ReconstructVector.cpp
37 if(m_loc == MPVLocation::UNDEFINED || m_loc == MPVLocation::INTERFACE) m_loc= MPVLocation::POINT;
183 (*m_log)<<"warning in "<<m_name<<" : trying to add field referred to a Point Cloud, while Class has Data Location referred to CELLS. Do Nothing."<<std::endl;
321 (*m_log)<<"Warning in "<<m_name<<". It seems a reconstruct field with values uncoherent with target geometry is generated."<<std::endl;
407 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, ReconstructVector>(&m_geometry, M_GEOM, true));
408 built = (built && createPortIn<dmpvecarr3E*, ReconstructVector>(this, &mimmo::ReconstructVector::addData, M_VECTORFIELD, true));
411 built = (built && createPortOut<dmpvecarr3E*, ReconstructVector>(this, &ReconstructVector::getResultField, M_VECTORFIELD));
412 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, ReconstructVector>(&m_geometry, M_GEOM));
413 built = (built && createPortOut<std::vector<dmpvecarr3E*>, ReconstructVector>(this, &mimmo::ReconstructVector::getResultFields, M_VECVECTORFIELDS));
422 void ReconstructVector::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
435 (*m_log)<<"Error absorbing DataLocation in "<<m_name<<". Class and read locations mismatch"<<std::endl;
437 if (temp == 3) (*m_log)<<"XML DataLocation in "<<m_name<<" is set to 3-INTERFACE, not supported for now."<<std::endl;
dmpvecarr3E * getResultField()
Definition: ReconstructVector.cpp:134
OverlapMethod
class for setting overlapping criterium for two different fields
Definition: ReconstructFields.hpp:36
int getOverlapCriterium()
Definition: ReconstructVector.cpp:116
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
virtual void plotOptionalResults()
Definition: ReconstructVector.cpp:343
std::vector< dmpvecarr3E * > getResultFields()
Definition: ReconstructVector.cpp:143
void setOverlapCriteriumENUM(OverlapMethod)
Definition: ReconstructVector.cpp:159
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
@ CELL
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
void swap(ReconstructVector &) noexcept
Definition: ReconstructVector.cpp:91
ReconstructVector(MPVLocation loc=MPVLocation::POINT)
Definition: ReconstructVector.cpp:33
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructVector.cpp:463
@ INTERFACE
@ AVERAGE
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual ~ReconstructVector()
Definition: ReconstructVector.cpp:74
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void removeData(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: ReconstructVector.cpp:194
Reconstruct a vector field from daughter mesh to mother mesh.
Definition: ReconstructFields.hpp:188
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
OverlapMethod getOverlapCriteriumENUM()
Definition: ReconstructVector.cpp:107
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
@ UNDEFINED
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructVector.cpp:422
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void setOverlapCriterium(int)
Definition: ReconstructVector.cpp:169
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
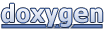