBJ.hpp>
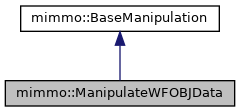

Public Types | |
enum | NormalsComputeMode { NormalsComputeMode::FLAT_BITPIT = 0, NormalsComputeMode::AREA_WEIGHTED = 1, NormalsComputeMode::ANGLE_WEIGHTED = 2 } |
Strategy Mode to recompute Wavefront vertex normals on a set of candidate cells. More... | |
enum | OverlapAnnotationMode { OverlapAnnotationMode::HARD = 0, OverlapAnnotationMode::SOFT = 1, OverlapAnnotationMode::GETALL = 2, OverlapAnnotationMode::GETALLNOBLANKS = 3 } |
Strategy Mode to deal with Multiple Annotations on a target cell in ManipulateWFOBJData. More... | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
Protected Member Functions | |
void | buildPorts () |
void | checkNormalsMagnitude () |
void | computeAnnotations () |
void | computeNormals () |
std::array< double, 3 > | evalVNormal (bitpit::SurfaceKernel *mesh, long idCell, int locVertex) |
void | extractPinnedLists () |
void | swap (ManipulateWFOBJData &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
OverlapAnnotationMode | m_annMode |
std::vector< MimmoPiercedVector< long > > | m_annotations |
bool | m_checkNormalsMag |
WavefrontOBJData * | m_extData |
MimmoPiercedVector< long > | m_normalsCells |
NormalsComputeMode | m_normalsMode |
std::unordered_set< std::string > | m_pinCellGroups |
std::unordered_set< std::string > | m_pinMaterials |
std::array< std::vector< long >, 4 > | m_pinnedCellLists |
std::unordered_set< std::string > | m_pinObjects |
std::unordered_set< int > | m_pinSmoothIds |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Executable block manipulating optional data of WavefrontOBJ mesh.
The class performs manipulation of Wavefront mesh optional data such as:
- cell annotation (annotating a group of cells throughout the sub-objects of the mesh). Different strategies for multiple annotations on a single cell are available. See OverlapAnnotationMode enum. OverlapAnnotationMode::HARD is class default.
- recompute vertex normals on nodes of a list of candidate cells of the mesh. Once a list of mesh cell-ids is provided data on normals (stored in WavefrontOBJData) are recomputed according to NormalsComputeMode enum. NormalsComputeMode::FLAT_BITPIT is the default. Please note that recomputing normals will modify MimmoObject internal structures, so its properties (kdtrees, adjacencies etc..) will be invalidated.
recover list of cell-ids referring to target Wavefront data cell properties such as materials, cellgroups, smoothids (f.e. extract all mesh cell-ids with material property "body").
In parallel the geometry is supposed owned entirely by the master rank 0. The mesh is read/written only by the master rank.
Ports available in ManipulateWFOBJData Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_WAVEFRONTDATA | setData | (MC_SCALAR, MD_WOBJDATA_) |
M_LONGFIELD | addAnnotation | (MC_SCALAR, MD_MPVECLONG_) |
M_LONGFIELD2 | setRecomputeNormalsCells | (MC_SCALAR, MD_MPVECLONG_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_WAVEFRONTDATA | getData | (MC_SCALAR, MD_WOBJDATA_) |
M_VECTORLI | getPinnedMaterialGroup | (MC_VECTOR, MD_LONG) |
M_VECTORLI2 | getPinnedCellGroup | (MC_VECTOR, MD_LONG) |
M_VECTORLI3 | getPinnedSmoothGroup | (MC_VECTOR, MD_LONG) |
M_VECTORLI4 | getPinnedObjectGroup | (MC_VECTOR, MD_LONG) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.ManipulateWFOBJData
; - Priority: uint marking priority in multi-chain execution;
Proper of the class:
- CheckNormalsMagnitude: 0/1 boolean, if 1, check and force Wavefront Data to have normals magnitude equal to 1. If no normals are available in wavefront data it does nothing.
- MultipleAnnotationStrategy: 0/1/2 choose strategy to deal with multiple annotations concurring into a target cell. see enum OverlapAnnotationMode. Default is 0 - FAWIN.
- NormalsComputeStrategy: 0/1/2 choose strategy to recompute normals on candidate cells, see NormalsComputeMode enum.
- PinObjects list of objects/subparts names (blank separated) to pin mesh cells belonging to them. Cells-ids will be available with method getPinnedObjectGroup method/M_VECTORLI4 port.
- PinMaterials list of materials names (blank separated) to pin mesh cells owning these properties. Cells-ids will be available with method getPinnedMaterialGroup method/M_VECTORLI port.
