ScaleGeometry is the class that applies a scaling to a given geometry patch in respect to the mean point of the vertices. More...
#include <ScaleGeometry.hpp>
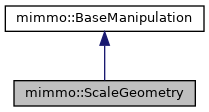
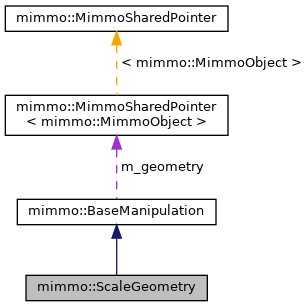
Public Member Functions | |
ScaleGeometry (const bitpit::Config::Section &rootXML) | |
ScaleGeometry (const ScaleGeometry &other) | |
ScaleGeometry (darray3E scaling={ {1.0, 1.0, 1.0} }) | |
~ScaleGeometry () | |
virtual void | absorbSectionXML (const bitpit::Config::Section &slotXML, std::string name="") |
void | apply () |
void | buildPorts () |
void | execute () |
virtual void | flushSectionXML (bitpit::Config::Section &slotXML, std::string name="") |
dmpvecarr3E * | getDisplacements () |
ScaleGeometry & | operator= (ScaleGeometry other) |
void | setFilter (dmpvector1D *filter) |
void | setMeanPoint (bool meanP) |
void | setOrigin (darray3E origin) |
void | setScaling (darray3E scaling) |
![]() | |
BaseManipulation () | |
BaseManipulation (const BaseManipulation &other) | |
virtual | ~BaseManipulation () |
void | activate () |
bool | arePortsBuilt () |
void | clear () |
void | disable () |
void | exec () |
BaseManipulation * | getChild (int i=0) |
ConnectionType | getConnectionType () |
MimmoSharedPointer< MimmoObject > | getGeometry () |
MimmoSharedPointer< MimmoObject > & | getGeometryReference () |
int | getId () |
bitpit::Logger & | getLog () |
std::string | getName () |
int | getNChild () |
int | getNParent () |
int | getNPortsIn () |
int | getNPortsOut () |
BaseManipulation * | getParent (int i=0) |
std::unordered_map< PortID, PortIn * > | getPortsIn () |
std::unordered_map< PortID, PortOut * > | getPortsOut () |
uint | getPriority () |
virtual std::vector< BaseManipulation * > | getSubBlocksEmbedded () |
bool | isActive () |
bool | isApply () |
bool | isChild (BaseManipulation *, int &) |
bool | isParent (BaseManipulation *, int &) |
bool | isPlotInExecution () |
BaseManipulation & | operator= (const BaseManipulation &other) |
void | removePins () |
void | removePinsIn () |
void | removePinsOut () |
void | setApply (bool flag=true) |
void | setGeometry (MimmoSharedPointer< MimmoObject > geometry) |
void | setId (int) |
void | setLog (bitpit::Logger &log) |
void | setName (std::string name) |
void | setOutputPlot (std::string path) |
void | setPlotInExecution (bool) |
void | setPriority (uint priority) |
void | unsetGeometry () |
Protected Member Functions | |
void | checkFilter () |
void | swap (ScaleGeometry &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
virtual void | plotOptionalResults () |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
ScaleGeometry is the class that applies a scaling to a given geometry patch in respect to the mean point of the vertices.
The used parameters are the scaling factor values for each direction of the cartesian reference system.
Ports available in ScaleGeometry Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_POINT | setOrigin | (MC_ARRAY3, MD_FLOAT) |
M_SPAN | setScaling | (MC_ARRAY3, MD_FLOAT) |
M_FILTER | setFilter | (MC_SCALAR, MD_MPVECFLOAT_) |
M_GEOM | setGeometry | (MC_SCALAR, MD_MIMMO_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GDISPLS | getDisplacements | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_GEOM | getGeometry | (MC_SCALAR,MD_MIMMO_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName: name of the class as
mimmo.ScaleGeometry
; - Priority: uint marking priority in multi-chain execution;
- Apply: boolean 0/1 activate apply deformation result on target geometry directly in execution;
Proper of the class:
- Origin: scaling center point (coords space separated);
- MeanPoint: 0/1 don't use/use mean point as center of scaling.If 1 it masks Origin input;
- Scaling: scaling values for each cartesian axis (space separated).
