SelectField.cpp
70 built = (built && createPortIn<mimmo::MimmoSharedPointer<MimmoObject>, SelectField>(this, &mimmo::SelectField::setGeometry, M_GEOM));
71 built = (built && createPortIn<std::string, SelectField>(this, &mimmo::SelectField::setFieldName, M_NAME));
72 built = (built && createPortOut<std::string, SelectField>(this, &mimmo::SelectField::getFieldName, M_NAME));
73 built = (built && createPortOut<mimmo::MimmoSharedPointer<MimmoObject>, SelectField>(this, &mimmo::BaseManipulation::getGeometry, M_GEOM));
143 (*m_log)<<" missing or unfound ref Geometry to select field ->see method setGeometry//M_GEOM port"<<std::endl;
146 (*m_log)<<" missing mapping Geometry to select field ->see method setGeometry//M_GEOM port"<<std::endl;
void setFieldName(std::string fieldname)
Definition: SelectField.cpp:84
@ NAME
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectField.cpp:164
@ MAPPING
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
@ GEOMETRY
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
SelectField is an abstract executable block class capable of Selecting a field from a list of fields.
Definition: SelectField.hpp:97
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: SelectField.cpp:207
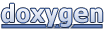