ReconstructScalar.cpp
37 if(m_loc == MPVLocation::UNDEFINED || m_loc == MPVLocation::INTERFACE) m_loc= MPVLocation::POINT;
184 (*m_log)<<"warning in "<<m_name<<" : trying to add field referred to a Point Cloud, while Class has Data Location referred to CELLS. Do Nothing."<<std::endl;
320 (*m_log)<<"Warning in "<<m_name<<". It seems a reconstruct field with values uncoherent with target geometry is generated."<<std::endl;
399 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, ReconstructScalar>(&m_geometry, M_GEOM, true));
400 built = (built && createPortIn<dmpvector1D*, ReconstructScalar>(this, &mimmo::ReconstructScalar::addData, M_SCALARFIELD, true));
403 built = (built && createPortOut<dmpvector1D*, ReconstructScalar>(this, &ReconstructScalar::getResultField, M_SCALARFIELD));
404 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, ReconstructScalar>(&m_geometry, M_GEOM));
405 built = (built && createPortOut<std::vector<dmpvector1D*>, ReconstructScalar>(this, &mimmo::ReconstructScalar::getResultFields, M_VECSCALARFIELDS));
428 (*m_log)<<"Error absorbing DataLocation in "<<m_name<<". Class and read locations mismatch"<<std::endl;
430 if (temp == 3) (*m_log)<<"XML DataLocation in "<<m_name<<" is set to 3-INTERFACE, not supported for now."<<std::endl;
Reconstruct a scalar field from daughter meshes to mother mesh.
Definition: ReconstructFields.hpp:91
OverlapMethod
class for setting overlapping criterium for two different fields
Definition: ReconstructFields.hpp:36
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
OverlapMethod getOverlapCriteriumENUM()
Definition: ReconstructScalar.cpp:108
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
@ CELL
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructScalar.cpp:456
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
@ INTERFACE
@ AVERAGE
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
dmpvector1D * getResultField()
Definition: ReconstructScalar.cpp:134
virtual ~ReconstructScalar()
Definition: ReconstructScalar.cpp:74
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
ReconstructScalar(MPVLocation loc=MPVLocation::POINT)
Definition: ReconstructScalar.cpp:33
int getOverlapCriterium()
Definition: ReconstructScalar.cpp:116
std::vector< dmpvector1D * > getResultFields()
Definition: ReconstructScalar.cpp:143
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void removeData(mimmo::MimmoSharedPointer< MimmoObject >)
Definition: ReconstructScalar.cpp:196
void swap(ReconstructScalar &) noexcept
Definition: ReconstructScalar.cpp:91
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
@ UNDEFINED
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
virtual void plotOptionalResults()
Definition: ReconstructScalar.cpp:341
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ReconstructScalar.cpp:415
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
void setOverlapCriteriumENUM(OverlapMethod)
Definition: ReconstructScalar.cpp:159
void setOverlapCriterium(int)
Definition: ReconstructScalar.cpp:169
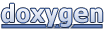