SelectField.hpp
void plotOptionalResults()
Definition: SelectLongField.cpp:167
void addField(MimmoPiercedVector< std::string > *field)
Definition: SelectStringField.cpp:145
void setFields(std::vector< MimmoPiercedVector< long > * > fields)
Definition: SelectLongField.cpp:128
void addField(dmpvector1D *field)
Definition: SelectScalarField.cpp:145
dmpvector1D * getSelectedField()
Definition: SelectScalarField.cpp:119
void swap(SelectVectorField &) noexcept
Definition: SelectVectorField.cpp:90
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
MimmoPiercedVector< std::string > * getSelectedField()
Definition: SelectStringField.cpp:119
SelectLongField is specialized derived class of SelectField to Select a scalar field of long data.
Definition: SelectField.hpp:373
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectLongField.cpp:298
void setFieldName(std::string fieldname)
Definition: SelectField.cpp:84
void addField(dmpvecarr3E *field)
Definition: SelectVectorField.cpp:144
virtual ~SelectScalarField()
Definition: SelectScalarField.cpp:76
@ NAME
void swap(SelectScalarField &) noexcept
Definition: SelectScalarField.cpp:91
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
SelectVectorField is specialized derived class of SelectField to Select a vector field.
Definition: SelectField.hpp:282
SelectScalarField is specialized derived class of SelectField to Select a scalar field.
Definition: SelectField.hpp:192
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectScalarField.cpp:280
MimmoPiercedVector is the basic data container for mimmo library.
Definition: MimmoPiercedVector.hpp:60
SelectStringField(MPVLocation loc=MPVLocation::POINT)
Definition: SelectStringField.cpp:36
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectField.cpp:164
@ MAPPING
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectVectorField.cpp:278
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectVectorField.cpp:306
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectScalarField.cpp:308
MimmoPiercedVector< long > * getSelectedField()
Definition: SelectLongField.cpp:119
@ GEOMETRY
virtual ~SelectLongField()
Definition: SelectLongField.cpp:76
virtual ~SelectVectorField()
Definition: SelectVectorField.cpp:75
SelectVectorField(MPVLocation loc=MPVLocation::POINT)
Definition: SelectVectorField.cpp:36
void addField(MimmoPiercedVector< long > *field)
Definition: SelectLongField.cpp:145
@ POINT
SelectStringField is specialized derived class of SelectField to Select a scalar field of string data...
Definition: SelectField.hpp:464
void setFields(std::vector< MimmoPiercedVector< std::string > * > fields)
Definition: SelectStringField.cpp:128
virtual ~SelectStringField()
Definition: SelectStringField.cpp:76
SelectScalarField(MPVLocation loc=MPVLocation::POINT)
Definition: SelectScalarField.cpp:36
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectLongField.cpp:270
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectStringField.cpp:297
void plotOptionalResults()
Definition: SelectStringField.cpp:167
void swap(SelectStringField &) noexcept
Definition: SelectStringField.cpp:91
SelectField is an abstract executable block class capable of Selecting a field from a list of fields.
Definition: SelectField.hpp:97
void plotOptionalResults()
Definition: SelectVectorField.cpp:165
SelectLongField(MPVLocation loc=MPVLocation::POINT)
Definition: SelectLongField.cpp:36
void plotOptionalResults()
Definition: SelectScalarField.cpp:167
void setFields(std::vector< dmpvecarr3E * > fields)
Definition: SelectVectorField.cpp:127
dmpvecarr3E * getSelectedField()
Definition: SelectVectorField.cpp:118
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectField.cpp:207
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectStringField.cpp:269
void setFields(std::vector< dmpvector1D * > fields)
Definition: SelectScalarField.cpp:128
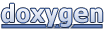