Create a 3DCurve from a point cloud. More...
#include <Create3DCurve.hpp>
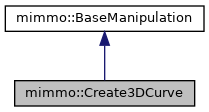

Protected Member Functions | |
void | buildPorts () |
void | plotOptionalResults () |
void | swap (Create3DCurve &x) noexcept |
![]() | |
void | _apply (MimmoPiercedVector< darray3E > &displacements) |
void | addChild (BaseManipulation *child) |
void | addParent (BaseManipulation *parent) |
void | addPinIn (BaseManipulation *objIn, PortID portR) |
void | addPinOut (BaseManipulation *objOut, PortID portS, PortID portR) |
virtual void | apply () |
void | cleanBufferIn (PortID port) |
template<typename T , typename O > | |
bool | createPortIn (O *obj, void(O::*setVar_)(T), PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortIn (T *var_, PortID portR, bool mandatory=false, int family=0) |
template<typename T , typename O > | |
bool | createPortOut (O *obj, T(O::*getVar_)(), PortID portS) |
template<typename T , typename O > | |
bool | createPortOut (T *var_, PortID portS) |
void | deletePorts () |
PortID | findPinIn (PortIn &pin) |
PortID | findPinOut (PortOut &pin) |
void | initializeLogger (bool logexists) |
void | readBufferIn (PortID port) |
void | removePinIn (BaseManipulation *objIn, PortID portR) |
void | removePinIn (PortID portR, int j) |
void | removePinOut (BaseManipulation *objOut, PortID portS) |
void | removePinOut (PortID portS, int j) |
void | setBufferIn (PortID port, mimmo::IBinaryStream &input) |
void | swap (BaseManipulation &x) noexcept |
void | unsetChild (BaseManipulation *child) |
void | unsetParent (BaseManipulation *parent) |
void | write (MimmoSharedPointer< MimmoObject > geometry) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, MimmoPiercedVector< mpv_t > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t > * > &data, Args ... args) |
template<typename mpv_t > | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data) |
template<typename mpv_t , typename... Args> | |
void | write (MimmoSharedPointer< MimmoObject > geometry, std::vector< MimmoPiercedVector< mpv_t >> &data, Args ... args) |
Protected Attributes | |
bool | m_closed |
int | m_nCells |
dmpvecarr3E | m_rawpoints |
dmpvector1D | m_rawscalar |
dmpvecarr3E | m_rawvector |
dmpvector1D | m_scalarfield |
dmpvecarr3E | m_vectorfield |
![]() | |
bool | m_active |
bool | m_apply |
bool | m_arePortsBuilt |
bmumap | m_child |
int | m_counter |
bool | m_execPlot |
MimmoSharedPointer< MimmoObject > | m_geometry |
bitpit::Logger * | m_log |
std::string | m_name |
std::string | m_outputPlot |
bmumap | m_parent |
std::unordered_map< PortID, PortIn * > | m_portIn |
std::unordered_map< PortID, PortOut * > | m_portOut |
ConnectionType | m_portsType |
uint | m_priority |
Additional Inherited Members | |
![]() | |
typedef std::unordered_map< BaseManipulation *, int > | bmumap |
typedef pin::ConnectionType | ConnectionType |
typedef std::string | PortID |
![]() | |
static int | sm_baseManipulationCounter |
Detailed Description
Create a 3DCurve from a point cloud.
The class takes point data from a raw list and reverse them in a MimmoObject 3D Curve container, using the consecutive ordering of the list to create connectivity of the curve. List can be set as pure vector or as a MimmoPiercedVector. Please be aware one set method exclude the other. Optionally, If any scalar/vector field are assigned to raw points, they will be trasformed in data containers associated to curve, with location on POINT. Please note if the raw points are passed with a specific container list (vector or MimmoPiercedVector), only data passed with the same kind of container will be taken into account.
MPI version retains data only on the master rank (rank 0). For now, MPI Setting of raw data is supposed to happen in this two configuration: 1) only master rank (0) retains the data 2) every rank has the same identical data set. So, each time the class will consider only data from master rank. No 3DCurve distribution among ranks is perfomed. Mesh and Field Data will be retained only on the master rank(0).