- PinCellGroups list of cellgroups names (blank separated) to pin mesh cells owning these properties. Cells-ids will be available with method getPinnedCellGroup method/M_VECTORLI2 port.
- PinSmoothIds list of smooth-ids int (blank separated) to pin mesh cells owning these properties. Cells-ids will be available with method getPinnedSmoothGroup method/M_VECTORLI3 port.
Data and additional input fields have to be mandatorily passed through ports.
Definition at line 158 of file IOWavefrontOBJ.hpp.
Constructor & Destructor Documentation
◆ ManipulateWFOBJData() [1/2]
mimmo::ManipulateWFOBJData::ManipulateWFOBJData | ( | ) |
Constructor
Definition at line 307 of file IOWavefrontOBJ.cpp.
◆ ~ManipulateWFOBJData()
|
virtual |
Destructor
Definition at line 341 of file IOWavefrontOBJ.cpp.
◆ ManipulateWFOBJData() [2/2]
mimmo::ManipulateWFOBJData::ManipulateWFOBJData | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 319 of file IOWavefrontOBJ.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
Absorb class parameters from a section xml
- Parameters
-
[in] slotXML xml section [in] name unused string
Reimplemented from mimmo::BaseManipulation.
Definition at line 625 of file IOWavefrontOBJ.cpp.
◆ addAnnotation()
void mimmo::ManipulateWFOBJData::addAnnotation | ( | MimmoPiercedVector< long > * | data | ) |
Add a field of cell ids as annotation for the Wavefront mesh. Annotated cell ids will be redistributed in cellgroups member of the WavefrontOBJData. Strategies to deal with cell referred by multiple annotations are summed up in setMultipleAnnotationStrategy method and OverlapAnnotationMode enum BEWARE: Annotation name/string mark must be specified as name of the MimmoPiercedVector structure.
Definition at line 481 of file IOWavefrontOBJ.cpp.
◆ buildPorts()
|
protectedvirtual |
Building class ports
Implements mimmo::BaseManipulation.
Definition at line 366 of file IOWavefrontOBJ.cpp.
◆ checkNormalsMagnitude()
|
protected |
Check and fix normals magnitudes. They must be always unitary. It assumes m_extData has already a valid normals MimmoObject in it, no check is perfomed internally.
Definition at line 786 of file IOWavefrontOBJ.cpp.
◆ clear()
void mimmo::ManipulateWFOBJData::clear | ( | ) |
Clear all class data and reset to defaults.
Definition at line 609 of file IOWavefrontOBJ.cpp.
◆ clearAnnotations()
void mimmo::ManipulateWFOBJData::clearAnnotations | ( | ) |
Empty the list of annotations
Definition at line 584 of file IOWavefrontOBJ.cpp.
◆ clearPinLists()
void mimmo::ManipulateWFOBJData::clearPinLists | ( | ) |
Empty the pinLists
Definition at line 596 of file IOWavefrontOBJ.cpp.
◆ clearRecomputeNormalsCells()
void mimmo::ManipulateWFOBJData::clearRecomputeNormalsCells | ( | ) |
Empty the list of cells for normals re-computation
Definition at line 590 of file IOWavefrontOBJ.cpp.
◆ computeAnnotations()
|
protected |
Compute annotations and send it as new pids to the reference geometry. New Created PID will be named as the oldPid (if any) + _name of annotation.
Definition at line 806 of file IOWavefrontOBJ.cpp.
◆ computeNormals()
|
protected |
Computing vertex normals on candidates of a mesh cell list a store it in WavefrontOBJData::normals structure;
Definition at line 866 of file IOWavefrontOBJ.cpp.
◆ evalVNormal()
|
protected |
Given a physical mesh, the target vertex id and its ring of cells compute the average normal on vertex id according to the strategy selected in m_normalsMode member. the target vertex is recognized through the cell id and local position in cell connectivity.
- Parameters
-
[in] mesh pointer to the mesh [in] idCell id of the reference cell [in] locVertex local index of the target vertex w.r.t cell connectivity chunk
Definition at line 1064 of file IOWavefrontOBJ.cpp.
◆ execute()
|
virtual |
Class workflow execution
Implements mimmo::BaseManipulation.
Definition at line 764 of file IOWavefrontOBJ.cpp.
◆ extractPinnedLists()
|
protected |
Extract pinned cell lists;
Definition at line 984 of file IOWavefrontOBJ.cpp.
◆ flushSectionXML()
|
virtual |
Flush class parameters to a section xml
- Parameters
-
[in] slotXML xml section to be filled [in] name unused string
Reimplemented from mimmo::BaseManipulation.
