ReconstructFields.hpp
Reconstruct a scalar field from daughter meshes to mother mesh.
Definition: ReconstructFields.hpp:91
dmpvecarr3E * getResultField()
Definition: ReconstructVector.cpp:134
OverlapMethod
class for setting overlapping criterium for two different fields
Definition: ReconstructFields.hpp:36
int getOverlapCriterium()
Definition: ReconstructVector.cpp:116
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
OverlapMethod getOverlapCriteriumENUM()
Definition: ReconstructScalar.cpp:108
virtual void plotOptionalResults()
Definition: ReconstructVector.cpp:343
std::vector< dmpvecarr3E * > getResultFields()
Definition: ReconstructVector.cpp:143
void setOverlapCriteriumENUM(OverlapMethod)
Definition: ReconstructVector.cpp:159
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructScalar.cpp:456
void swap(ReconstructVector &) noexcept
Definition: ReconstructVector.cpp:91
ReconstructVector(MPVLocation loc=MPVLocation::POINT)
Definition: ReconstructVector.cpp:33
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructVector.cpp:463
MimmoPiercedVector is the basic data container for mimmo library.
Definition: MimmoPiercedVector.hpp:60
@ AVERAGE
dmpvector1D * getResultField()
Definition: ReconstructScalar.cpp:134
virtual ~ReconstructScalar()
Definition: ReconstructScalar.cpp:74
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
ReconstructScalar(MPVLocation loc=MPVLocation::POINT)
Definition: ReconstructScalar.cpp:33
virtual ~ReconstructVector()
Definition: ReconstructVector.cpp:74
int getOverlapCriterium()
Definition: ReconstructScalar.cpp:116
std::vector< dmpvector1D * > getResultFields()
Definition: ReconstructScalar.cpp:143
void removeData(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: ReconstructVector.cpp:194
Reconstruct a vector field from daughter mesh to mother mesh.
Definition: ReconstructFields.hpp:188
void removeData(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: ReconstructScalar.cpp:196
OverlapMethod getOverlapCriteriumENUM()
Definition: ReconstructVector.cpp:107
void swap(ReconstructScalar &) noexcept
Definition: ReconstructScalar.cpp:91
@ POINT
virtual void plotOptionalResults()
Definition: ReconstructScalar.cpp:341
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructVector.cpp:422
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructScalar.cpp:415
void setOverlapCriterium(int)
Definition: ReconstructVector.cpp:169
void setOverlapCriteriumENUM(OverlapMethod)
Definition: ReconstructScalar.cpp:159
void setOverlapCriterium(int)
Definition: ReconstructScalar.cpp:169
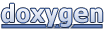