Create3DCurve.hpp
157 int fillPreliminaryStructure(dmpvecarr3E & points, std::unordered_map<long, std::array<long,2> > &connectivity);
158 void refineObject(dmpvecarr3E & points, dmpvector1D & scalarf, dmpvecarr3E & vectorf, std::unordered_map<long, std::array<long,2> > &connectivity, int fCells);
160 void setGeometry(MimmoSharedPointer<MimmoObject> geometry){BaseManipulation::setGeometry(geometry);};
BaseManipulation is the base class of any manipulation object of the library.
Definition: BaseManipulation.hpp:102
void setRawVectorField(dvecarr3E rawVectorField)
Definition: Create3DCurve.cpp:191
#define REGISTER_PORT(Name, Container, Datatype, ManipBlock)
Definition: portManager.hpp:211
void plotOptionalResults()
Definition: Create3DCurve.cpp:325
void setRawPoints(dvecarr3E rawPoints)
Definition: Create3DCurve.cpp:167
void setRawScalarField(dvector1D rawScalarField)
Definition: Create3DCurve.cpp:215
void flushSectionXML(bitpit::Config::Section &slotXML, std::string name="")
Definition: Create3DCurve.cpp:311
void setGeometry(MimmoSharedPointer< MimmoObject > geometry)
Definition: BaseManipulation.cpp:433
Create3DCurve & operator=(Create3DCurve other)
Definition: Create3DCurve.cpp:90
void absorbSectionXML(const bitpit::Config::Section &slotXML, std::string name="")
Definition: Create3DCurve.cpp:276
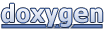