SelectField is an abstract executable block class capable of Selecting a field from a list of fields. More...
#include <SelectField.hpp>
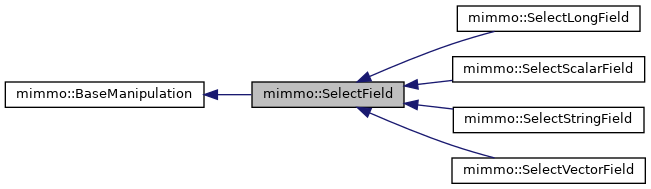
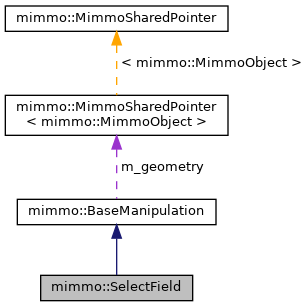
Public Member Functions | |
SelectField () | |
SelectField (const SelectField &other) | |
virtual | ~SelectField () |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | buildPorts () |
void | clear () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
std::string | getFieldName () |
void | setFieldName (std::string fieldname) |
void | setMode (int mode) |
void | setMode (SelectType mode) |
void | setTolerance (double tol) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | swap (SelectField &) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
std::string | m_fieldname |
SelectType | m_mode |
double | m_tol |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
SelectField is an abstract executable block class capable of Selecting a field from a list of fields.
SelectField can work with different selection mode:
- SelectType::GEOMETRY, SelectField takes as input the target geometry used to identify the related field to choose from the input list. It tries to Select the field by investigating the linked geometries in the MimmoPiercedVector input fields and by extracting the first corresponding field.
- SelectType::NAME, SelectField takes as input a field name used to identify the field to choose from the input list. It tries to Select the field by extracting the first field with name equal to the input name.
SelectType::MAPPING, SelectField performs a mapping by testing if the geometry overlap with the geometries linked to the input fields; for each element of the target geometry is chosen the first related element found in the list of the input geometries.
SelectField is an abstract class. To use its features take a look to its specializations, here presented as derived class, SelectScalarField and SelectVectorField.
Ports available in SelectField Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
M_NAME | setName | (MC_SCALAR, MD_STRING) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR, MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.Select<...>Fields
- Priority: uint marking priority in multi-chain execution;
- PlotInExecution: boolean 0/1 print optional results of the class.
- OutputPlot: target directory for optional results writing.
Proper of the class:
- SelectType: selection method. Default SelectType::NAME.
- Tolerance: double > 0, set tolerance of mapping. The option is ignored if mapping is not active.
- FieldName: name of the field to be selected. Default "data".
Fields have to be mandatorily passed through port.
Definition at line 97 of file SelectField.hpp.
Constructor & Destructor Documentation
◆ SelectField() [1/2]
mimmo::SelectField::SelectField | ( | ) |
Default constructor of SelectField.
Definition at line 31 of file SelectField.cpp.
◆ ~SelectField()
|
virtual |
Default destructor of SelectField.
Definition at line 40 of file SelectField.cpp.
◆ SelectField() [2/2]
mimmo::SelectField::SelectField | ( | const SelectField & | other | ) |
Copy constructor of SelectField.
Definition at line 46 of file SelectField.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::SelectStringField, mimmo::SelectLongField, mimmo::SelectVectorField, and mimmo::SelectScalarField.
Definition at line 164 of file SelectField.cpp.
◆ buildPorts()
|
virtual |
Build the ports of the class;
Implements mimmo::BaseManipulation.
Reimplemented in mimmo::SelectStringField, mimmo::SelectLongField, mimmo::SelectVectorField, and mimmo::SelectScalarField.
Definition at line 68 of file SelectField.cpp.
◆ clear()
void mimmo::SelectField::clear | ( | ) |
Clear all stuffs in your class
Definition at line 127 of file SelectField.cpp.
◆ execute()
|
virtual |
Execution command. Select a field over a target geometry from a vector of fields.
Implements mimmo::BaseManipulation.
Definition at line 135 of file SelectField.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Reimplemented in mimmo::SelectStringField, mimmo::SelectLongField, mimmo::SelectVectorField, and mimmo::SelectScalarField.
Definition at line 207 of file SelectField.cpp.
◆ getFieldName()
std::string mimmo::SelectField::getFieldName | ( | ) |
Get the name of your target Selected field.
- Returns
- Target field name
Definition at line 120 of file SelectField.cpp.
◆ setFieldName()
void mimmo::SelectField::setFieldName | ( | std::string | fieldname | ) |
Set the name of your target Selected field. Note. This is the name used as result field after selection; in case of SelectType by geoemtry, the name of the field is replaced by the selected one.
- Parameters
-
[in] fieldname Input field name
Definition at line 84 of file SelectField.cpp.
◆ setMode() [1/2]
void mimmo::SelectField::setMode | ( | int | mode | ) |
Set selection method.
- Parameters
-
[in] mode Selection mode
Definition at line 102 of file SelectField.cpp.
◆ setMode() [2/2]
void mimmo::SelectField::setMode | ( | SelectType | mode | ) |
Set selection method.
- Parameters
-
[in] mode Selection mode
Definition at line 93 of file SelectField.cpp.
◆ setTolerance()
void mimmo::SelectField::setTolerance | ( | double | tol | ) |
Set tolerance for extraction by patch.
- Parameters
-
[in] tol Tolerance
Definition at line 111 of file SelectField.cpp.
◆ swap()
|
protectednoexcept |
Member Data Documentation
◆ m_fieldname
|
protected |
Name of the field to be selected. If SelectType is by geometry, after the execution m_fieldname is the name of the selcted field. If SelectType is by mapping, the user can set the result field name by setFieldName method (or FieldName option in xml input).
Definition at line 101 of file SelectField.hpp.
◆ m_mode
|
protected |
Selection method to be used.
Definition at line 99 of file SelectField.hpp.
◆ m_tol
|
protected |
Tolerance for extraction by patch.
Definition at line 100 of file SelectField.hpp.
The documentation for this class was generated from the following files:
- src/geohandlers/SelectField.hpp
- src/geohandlers/SelectField.cpp
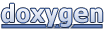