Definition at line 728 of file IOWavefrontOBJ.cpp.
◆ getCheckNormalsMagnitude()
bool mimmo::ManipulateWFOBJData::getCheckNormalsMagnitude | ( | ) |
- Returns
- true if check of Normals magnitude is active. see setCheckNormalsMagnitude method
Definition at line 393 of file IOWavefrontOBJ.cpp.
◆ getData()
WavefrontOBJData * mimmo::ManipulateWFOBJData::getData | ( | ) |
Return pointer to WavefrontOBJData (materials, smoothids, textures and normals, etc...) linked.
- Returns
- data attached to the Wavefront mesh
Definition at line 386 of file IOWavefrontOBJ.cpp.
◆ getMultipleAnnotationStrategy()
ManipulateWFOBJData::OverlapAnnotationMode mimmo::ManipulateWFOBJData::getMultipleAnnotationStrategy | ( | ) |
- Returns
- active strategy mode for multiple annotations handling on a sigle cell. see setMultipleAnnotationStrategy method
Definition at line 402 of file IOWavefrontOBJ.cpp.
◆ getNormalsComputeStrategy()
ManipulateWFOBJData::NormalsComputeMode mimmo::ManipulateWFOBJData::getNormalsComputeStrategy | ( | ) |
- Returns
- active strategy mode for vertex normals computation on a list of candidate cells nodes. see setNormalsComputeStrategy method
Definition at line 411 of file IOWavefrontOBJ.cpp.
◆ getPinnedCellGroup()
std::vector< long > mimmo::ManipulateWFOBJData::getPinnedCellGroup | ( | ) |
- Returns
- cell list pinned by cellgroup names specified with setPinCellGroups method. Return Empty list if cellgroup are not present in Wavefront data linked to the class.
Definition at line 428 of file IOWavefrontOBJ.cpp.
◆ getPinnedMaterialGroup()
std::vector< long > mimmo::ManipulateWFOBJData::getPinnedMaterialGroup | ( | ) |
- Returns
- cell list pinned by material names specified with setPinMaterials method. Return Empty list if materials are not present in Wavefront data linked to the class.
Definition at line 420 of file IOWavefrontOBJ.cpp.
◆ getPinnedObjectGroup()
std::vector< long > mimmo::ManipulateWFOBJData::getPinnedObjectGroup | ( | ) |
- Returns
- cell list pinned by object/subpart names specified with setPinObjects method. Return Empty list if object subdivision is not present in Wavefront data linked to the class (pid of refGeometry member).
Definition at line 446 of file IOWavefrontOBJ.cpp.
◆ getPinnedSmoothGroup()
std::vector< long > mimmo::ManipulateWFOBJData::getPinnedSmoothGroup | ( | ) |
- Returns
- cell list pinned by smoothids int entries specified with setPinSmoothIds method. Return Empty list if smooth ids are not present in Wavefront data linked to the class.
Definition at line 437 of file IOWavefrontOBJ.cpp.
◆ setCheckNormalsMagnitude()
void mimmo::ManipulateWFOBJData::setCheckNormalsMagnitude | ( | bool | flag | ) |
If any vertex normals data are present in linked Wavefront data object, check their magnitudes to be always unitary, and if not fix them.
- Parameters
-
[in] flag true/false to activate check & fix of normals magnitudes.
Definition at line 493 of file IOWavefrontOBJ.cpp.
◆ setData()
void mimmo::ManipulateWFOBJData::setData | ( | WavefrontOBJData * | data | ) |
Link the target WavefrontOBJData (materials, smoothids, textures and normals, etc...) to be manipulated.
- Parameters
-
[in] data attached to the Wavefront mesh
Definition at line 465 of file IOWavefrontOBJ.cpp.
◆ setMultipleAnnotationStrategy()
void mimmo::ManipulateWFOBJData::setMultipleAnnotationStrategy | ( | ManipulateWFOBJData::OverlapAnnotationMode | mode | ) |
Set the strategy mode to handle multiple concurrent annotation markers on the same cell. Available strategies are summed up in ManipulateWFOBJData::OverlapAnnotationMode enum. Class default strategy is OverlapAnnotationMode::HARD method.
- Parameters
-
[in] mode multiple annotations handling strategy.
Definition at line 505 of file IOWavefrontOBJ.cpp.
◆ setNormalsComputeStrategy()
void mimmo::ManipulateWFOBJData::setNormalsComputeStrategy | ( | ManipulateWFOBJData::NormalsComputeMode | mode | ) |
Set the strategy mode to handle vertex normals recomputation on nodes of mesh cells candidates. Available methods are summed up in ManipulateWFOBJData::NormalsComputeMode enum. Class default method is NormalsComputeMode::FLAT_BITPIT method.
