ScaleGeometry.cpp
103 built = (built && createPortIn<dmpvector1D*, ScaleGeometry>(this, &mimmo::ScaleGeometry::setFilter, M_FILTER));
104 built = (built && createPortIn<MimmoSharedPointer<MimmoObject>, ScaleGeometry>(&m_geometry, M_GEOM, true));
105 built = (built && createPortOut<dmpvecarr3E*, ScaleGeometry>(this, &mimmo::ScaleGeometry::getDisplacements, M_GDISPLS));
106 built = (built && createPortOut<MimmoSharedPointer<MimmoObject>, ScaleGeometry>(this, &BaseManipulation::getGeometry, M_GEOM));
212 (*m_log)<<"Not valid filter found in "<<m_name<<". Proceeding with default unitary field"<<std::endl;
dmpvecarr3E * getDisplacements()
Definition: ScaleGeometry.cpp:149
ScaleGeometry is the class that applies a scaling to a given geometry patch in respect to the mean po...
Definition: ScaleGeometry.hpp:78
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: ScaleGeometry.cpp:278
MPVLocation getDataLocation()
Definition: MimmoPiercedVector.tpp:190
bool completeMissingData(const mpv_t &defValue)
Definition: MimmoPiercedVector.tpp:567
void warningXML(bitpit::Logger *log, std::string name)
Definition: MimmoNamespace.cpp:211
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: ScaleGeometry.cpp:231
ScaleGeometry & operator=(ScaleGeometry other)
Definition: ScaleGeometry.cpp:76
MimmoSharedPointer< MimmoObject > getGeometry() const
Definition: MimmoPiercedVector.tpp:170
virtual void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:615
virtual void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: BaseManipulation.cpp:670
MimmoSharedPointer< MimmoObject > m_geometry
Definition: BaseManipulation.hpp:144
void setGeometry(MimmoSharedPointer< MimmoObject > geo)
Definition: MimmoPiercedVector.tpp:314
@ POINT
void _apply(MimmoPiercedVector< darray3E > &displacements)
Definition: BaseManipulation.cpp:956
void setDataLocation(MPVLocation loc)
Definition: MimmoPiercedVector.tpp:324
ScaleGeometry(darray3E scaling={ {1.0, 1.0, 1.0} })
Definition: ScaleGeometry.cpp:32
MimmoSharedPointer< MimmoObject > getGeometry()
Definition: BaseManipulation.cpp:235
void swap(BaseManipulation &x) noexcept
Definition: BaseManipulation.cpp:140
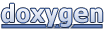