Ports available in Create3DCurve Class :
Port Input | ||
---|---|---|
PortType | variable/function | DataType |
M_COORDS | setRawPoints | (MC_VECARR3, MD_FLOAT) |
M_DISPLS | setRawVectorField | (MC_VECARR3, MD_FLOAT) |
M_DATAFIELD | setRawScalarField | (MC_VECTOR, MD_FLOAT) |
M_VECTORFIELD | setRawPoints | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_VECTORFIELD2 | setRawVectorField | (MC_SCALAR, MD_MPVECARR3FLOAT_) |
M_SCALARFIELD | setRawScalarField | (MC_SCALAR,MD_MPVECFLOAT_) |
Port Output | ||
---|---|---|
PortType | variable/function | DataType |
M_GEOM | getGeometry | (MC_SCALAR,MD_MIMMO_) |
M_SCALARFIELD | getScalarField | (MC_SCALAR,MD_MPVECFLOAT_) |
M_VECTORFIELD | getVectorField | (MC_SCALAR,MD_MPVECARR3FLOAT_) |
The xml available parameters, sections and subsections are the following :
Inherited from BaseManipulation:
- ClassName : name of the class as
mimmo.Create3DCurve
; - Priority : uint marking priority in multi-chain execution;
- PlotInExecution : boolean 0/1 print optional results of the class;
- OutputPlot : target directory for optional results writing.
Proper of the class:
- ClosedLoop : 0/1 to connect the point cloud list as open/closed 3D curve;
- nCells : refine curve tessellation up to a target number of cells (>= number of cloud points);
Geometry has to be mandatorily passed through port.
Definition at line 105 of file Create3DCurve.hpp.
Constructor & Destructor Documentation
◆ Create3DCurve() [1/3]
mimmo::Create3DCurve::Create3DCurve | ( | ) |
Default constructor
Definition at line 43 of file Create3DCurve.cpp.
◆ Create3DCurve() [2/3]
mimmo::Create3DCurve::Create3DCurve | ( | const bitpit::Config::Section & | rootXML | ) |
Custom constructor reading xml data
- Parameters
-
[in] rootXML reference to your xml tree section
Definition at line 53 of file Create3DCurve.cpp.
◆ ~Create3DCurve()
|
virtual |
Default destructor
Definition at line 72 of file Create3DCurve.cpp.
◆ Create3DCurve() [3/3]
mimmo::Create3DCurve::Create3DCurve | ( | const Create3DCurve & | other | ) |
Copy constructor. /param[in] other to be copied
Definition at line 78 of file Create3DCurve.cpp.
Member Function Documentation
◆ absorbSectionXML()
|
virtual |
It sets infos reading from a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 276 of file Create3DCurve.cpp.
◆ buildPorts()
|
protectedvirtual |
It builds the input/output ports of the object
Implements mimmo::BaseManipulation.
Definition at line 116 of file Create3DCurve.cpp.
◆ clear()
void mimmo::Create3DCurve::clear | ( | ) |
Clear all data stored in the class
Definition at line 229 of file Create3DCurve.cpp.
◆ execute()
|
virtual |
Execute command.
Implements mimmo::BaseManipulation.
Definition at line 336 of file Create3DCurve.cpp.
◆ flushSectionXML()
|
virtual |
It sets infos from class members in a XML bitpit::Config::section.
- Parameters
-
[in] slotXML bitpit::Config::Section of XML file [in] name name associated to the slot
Reimplemented from mimmo::BaseManipulation.
Definition at line 311 of file Create3DCurve.cpp.
◆ getScalarField()
dmpvector1D * mimmo::Create3DCurve::getScalarField | ( | ) |
Return the scalar field stored in the class as pointer to MimmoPiercedVector object.
- Returns
- scalar field stored in the class
Definition at line 139 of file Create3DCurve.cpp.
◆ getVectorField()
dmpvecarr3E * mimmo::Create3DCurve::getVectorField | ( | ) |
Return the vector field stored in the class as pointer to MimmoPiercedVector object.
- Returns
- vector field stored in the class
Definition at line 148 of file Create3DCurve.cpp.
◆ isClosedLoop()
bool mimmo::Create3DCurve::isClosedLoop | ( | ) |
- Returns
- true if the class is set to create a final closed 3D Curve
Definition at line 245 of file Create3DCurve.cpp.
