SelectVectorField.cpp
105 built = (built && createPortIn<std::vector<dmpvecarr3E*>, SelectVectorField>(this, &mimmo::SelectVectorField::setFields, M_VECVECTORFIELDS, true, 1));
106 built = (built && createPortIn<dmpvecarr3E*, SelectVectorField>(this, &mimmo::SelectVectorField::addField, M_VECTORFIELD, true, 1));
107 built = (built && createPortOut<dmpvecarr3E*, SelectVectorField>(this, &mimmo::SelectVectorField::getSelectedField, M_VECTORFIELD));
278 void SelectVectorField::absorbSectionXML(const bitpit::Config::Section & slotXML, std::string name){
291 (*m_log)<<"Error absorbing DataLocation in "<<m_name<<". Class and read locations mismatch"<<std::endl;
void swap(SelectVectorField &) noexcept
Definition: SelectVectorField.cpp:90
MPVLocation
Define data location for the MimmoPiercedVector field.
Definition: MimmoPiercedVector.hpp:39
void setName(std::string name)
Definition: MimmoPiercedVector.tpp:364
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
void addField(dmpvecarr3E *field)
Definition: SelectVectorField.cpp:144
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
@ NAME
SelectVectorField is specialized derived class of SelectField to Select a vector field.
Definition: SelectField.hpp:282
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectField.cpp:164
@ MAPPING
void write(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:979
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectVectorField.cpp:278
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectVectorField.cpp:306
@ GEOMETRY
virtual ~SelectVectorField()
Definition: SelectVectorField.cpp:75
SelectVectorField(MPVLocation loc=MPVLocation::POINT)
Definition: SelectVectorField.cpp:36
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
@ UNDEFINED
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
SelectField is an abstract executable block class capable of Selecting a field from a list of fields.
Definition: SelectField.hpp:97
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void plotOptionalResults()
Definition: SelectVectorField.cpp:165
@ MAPPING
void setFields(std::vector< dmpvecarr3E * > fields)
Definition: SelectVectorField.cpp:127
dmpvecarr3E * getSelectedField()
Definition: SelectVectorField.cpp:118
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectField.cpp:207
mimmo::MimmoPiercedVector< darray3E > dmpvecarr3E
Definition: MimmoPiercedVector.hpp:129
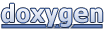