Reconstruct a vector field from daughter mesh to mother mesh. More...
#include <ReconstructFields.hpp>
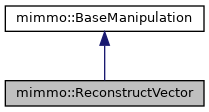
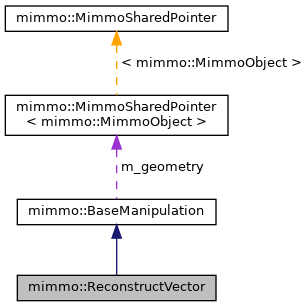
Protected Member Functions | |
virtual void | plotOptionalResults () |
void | swap (ReconstructVector &) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Reconstruct a vector field from daughter mesh to mother mesh.
Class/BaseManipulation Object reconstructing a vector field on a mimmo::MimmoObject mesh, from several vector fields defined on sub-patches of the target mesh, where for sub-patches is meant portion of target mesh, preserving their vertex/cell-ids, as in the case of mimmo::Selection Blocks. Field values can be defined on nodes or cells. No interfaces are supported up to now. Reconstructed field on the whole geometry is provided as result as well as the reconstructed fields on the input sub-patches separately.
Ports available in ReconstructVector Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_VECTORFIELD | addData | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_GEOM | m_geometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_VECTORFIELD | getResultField | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_VECVFIELDS | getResultFields | (MC_VECTOR, MD_MPVECARR3FLOAT_) |
M_GEOM | getGeometry | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation
- ClassName: name of the class as
mimmo.ReconstructVector
; - Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class;
- OutputPlot: target directory for optional results writing;
Proper of the class:
- DataLocation: data location of fields 1-POINT, 2-CELL, 3-INTERFACE;
- OverlapCriterium: set how to treat fields in the overlapped region 1-MaxVal, 2-MinVal, 3-AverageVal, 4-Summing;
Fields and Geometry have to be mandatorily passed through port.
Definition at line 188 of file ReconstructFields.hpp.
Constructor & Destructor Documentation
◆ ReconstructVector() [1/3]
mimmo::ReconstructVector::ReconstructVector | ( | MPVLocation | loc = MPVLocation::POINT | ) |
Constructor
Definition at line 33 of file ReconstructVector.cpp.
◆ ReconstructVector() [2/3]
mimmo::ReconstructVector::ReconstructVector | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 44 of file ReconstructVector.cpp.
◆ ~ReconstructVector()
|
virtual |
Destructor
Definition at line 74 of file ReconstructVector.cpp.
◆ ReconstructVector() [3/3]
mimmo::ReconstructVector::ReconstructVector | ( | const ReconstructVector & | other | ) |
Copy Constructor
Definition at line 79 of file ReconstructVector.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 422 of file ReconstructVector.cpp.
◆ addData()
void mimmo::ReconstructVector::addData | ( | dmpvecarr3E * | field | ) |
Insert in the list data field of a sub-patch.
- Parameters
-
[in] field Sub-patch to be inserted
Definition at line 179 of file ReconstructVector.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 402 of file ReconstructVector.cpp.
◆ clear()
void mimmo::ReconstructVector::clear | ( | ) |
Clear your class data and restore defaults settings
Definition at line 232 of file ReconstructVector.cpp.
◆ execute()
|
virtual |
Execution command. Reconstruct fields and save result in results member.
Implements mimmo::BaseManipulation.
Definition at line 269 of file ReconstructVector.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 463 of file ReconstructVector.cpp.
◆ getNData()
int mimmo::ReconstructVector::getNData | ( | ) |
Return number of fields data actually set in your class
- Returns
- number of fields
Definition at line 125 of file ReconstructVector.cpp.
◆ getOverlapCriterium()
int mimmo::ReconstructVector::getOverlapCriterium | ( | ) |
Get actually used criterium for overlap regions of given fields
- Returns
- criterium for overlap regions
Definition at line 116 of file ReconstructVector.cpp.
◆ getOverlapCriteriumENUM()
OverlapMethod mimmo::ReconstructVector::getOverlapCriteriumENUM | ( | ) |
Get actually used criterium for overlap regions of given fields
- Returns
- criterium for overlap regions
Definition at line 107 of file ReconstructVector.cpp.
◆ getResultField()
dmpvecarr3E * mimmo::ReconstructVector::getResultField | ( | ) |
◆ getResultFields()
std::vector< dmpvecarr3E * > mimmo::ReconstructVector::getResultFields | ( | ) |
Return your result fields
- Returns
- result fields
Definition at line 143 of file ReconstructVector.cpp.
◆ plotData()
void mimmo::ReconstructVector::plotData | ( | ) |
Plot data (resulting field data) on vtu unstructured grid file
Definition at line 242 of file ReconstructVector.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
Plot Optional results in execution, that is the reconstructed vector field .
Reimplemented from mimmo::BaseManipulation.
Definition at line 343 of file ReconstructVector.cpp.
◆ plotSubData()
void mimmo::ReconstructVector::plotSubData | ( | int | i | ) |
Plot sub data (resulting field data) on vtu unstructured grid file
- Parameters
-
[in] i index of the sub-patch
Definition at line 254 of file ReconstructVector.cpp.
◆ removeAllData()
void mimmo::ReconstructVector::removeAllData | ( | ) |
Remove all data present in the list
Definition at line 222 of file ReconstructVector.cpp.
◆ removeData()
void mimmo::ReconstructVector::removeData | ( | mimmo::MimmoSharedPointer< MimmoObject > | patch | ) |
Remove a data field on the list by passing as key its pointer to geometry mesh
- Parameters
-
[in] patch Pointer to sub-patch to be removed.
Definition at line 194 of file ReconstructVector.cpp.
◆ setOverlapCriterium()
void mimmo::ReconstructVector::setOverlapCriterium | ( | int | funct | ) |
Set overlap criterium for multi-fields reconstruction. See OverlapMethod enum Class default is OverlapMethod::MAX. Enum overloading
- Parameters
-
[in] funct Type of overlap method
Definition at line 169 of file ReconstructVector.cpp.
◆ setOverlapCriteriumENUM()
void mimmo::ReconstructVector::setOverlapCriteriumENUM | ( | OverlapMethod | funct | ) |
Set overlap criterium for multi-fields reconstruction. See OverlapMethod enum Class default is OverlapMethod::MAX. Enum overloading
- Parameters
-
[in] funct Type of overlap method
Definition at line 159 of file ReconstructVector.cpp.
◆ swap()
|
protectednoexcept |
Swap function of ReconstructVector
- Parameters
-
[in] x object to be swapped.
Definition at line 91 of file ReconstructVector.cpp.
The documentation for this class was generated from the following files:
- src/geohandlers/ReconstructFields.hpp
- src/geohandlers/ReconstructVector.cpp
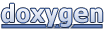