◆ operator=()
Create3DCurve & mimmo::Create3DCurve::operator= | ( | Create3DCurve | other | ) |
Assignement operator. /param[in] other to be copied
Definition at line 90 of file Create3DCurve.cpp.
◆ plotOptionalResults()
|
protectedvirtual |
Plot 3DCurve and store it in *.vtu file
Reimplemented from mimmo::BaseManipulation.
Definition at line 325 of file Create3DCurve.cpp.
◆ setClosedLoop()
void mimmo::Create3DCurve::setClosedLoop | ( | bool | flag | ) |
Set your final 3D curve as open/closed.
- Parameters
-
[in] flag false to get an open curve, true for a closed one.
Definition at line 254 of file Create3DCurve.cpp.
◆ setNCells()
void mimmo::Create3DCurve::setNCells | ( | int | ncells | ) |
Set target cells of the 3D curve final tessellation, in order to refine/regularize it. This number must be >= of the number of raw point list. If it is, the class will split in half the longest segments of tessellation, until the target number of cells is achieved.
- Parameters
-
[in] ncells number of target cells.
Definition at line 266 of file Create3DCurve.cpp.
◆ setRawPoints() [1/2]
void mimmo::Create3DCurve::setRawPoints | ( | dmpvecarr3E * | rawPoints | ) |
It sets the point cloud raw list
- Parameters
-
[in] rawPoints raw list of points.
Definition at line 158 of file Create3DCurve.cpp.
◆ setRawPoints() [2/2]
void mimmo::Create3DCurve::setRawPoints | ( | dvecarr3E | rawPoints | ) |
It sets the point cloud raw list
- Parameters
-
[in] rawPoints raw list of points.
Definition at line 167 of file Create3DCurve.cpp.
◆ setRawScalarField() [1/2]
void mimmo::Create3DCurve::setRawScalarField | ( | dmpvector1D * | rawScalarField | ) |
It sets the scalar field optionally attached to point cloud raw list
- Parameters
-
[in] rawScalarField raw scalar field.
Definition at line 206 of file Create3DCurve.cpp.
◆ setRawScalarField() [2/2]
void mimmo::Create3DCurve::setRawScalarField | ( | dvector1D | rawScalarField | ) |
It sets the scalar field optionally attached to point cloud raw list
- Parameters
-
[in] rawScalarField raw scalar field.
Definition at line 215 of file Create3DCurve.cpp.
◆ setRawVectorField() [1/2]
void mimmo::Create3DCurve::setRawVectorField | ( | dmpvecarr3E * | rawVectorField | ) |
It sets the vector field optionally attached to point cloud raw list
- Parameters
-
[in] rawVectorField raw vector field.
Definition at line 182 of file Create3DCurve.cpp.
◆ setRawVectorField() [2/2]
void mimmo::Create3DCurve::setRawVectorField | ( | dvecarr3E | rawVectorField | ) |
It sets the vector field optionally attached to point cloud raw list
- Parameters
-
[in] rawVectorField raw vector field.
Definition at line 191 of file Create3DCurve.cpp.
◆ swap()
|
protectednoexcept |
Member Data Documentation
◆ m_closed
|
protected |
flag to create closed/open loops
Definition at line 116 of file Create3DCurve.hpp.
◆ m_nCells
|
protected |
flag to set a target number of cells in the curve
Definition at line 117 of file Create3DCurve.hpp.
◆ m_rawpoints
|
protected |
input cloud points list, in raw format.
Definition at line 112 of file Create3DCurve.hpp.
◆ m_rawscalar
|
protected |
input scalar attached to cloud points, in raw format.
Definition at line 113 of file Create3DCurve.hpp.
◆ m_rawvector
|
protected |
input vector attached to cloud points, in raw format
Definition at line 114 of file Create3DCurve.hpp.
◆ m_scalarfield
|
protected |
MimmoPiercedVector scalar field
Definition at line 109 of file Create3DCurve.hpp.
◆ m_vectorfield
|
protected |
MimmoPiercedVector vector field
Definition at line 110 of file Create3DCurve.hpp.
The documentation for this class was generated from the following files:
- src/iogeneric/Create3DCurve.hpp
- src/iogeneric/Create3DCurve.cpp
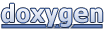