- Parameters
-
[in] mode vertex normals computing strategy.
Definition at line 516 of file IOWavefrontOBJ.cpp.
◆ setPinCellGroups()
void mimmo::ManipulateWFOBJData::setPinCellGroups | ( | const std::vector< std::string > & | cellgroupsList | ) |
Specify a list of cellgroup names to extract mesh cells belonging to these groups. List will be available after class execution, through method getPinnedCellGroup().
- Parameters
-
[in] cellgroupsList list of cellgroups passed as their name(string)
Definition at line 543 of file IOWavefrontOBJ.cpp.
◆ setPinMaterials()
void mimmo::ManipulateWFOBJData::setPinMaterials | ( | const std::vector< std::string > & | materialsList | ) |
Specify a list of material names to extract mesh cells owning those material properties. List will be available after class execution, through method getPinnedMaterialGroup().
- Parameters
-
[in] materialsList list of materials passed as their name(string)
Definition at line 526 of file IOWavefrontOBJ.cpp.
◆ setPinObjects()
void mimmo::ManipulateWFOBJData::setPinObjects | ( | const std::vector< std::string > & | objectsList | ) |
Specify a list of object/subparts names to extract mesh cells belonging to them. List will be available after class execution, through method getPinnedObjectGroup().
- Parameters
-
[in] objectsList list of subparts/objects passed as their name(string)
Definition at line 570 of file IOWavefrontOBJ.cpp.
◆ setPinSmoothIds()
void mimmo::ManipulateWFOBJData::setPinSmoothIds | ( | const std::vector< int > & | smoothidsList | ) |
Specify a list of smoothids entries to extract mesh cells belonging to these smooth groups. List will be available after class execution, through method getPinnedSmoothGroup().
- Parameters
-
[in] smoothidsList list of smoothids passed as their integer signature
Definition at line 559 of file IOWavefrontOBJ.cpp.
◆ setRecomputeNormalsCells()
void mimmo::ManipulateWFOBJData::setRecomputeNormalsCells | ( | MimmoPiercedVector< long > * | cellList | ) |
Set the list of candidate mesh cells on which vertex normals (stored in WavefrontOBJData::normals) must be recomputed. The class perform recomputation only if a valid cellList is linked, otherwise it does nothing.
Definition at line 454 of file IOWavefrontOBJ.cpp.
◆ swap()
|
protectednoexcept |
Swap utility
- Parameters
-
[in] x other object to swap from
Definition at line 347 of file IOWavefrontOBJ.cpp.
Member Data Documentation
◆ m_annMode
|
protected |
mode to deal with multiple concurrent annotations on a cell
Definition at line 235 of file IOWavefrontOBJ.hpp.
◆ m_annotations
|
protected |
list of annotations
Definition at line 233 of file IOWavefrontOBJ.hpp.
◆ m_checkNormalsMag
|
protected |
boolean to set check on normals magnitude
Definition at line 234 of file IOWavefrontOBJ.hpp.
◆ m_extData
|
protected |
externally linked data
Definition at line 231 of file IOWavefrontOBJ.hpp.
◆ m_normalsCells
|
protected |
candidate cell list for normals recomputation.
Definition at line 232 of file IOWavefrontOBJ.hpp.
◆ m_normalsMode
|
protected |
mode to deal with normals recompute on a list of candidate cells
Definition at line 236 of file IOWavefrontOBJ.hpp.
◆ m_pinCellGroups
|
protected |
list of cellgroup names for cell list pinning
Definition at line 240 of file IOWavefrontOBJ.hpp.
◆ m_pinMaterials
|
protected |
list of material names for cell list pinning
Definition at line 239 of file IOWavefrontOBJ.hpp.
◆ m_pinnedCellLists
|
protected |
list of cell pinned 0-material, 1-cellgroups, 2-smoothids, 3-objects/subparts
Definition at line 243 of file IOWavefrontOBJ.hpp.
◆ m_pinObjects
|
protected |
list of object/parts names for cell list pinning
Definition at line 238 of file IOWavefrontOBJ.hpp.
◆ m_pinSmoothIds
|
protected |
list of smooth-ids int entries for cell list pinning
Definition at line 241 of file IOWavefrontOBJ.hpp.
The documentation for this class was generated from the following files:
- src/iogeneric/IOWavefrontOBJ.hpp
- src/iogeneric/IOWavefrontOBJ.cpp
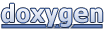