Geometry has to be mandatorily passed through port.
- Examples
- manipulators_example_00001.cpp.
Definition at line 78 of file ScaleGeometry.hpp.
Constructor & Destructor Documentation
◆ ScaleGeometry() [1/3]
mimmo::ScaleGeometry::ScaleGeometry | ( | darray3E | scaling = { {1.0, 1.0, 1.0} } | ) |
Default constructor of ScaleGeometry
Definition at line 32 of file ScaleGeometry.cpp.
◆ ScaleGeometry() [2/3]
mimmo::ScaleGeometry::ScaleGeometry | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 43 of file ScaleGeometry.cpp.
◆ ~ScaleGeometry()
mimmo::ScaleGeometry::~ScaleGeometry | ( | ) |
Default destructor of ScaleGeometry
Definition at line 62 of file ScaleGeometry.cpp.
◆ ScaleGeometry() [3/3]
mimmo::ScaleGeometry::ScaleGeometry | ( | const ScaleGeometry & | other | ) |
Copy constructor of ScaleGeometry. No result geometry displacements are copied.
Definition at line 66 of file ScaleGeometry.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 231 of file ScaleGeometry.cpp.
◆ apply()
|
virtual |
Directly apply deformation field to target geometry.
Reimplemented from mimmo::BaseManipulation.
Definition at line 196 of file ScaleGeometry.cpp.
◆ buildPorts()
|
virtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 99 of file ScaleGeometry.cpp.
◆ checkFilter()
|
protected |
Check if the filter is related to the target geometry. If not create a unitary filter field.
Definition at line 205 of file ScaleGeometry.cpp.
◆ execute()
|
virtual |
Execution command. It perform the scaling by computing the displacements of the points of the geometry. It applies a filter field eventually set as input.
Implements mimmo::BaseManipulation.
Definition at line 157 of file ScaleGeometry.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 278 of file ScaleGeometry.cpp.
◆ getDisplacements()
dmpvecarr3E * mimmo::ScaleGeometry::getDisplacements | ( | ) |
Return actual computed displacements field (if any) for the geometry linked.
- Returns
- deformation field
Definition at line 149 of file ScaleGeometry.cpp.
◆ operator=()
ScaleGeometry & mimmo::ScaleGeometry::operator= | ( | ScaleGeometry | other | ) |
Assignment operator. No result geometry displacements are copied.
Definition at line 76 of file ScaleGeometry.cpp.
◆ setFilter()
void mimmo::ScaleGeometry::setFilter | ( | dmpvector1D * | filter | ) |
It sets the filter field to modulate the displacements of the vertices of the target geometry.
- Parameters
-
[in] filter filter field defined on geometry vertices.
Definition at line 139 of file ScaleGeometry.cpp.
◆ setMeanPoint()
void mimmo::ScaleGeometry::setMeanPoint | ( | bool | meanP | ) |
It sets if the center point for scaling is the mean point of the geometry.
- Parameters
-
[in] meanP origin for scaling transform is the mean point?
Definition at line 122 of file ScaleGeometry.cpp.
◆ setOrigin()
void mimmo::ScaleGeometry::setOrigin | ( | darray3E | origin | ) |
It sets the center point.
- Parameters
-
[in] origin origin for scaling transform
Definition at line 114 of file ScaleGeometry.cpp.
◆ setScaling()
void mimmo::ScaleGeometry::setScaling | ( | darray3E | scaling | ) |
It sets the scaling factors of each axis.
- Parameters
-
[in] scaling scaling factor values for x, y and z absolute axis.
- Examples
- manipulators_example_00001.cpp.
Definition at line 130 of file ScaleGeometry.cpp.
◆ swap()
|
protectednoexcept |
The documentation for this class was generated from the following files:
- src/manipulators/ScaleGeometry.hpp
- src/manipulators/ScaleGeometry.cpp
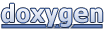